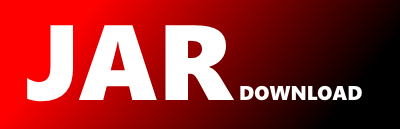
com.pulumi.gitlab.kotlin.UserArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.UserArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.User` resource allows to manage the lifecycle of a user.
* > the provider needs to be configured with admin-level access for this resource to work.
* > You must specify either password or reset_password.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/users.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const example = new gitlab.User("example", {
* name: "Example Foo",
* username: "example",
* password: "superPassword",
* email: "[email protected]",
* isAdmin: true,
* projectsLimit: 4,
* canCreateGroup: false,
* isExternal: true,
* resetPassword: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* example = gitlab.User("example",
* name="Example Foo",
* username="example",
* password="superPassword",
* email="[email protected]",
* is_admin=True,
* projects_limit=4,
* can_create_group=False,
* is_external=True,
* reset_password=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var example = new GitLab.User("example", new()
* {
* Name = "Example Foo",
* Username = "example",
* Password = "superPassword",
* Email = "[email protected]",
* IsAdmin = true,
* ProjectsLimit = 4,
* CanCreateGroup = false,
* IsExternal = true,
* ResetPassword = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewUser(ctx, "example", &gitlab.UserArgs{
* Name: pulumi.String("Example Foo"),
* Username: pulumi.String("example"),
* Password: pulumi.String("superPassword"),
* Email: pulumi.String("[email protected]"),
* IsAdmin: pulumi.Bool(true),
* ProjectsLimit: pulumi.Int(4),
* CanCreateGroup: pulumi.Bool(false),
* IsExternal: pulumi.Bool(true),
* ResetPassword: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.User;
* import com.pulumi.gitlab.UserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new User("example", UserArgs.builder()
* .name("Example Foo")
* .username("example")
* .password("superPassword")
* .email("[email protected]")
* .isAdmin(true)
* .projectsLimit(4)
* .canCreateGroup(false)
* .isExternal(true)
* .resetPassword(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gitlab:User
* properties:
* name: Example Foo
* username: example
* password: superPassword
* email: [email protected]
* isAdmin: true
* projectsLimit: 4
* canCreateGroup: false
* isExternal: true
* resetPassword: false
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_user`. For example:
* terraform
* import {
* to = gitlab_user.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* ```sh
* $ pulumi import gitlab:index/user:User You can import a user to terraform state using ` `.
* ```
* The `id` must be an integer for the id of the user you want to import,
* for example:
* ```sh
* $ pulumi import gitlab:index/user:User example 42
* ```
* @property canCreateGroup Boolean, defaults to false. Whether to allow the user to create groups.
* @property email The e-mail address of the user.
* @property externUid String, a specific external authentication provider UID.
* @property externalProvider String, the external provider.
* @property isAdmin Boolean, defaults to false. Whether to enable administrative privileges
* @property isExternal Boolean, defaults to false. Whether a user has access only to some internal or private projects. External users can only access projects to which they are explicitly granted access.
* @property name The name of the user.
* @property namespaceId The ID of the user's namespace. Available since GitLab 14.10.
* @property note The note associated to the user.
* @property password The password of the user.
* @property projectsLimit Integer, defaults to 0. Number of projects user can create.
* @property resetPassword Boolean, defaults to false. Send user password reset link.
* @property skipConfirmation Boolean, defaults to true. Whether to skip confirmation.
* @property state String, defaults to 'active'. The state of the user account. Valid values are `active`, `deactivated`, `blocked`.
* @property username The username of the user.
*/
public data class UserArgs(
public val canCreateGroup: Output? = null,
public val email: Output? = null,
public val externUid: Output? = null,
public val externalProvider: Output? = null,
public val isAdmin: Output? = null,
public val isExternal: Output? = null,
public val name: Output? = null,
public val namespaceId: Output? = null,
public val note: Output? = null,
public val password: Output? = null,
public val projectsLimit: Output? = null,
public val resetPassword: Output? = null,
public val skipConfirmation: Output? = null,
public val state: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.UserArgs = com.pulumi.gitlab.UserArgs.builder()
.canCreateGroup(canCreateGroup?.applyValue({ args0 -> args0 }))
.email(email?.applyValue({ args0 -> args0 }))
.externUid(externUid?.applyValue({ args0 -> args0 }))
.externalProvider(externalProvider?.applyValue({ args0 -> args0 }))
.isAdmin(isAdmin?.applyValue({ args0 -> args0 }))
.isExternal(isExternal?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespaceId(namespaceId?.applyValue({ args0 -> args0 }))
.note(note?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.projectsLimit(projectsLimit?.applyValue({ args0 -> args0 }))
.resetPassword(resetPassword?.applyValue({ args0 -> args0 }))
.skipConfirmation(skipConfirmation?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserArgs].
*/
@PulumiTagMarker
public class UserArgsBuilder internal constructor() {
private var canCreateGroup: Output? = null
private var email: Output? = null
private var externUid: Output? = null
private var externalProvider: Output? = null
private var isAdmin: Output? = null
private var isExternal: Output? = null
private var name: Output? = null
private var namespaceId: Output? = null
private var note: Output? = null
private var password: Output? = null
private var projectsLimit: Output? = null
private var resetPassword: Output? = null
private var skipConfirmation: Output? = null
private var state: Output? = null
private var username: Output? = null
/**
* @param value Boolean, defaults to false. Whether to allow the user to create groups.
*/
@JvmName("jwcvuslrfcaxkhrb")
public suspend fun canCreateGroup(`value`: Output) {
this.canCreateGroup = value
}
/**
* @param value The e-mail address of the user.
*/
@JvmName("oyfcdyuttartkkti")
public suspend fun email(`value`: Output) {
this.email = value
}
/**
* @param value String, a specific external authentication provider UID.
*/
@JvmName("cuhvewnfbjyhfgut")
public suspend fun externUid(`value`: Output) {
this.externUid = value
}
/**
* @param value String, the external provider.
*/
@JvmName("hxsvxlbbwtkkoiya")
public suspend fun externalProvider(`value`: Output) {
this.externalProvider = value
}
/**
* @param value Boolean, defaults to false. Whether to enable administrative privileges
*/
@JvmName("kwebqnmbrgiedpxt")
public suspend fun isAdmin(`value`: Output) {
this.isAdmin = value
}
/**
* @param value Boolean, defaults to false. Whether a user has access only to some internal or private projects. External users can only access projects to which they are explicitly granted access.
*/
@JvmName("dutnkvtsssmsqdtr")
public suspend fun isExternal(`value`: Output) {
this.isExternal = value
}
/**
* @param value The name of the user.
*/
@JvmName("itspghdomvyxeswn")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID of the user's namespace. Available since GitLab 14.10.
*/
@JvmName("ghfjvnhpwacocjml")
public suspend fun namespaceId(`value`: Output) {
this.namespaceId = value
}
/**
* @param value The note associated to the user.
*/
@JvmName("luxxokedbkmocgnu")
public suspend fun note(`value`: Output) {
this.note = value
}
/**
* @param value The password of the user.
*/
@JvmName("apksxcnwjnapmqve")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Integer, defaults to 0. Number of projects user can create.
*/
@JvmName("elnootwtipefrnso")
public suspend fun projectsLimit(`value`: Output) {
this.projectsLimit = value
}
/**
* @param value Boolean, defaults to false. Send user password reset link.
*/
@JvmName("reqlqcafmlwusuyi")
public suspend fun resetPassword(`value`: Output) {
this.resetPassword = value
}
/**
* @param value Boolean, defaults to true. Whether to skip confirmation.
*/
@JvmName("yvtwqcbkwauynsyv")
public suspend fun skipConfirmation(`value`: Output) {
this.skipConfirmation = value
}
/**
* @param value String, defaults to 'active'. The state of the user account. Valid values are `active`, `deactivated`, `blocked`.
*/
@JvmName("aveecdglnrcwrmir")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value The username of the user.
*/
@JvmName("mukkkamxiwfyamjq")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Boolean, defaults to false. Whether to allow the user to create groups.
*/
@JvmName("canhceiushfymsua")
public suspend fun canCreateGroup(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.canCreateGroup = mapped
}
/**
* @param value The e-mail address of the user.
*/
@JvmName("mgguhjgesqclxixh")
public suspend fun email(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.email = mapped
}
/**
* @param value String, a specific external authentication provider UID.
*/
@JvmName("sfwhyldsqnqnnrnt")
public suspend fun externUid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externUid = mapped
}
/**
* @param value String, the external provider.
*/
@JvmName("xjsejtejfwgpnluc")
public suspend fun externalProvider(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalProvider = mapped
}
/**
* @param value Boolean, defaults to false. Whether to enable administrative privileges
*/
@JvmName("ovublqqqoggcthpj")
public suspend fun isAdmin(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isAdmin = mapped
}
/**
* @param value Boolean, defaults to false. Whether a user has access only to some internal or private projects. External users can only access projects to which they are explicitly granted access.
*/
@JvmName("mmxgiewlxeamymcn")
public suspend fun isExternal(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isExternal = mapped
}
/**
* @param value The name of the user.
*/
@JvmName("gbwewdwkiuybkeyf")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ID of the user's namespace. Available since GitLab 14.10.
*/
@JvmName("vpobfkyqfbqhauvo")
public suspend fun namespaceId(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespaceId = mapped
}
/**
* @param value The note associated to the user.
*/
@JvmName("kecrlvdsmthfptlb")
public suspend fun note(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.note = mapped
}
/**
* @param value The password of the user.
*/
@JvmName("jfxojtvssibajqgb")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Integer, defaults to 0. Number of projects user can create.
*/
@JvmName("jyrnvxjtmbxsouyj")
public suspend fun projectsLimit(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.projectsLimit = mapped
}
/**
* @param value Boolean, defaults to false. Send user password reset link.
*/
@JvmName("bwcxwyftdkxxfbqe")
public suspend fun resetPassword(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resetPassword = mapped
}
/**
* @param value Boolean, defaults to true. Whether to skip confirmation.
*/
@JvmName("abupiqwhbcbayujf")
public suspend fun skipConfirmation(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skipConfirmation = mapped
}
/**
* @param value String, defaults to 'active'. The state of the user account. Valid values are `active`, `deactivated`, `blocked`.
*/
@JvmName("hobxubdqbxfqsnbm")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
/**
* @param value The username of the user.
*/
@JvmName("cdewcjdrnlplaysh")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): UserArgs = UserArgs(
canCreateGroup = canCreateGroup,
email = email,
externUid = externUid,
externalProvider = externalProvider,
isAdmin = isAdmin,
isExternal = isExternal,
name = name,
namespaceId = namespaceId,
note = note,
password = password,
projectsLimit = projectsLimit,
resetPassword = resetPassword,
skipConfirmation = skipConfirmation,
state = state,
username = username,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy