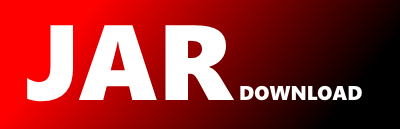
com.pulumi.gitlab.kotlin.inputs.GetGroupSubgroupsPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin.inputs
import com.pulumi.gitlab.inputs.GetGroupSubgroupsPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getGroupSubgroups.
* @property allAvailable Show all the groups you have access to.
* @property groupId The ID of the group.
* @property minAccessLevel Limit to groups where current user has at least this access level.
* @property orderBy Order groups by name, path or id.
* @property owned Limit to groups explicitly owned by the current user.
* @property search Return the list of authorized groups matching the search criteria.
* @property skipGroups Skip the group IDs passed.
* @property sort Order groups in asc or desc order.
* @property statistics Include group statistics (administrators only).
* @property withCustomAttributes Include custom attributes in response (administrators only).
*/
public data class GetGroupSubgroupsPlainArgs(
public val allAvailable: Boolean? = null,
public val groupId: Int,
public val minAccessLevel: String? = null,
public val orderBy: String? = null,
public val owned: Boolean? = null,
public val search: String? = null,
public val skipGroups: List? = null,
public val sort: String? = null,
public val statistics: Boolean? = null,
public val withCustomAttributes: Boolean? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.inputs.GetGroupSubgroupsPlainArgs =
com.pulumi.gitlab.inputs.GetGroupSubgroupsPlainArgs.builder()
.allAvailable(allAvailable?.let({ args0 -> args0 }))
.groupId(groupId.let({ args0 -> args0 }))
.minAccessLevel(minAccessLevel?.let({ args0 -> args0 }))
.orderBy(orderBy?.let({ args0 -> args0 }))
.owned(owned?.let({ args0 -> args0 }))
.search(search?.let({ args0 -> args0 }))
.skipGroups(skipGroups?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.sort(sort?.let({ args0 -> args0 }))
.statistics(statistics?.let({ args0 -> args0 }))
.withCustomAttributes(withCustomAttributes?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetGroupSubgroupsPlainArgs].
*/
@PulumiTagMarker
public class GetGroupSubgroupsPlainArgsBuilder internal constructor() {
private var allAvailable: Boolean? = null
private var groupId: Int? = null
private var minAccessLevel: String? = null
private var orderBy: String? = null
private var owned: Boolean? = null
private var search: String? = null
private var skipGroups: List? = null
private var sort: String? = null
private var statistics: Boolean? = null
private var withCustomAttributes: Boolean? = null
/**
* @param value Show all the groups you have access to.
*/
@JvmName("ykucvlrcigufcowp")
public suspend fun allAvailable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.allAvailable = mapped
}
/**
* @param value The ID of the group.
*/
@JvmName("xqgwtplfsscvcdeu")
public suspend fun groupId(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.groupId = mapped
}
/**
* @param value Limit to groups where current user has at least this access level.
*/
@JvmName("gxggnolcufoxbdfx")
public suspend fun minAccessLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.minAccessLevel = mapped
}
/**
* @param value Order groups by name, path or id.
*/
@JvmName("tokqfvixsjrdoojk")
public suspend fun orderBy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.orderBy = mapped
}
/**
* @param value Limit to groups explicitly owned by the current user.
*/
@JvmName("fbcfcjkickpiydsa")
public suspend fun owned(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.owned = mapped
}
/**
* @param value Return the list of authorized groups matching the search criteria.
*/
@JvmName("ttmpvjvydoghmcmf")
public suspend fun search(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.search = mapped
}
/**
* @param value Skip the group IDs passed.
*/
@JvmName("oklibefwtaxurhdt")
public suspend fun skipGroups(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.skipGroups = mapped
}
/**
* @param values Skip the group IDs passed.
*/
@JvmName("kfnssshjsywkfalv")
public suspend fun skipGroups(vararg values: Int) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.skipGroups = mapped
}
/**
* @param value Order groups in asc or desc order.
*/
@JvmName("vuqsxmiddqggiwwu")
public suspend fun sort(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.sort = mapped
}
/**
* @param value Include group statistics (administrators only).
*/
@JvmName("qycxlcfcgtecntqf")
public suspend fun statistics(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.statistics = mapped
}
/**
* @param value Include custom attributes in response (administrators only).
*/
@JvmName("wwuvxieedgekwiqo")
public suspend fun withCustomAttributes(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.withCustomAttributes = mapped
}
internal fun build(): GetGroupSubgroupsPlainArgs = GetGroupSubgroupsPlainArgs(
allAvailable = allAvailable,
groupId = groupId ?: throw PulumiNullFieldException("groupId"),
minAccessLevel = minAccessLevel,
orderBy = orderBy,
owned = owned,
search = search,
skipGroups = skipGroups,
sort = sort,
statistics = statistics,
withCustomAttributes = withCustomAttributes,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy