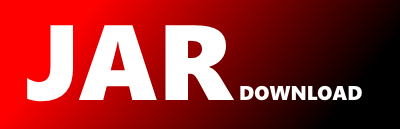
com.pulumi.gitlab.kotlin.Topic.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Topic].
*/
@PulumiTagMarker
public class TopicResourceBuilder internal constructor() {
public var name: String? = null
public var args: TopicArgs = TopicArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TopicArgsBuilder.() -> Unit) {
val builder = TopicArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Topic {
val builtJavaResource = com.pulumi.gitlab.Topic(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Topic(builtJavaResource)
}
}
/**
* The `gitlab.Topic` resource allows to manage the lifecycle of topics that are then assignable to projects.
* > Topics are the successors for project tags. Aside from avoiding terminology collisions with Git tags, they are more descriptive and better searchable.
* > Deleting a topic was implemented in GitLab 14.9. For older versions of GitLab set `soft_destroy = true` to empty out a topic instead of deleting it.
* **Upstream API**: [GitLab REST API docs for topics](https://docs.gitlab.com/ee/api/topics.html)
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_topic`. For example:
* terraform
* import {
* to = gitlab_topic.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* ```sh
* $ pulumi import gitlab:index/topic:Topic You can import a topic to terraform state using ` `.
* ```
* The `id` must be an integer for the id of the topic you want to import,
* for example:
* ```sh
* $ pulumi import gitlab:index/topic:Topic functional_programming 1
* ```
*/
public class Topic internal constructor(
override val javaResource: com.pulumi.gitlab.Topic,
) : KotlinCustomResource(javaResource, TopicMapper) {
/**
* A local path to the avatar image to upload. **Note**: not available for imported resources.
*/
public val avatar: Output?
get() = javaResource.avatar().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The hash of the avatar image. Use `filesha256("path/to/avatar.png")` whenever possible. **Note**: this is used to trigger an update of the avatar. If it's not given, but an avatar is given, the avatar will be updated each time.
*/
public val avatarHash: Output
get() = javaResource.avatarHash().applyValue({ args0 -> args0 })
/**
* The URL of the avatar image.
*/
public val avatarUrl: Output
get() = javaResource.avatarUrl().applyValue({ args0 -> args0 })
/**
* A text describing the topic.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The topic's name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Empty the topics fields instead of deleting it.
*/
@Deprecated(
message = """
GitLab 14.9 introduced the proper deletion of topics. This field is no longer needed.
""",
)
public val softDestroy: Output?
get() = javaResource.softDestroy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The topic's description. Requires at least GitLab 15.0 for which it's a required argument.
*/
public val title: Output?
get() = javaResource.title().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object TopicMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.Topic::class == javaResource::class
override fun map(javaResource: Resource): Topic = Topic(javaResource as com.pulumi.gitlab.Topic)
}
/**
* @see [Topic].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Topic].
*/
public suspend fun topic(name: String, block: suspend TopicResourceBuilder.() -> Unit): Topic {
val builder = TopicResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Topic].
* @param name The _unique_ name of the resulting resource.
*/
public fun topic(name: String): Topic {
val builder = TopicResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy