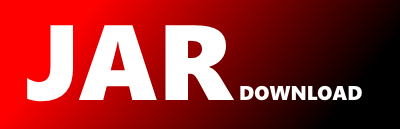
com.pulumi.gitlab.kotlin.Runner.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [Runner].
*/
@PulumiTagMarker
public class RunnerResourceBuilder internal constructor() {
public var name: String? = null
public var args: RunnerArgs = RunnerArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RunnerArgsBuilder.() -> Unit) {
val builder = RunnerArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Runner {
val builtJavaResource = com.pulumi.gitlab.Runner(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Runner(builtJavaResource)
}
}
/**
* The `gitlab.Runner` resource allows to manage the lifecycle of a runner.
* A runner can either be registered at an instance level or group level.
* The runner will be registered at a group level if the token used is from a group, or at an instance level if the token used is for the instance.
* ~ > Using this resource will register a runner using the deprecated `registration_token` flow. To use the new `authentication_token` flow instead,
* use the `gitlab.UserRunner` resource!
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/runners.html#register-a-new-runner)
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_runner`. For example:
* terraform
* import {
* to = gitlab_runner.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* A GitLab Runner can be imported using the runner's ID, eg
* ```sh
* $ pulumi import gitlab:index/runner:Runner this 1
* ```
*/
public class Runner internal constructor(
override val javaResource: com.pulumi.gitlab.Runner,
) : KotlinCustomResource(javaResource, RunnerMapper) {
/**
* The access_level of the runner. Valid values are: `not_protected`, `ref_protected`.
*/
public val accessLevel: Output
get() = javaResource.accessLevel().applyValue({ args0 -> args0 })
/**
* The authentication token used for building a config.toml file. This value is not present when imported.
*/
public val authenticationToken: Output
get() = javaResource.authenticationToken().applyValue({ args0 -> args0 })
/**
* The runner's description.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether the runner should be locked for current project.
*/
public val locked: Output
get() = javaResource.locked().applyValue({ args0 -> args0 })
/**
* Free-form maintenance notes for the runner (1024 characters).
*/
public val maintenanceNote: Output?
get() = javaResource.maintenanceNote().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Maximum timeout set when this runner handles the job.
*/
public val maximumTimeout: Output?
get() = javaResource.maximumTimeout().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether the runner should ignore new jobs.
*/
public val paused: Output
get() = javaResource.paused().applyValue({ args0 -> args0 })
/**
* The registration token used to register the runner.
*/
public val registrationToken: Output
get() = javaResource.registrationToken().applyValue({ args0 -> args0 })
/**
* Whether the runner should handle untagged jobs.
*/
public val runUntagged: Output
get() = javaResource.runUntagged().applyValue({ args0 -> args0 })
/**
* The status of runners to show, one of: online and offline. active and paused are also possible values
* which were deprecated in GitLab 14.8 and will be removed in GitLab 16.0.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
/**
* List of runner’s tags.
*/
public val tagLists: Output>?
get() = javaResource.tagLists().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
}
public object RunnerMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.Runner::class == javaResource::class
override fun map(javaResource: Resource): Runner = Runner(
javaResource as
com.pulumi.gitlab.Runner,
)
}
/**
* @see [Runner].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Runner].
*/
public suspend fun runner(name: String, block: suspend RunnerResourceBuilder.() -> Unit): Runner {
val builder = RunnerResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Runner].
* @param name The _unique_ name of the resulting resource.
*/
public fun runner(name: String): Runner {
val builder = RunnerResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy