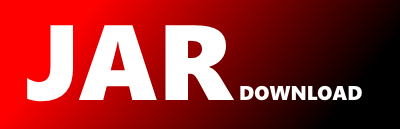
com.pulumi.gitlab.kotlin.ProjectLevelMrApprovalsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.ProjectLevelMrApprovalsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab_project_level_mr_approval_rule` resource allows to manage the lifecycle of a Merge Request-level approval rule.
* > This resource requires a GitLab Enterprise instance.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/merge_request_approvals.html#merge-request-level-mr-approvals)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const foo = new gitlab.Project("foo", {
* name: "Example",
* description: "My example project",
* });
* const fooProjectLevelMrApprovals = new gitlab.ProjectLevelMrApprovals("foo", {
* project: foo.id,
* resetApprovalsOnPush: true,
* disableOverridingApproversPerMergeRequest: false,
* mergeRequestsAuthorApproval: false,
* mergeRequestsDisableCommittersApproval: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* foo = gitlab.Project("foo",
* name="Example",
* description="My example project")
* foo_project_level_mr_approvals = gitlab.ProjectLevelMrApprovals("foo",
* project=foo.id,
* reset_approvals_on_push=True,
* disable_overriding_approvers_per_merge_request=False,
* merge_requests_author_approval=False,
* merge_requests_disable_committers_approval=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var foo = new GitLab.Project("foo", new()
* {
* Name = "Example",
* Description = "My example project",
* });
* var fooProjectLevelMrApprovals = new GitLab.ProjectLevelMrApprovals("foo", new()
* {
* Project = foo.Id,
* ResetApprovalsOnPush = true,
* DisableOverridingApproversPerMergeRequest = false,
* MergeRequestsAuthorApproval = false,
* MergeRequestsDisableCommittersApproval = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* foo, err := gitlab.NewProject(ctx, "foo", &gitlab.ProjectArgs{
* Name: pulumi.String("Example"),
* Description: pulumi.String("My example project"),
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewProjectLevelMrApprovals(ctx, "foo", &gitlab.ProjectLevelMrApprovalsArgs{
* Project: foo.ID(),
* ResetApprovalsOnPush: pulumi.Bool(true),
* DisableOverridingApproversPerMergeRequest: pulumi.Bool(false),
* MergeRequestsAuthorApproval: pulumi.Bool(false),
* MergeRequestsDisableCommittersApproval: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.Project;
* import com.pulumi.gitlab.ProjectArgs;
* import com.pulumi.gitlab.ProjectLevelMrApprovals;
* import com.pulumi.gitlab.ProjectLevelMrApprovalsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Project("foo", ProjectArgs.builder()
* .name("Example")
* .description("My example project")
* .build());
* var fooProjectLevelMrApprovals = new ProjectLevelMrApprovals("fooProjectLevelMrApprovals", ProjectLevelMrApprovalsArgs.builder()
* .project(foo.id())
* .resetApprovalsOnPush(true)
* .disableOverridingApproversPerMergeRequest(false)
* .mergeRequestsAuthorApproval(false)
* .mergeRequestsDisableCommittersApproval(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: gitlab:Project
* properties:
* name: Example
* description: My example project
* fooProjectLevelMrApprovals:
* type: gitlab:ProjectLevelMrApprovals
* name: foo
* properties:
* project: ${foo.id}
* resetApprovalsOnPush: true
* disableOverridingApproversPerMergeRequest: false
* mergeRequestsAuthorApproval: false
* mergeRequestsDisableCommittersApproval: true
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_project_level_mr_approvals`. For example:
* terraform
* import {
* to = gitlab_project_level_mr_approvals.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* ```sh
* $ pulumi import gitlab:index/projectLevelMrApprovals:ProjectLevelMrApprovals You can import an approval configuration state using ` `.
* ```
* For example:
* ```sh
* $ pulumi import gitlab:index/projectLevelMrApprovals:ProjectLevelMrApprovals foo 1234
* ```
* @property disableOverridingApproversPerMergeRequest Set to `true` to disable overriding approvers per merge request.
* @property mergeRequestsAuthorApproval Set to `true` to allow merge requests authors to approve their own merge requests.
* @property mergeRequestsDisableCommittersApproval Set to `true` to disable merge request committers from approving their own merge requests.
* @property project The ID or URL-encoded path of a project to change MR approval configuration.
* @property requirePasswordToApprove Set to `true` to require authentication to approve merge requests.
* @property resetApprovalsOnPush Set to `true` to remove all approvals in a merge request when new commits are pushed to its source branch. Default is `true`.
* @property selectiveCodeOwnerRemovals Reset approvals from Code Owners if their files changed. Can be enabled only if reset*approvals*on_push is disabled.
*/
public data class ProjectLevelMrApprovalsArgs(
public val disableOverridingApproversPerMergeRequest: Output? = null,
public val mergeRequestsAuthorApproval: Output? = null,
public val mergeRequestsDisableCommittersApproval: Output? = null,
public val project: Output? = null,
public val requirePasswordToApprove: Output? = null,
public val resetApprovalsOnPush: Output? = null,
public val selectiveCodeOwnerRemovals: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.ProjectLevelMrApprovalsArgs =
com.pulumi.gitlab.ProjectLevelMrApprovalsArgs.builder()
.disableOverridingApproversPerMergeRequest(
disableOverridingApproversPerMergeRequest?.applyValue({ args0 ->
args0
}),
)
.mergeRequestsAuthorApproval(mergeRequestsAuthorApproval?.applyValue({ args0 -> args0 }))
.mergeRequestsDisableCommittersApproval(
mergeRequestsDisableCommittersApproval?.applyValue({ args0 ->
args0
}),
)
.project(project?.applyValue({ args0 -> args0 }))
.requirePasswordToApprove(requirePasswordToApprove?.applyValue({ args0 -> args0 }))
.resetApprovalsOnPush(resetApprovalsOnPush?.applyValue({ args0 -> args0 }))
.selectiveCodeOwnerRemovals(selectiveCodeOwnerRemovals?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectLevelMrApprovalsArgs].
*/
@PulumiTagMarker
public class ProjectLevelMrApprovalsArgsBuilder internal constructor() {
private var disableOverridingApproversPerMergeRequest: Output? = null
private var mergeRequestsAuthorApproval: Output? = null
private var mergeRequestsDisableCommittersApproval: Output? = null
private var project: Output? = null
private var requirePasswordToApprove: Output? = null
private var resetApprovalsOnPush: Output? = null
private var selectiveCodeOwnerRemovals: Output? = null
/**
* @param value Set to `true` to disable overriding approvers per merge request.
*/
@JvmName("vcgvybnpojgblchm")
public suspend fun disableOverridingApproversPerMergeRequest(`value`: Output) {
this.disableOverridingApproversPerMergeRequest = value
}
/**
* @param value Set to `true` to allow merge requests authors to approve their own merge requests.
*/
@JvmName("fwdfffpualovgbsq")
public suspend fun mergeRequestsAuthorApproval(`value`: Output) {
this.mergeRequestsAuthorApproval = value
}
/**
* @param value Set to `true` to disable merge request committers from approving their own merge requests.
*/
@JvmName("kffajguiihkrwjlx")
public suspend fun mergeRequestsDisableCommittersApproval(`value`: Output) {
this.mergeRequestsDisableCommittersApproval = value
}
/**
* @param value The ID or URL-encoded path of a project to change MR approval configuration.
*/
@JvmName("pvslylkyjxsewols")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Set to `true` to require authentication to approve merge requests.
*/
@JvmName("bttmupfhrrtdxnxb")
public suspend fun requirePasswordToApprove(`value`: Output) {
this.requirePasswordToApprove = value
}
/**
* @param value Set to `true` to remove all approvals in a merge request when new commits are pushed to its source branch. Default is `true`.
*/
@JvmName("xosnjputbmvergbs")
public suspend fun resetApprovalsOnPush(`value`: Output) {
this.resetApprovalsOnPush = value
}
/**
* @param value Reset approvals from Code Owners if their files changed. Can be enabled only if reset*approvals*on_push is disabled.
*/
@JvmName("pifgvcamivuinhqb")
public suspend fun selectiveCodeOwnerRemovals(`value`: Output) {
this.selectiveCodeOwnerRemovals = value
}
/**
* @param value Set to `true` to disable overriding approvers per merge request.
*/
@JvmName("dpfyhrydtbrhouvv")
public suspend fun disableOverridingApproversPerMergeRequest(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableOverridingApproversPerMergeRequest = mapped
}
/**
* @param value Set to `true` to allow merge requests authors to approve their own merge requests.
*/
@JvmName("qtsojfoacuaaekbn")
public suspend fun mergeRequestsAuthorApproval(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mergeRequestsAuthorApproval = mapped
}
/**
* @param value Set to `true` to disable merge request committers from approving their own merge requests.
*/
@JvmName("rimspsuwctgssfkd")
public suspend fun mergeRequestsDisableCommittersApproval(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mergeRequestsDisableCommittersApproval = mapped
}
/**
* @param value The ID or URL-encoded path of a project to change MR approval configuration.
*/
@JvmName("eiswfglrwqgtaqws")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Set to `true` to require authentication to approve merge requests.
*/
@JvmName("icbmbclxqohuskoc")
public suspend fun requirePasswordToApprove(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requirePasswordToApprove = mapped
}
/**
* @param value Set to `true` to remove all approvals in a merge request when new commits are pushed to its source branch. Default is `true`.
*/
@JvmName("jvkayblefiyvayuq")
public suspend fun resetApprovalsOnPush(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resetApprovalsOnPush = mapped
}
/**
* @param value Reset approvals from Code Owners if their files changed. Can be enabled only if reset*approvals*on_push is disabled.
*/
@JvmName("sqihltbqwxjgoreo")
public suspend fun selectiveCodeOwnerRemovals(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selectiveCodeOwnerRemovals = mapped
}
internal fun build(): ProjectLevelMrApprovalsArgs = ProjectLevelMrApprovalsArgs(
disableOverridingApproversPerMergeRequest = disableOverridingApproversPerMergeRequest,
mergeRequestsAuthorApproval = mergeRequestsAuthorApproval,
mergeRequestsDisableCommittersApproval = mergeRequestsDisableCommittersApproval,
project = project,
requirePasswordToApprove = requirePasswordToApprove,
resetApprovalsOnPush = resetApprovalsOnPush,
selectiveCodeOwnerRemovals = selectiveCodeOwnerRemovals,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy