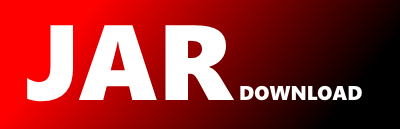
com.pulumi.gitlab.kotlin.inputs.GetUsersPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin.inputs
import com.pulumi.gitlab.inputs.GetUsersPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getUsers.
* @property active Filter users that are active.
* @property blocked Filter users that are blocked.
* @property createdAfter Search for users created after a specific date. (Requires administrator privileges)
* @property createdBefore Search for users created before a specific date. (Requires administrator privileges)
* @property externProvider Lookup users by external provider. (Requires administrator privileges)
* @property externUid Lookup users by external UID. (Requires administrator privileges)
* @property orderBy Order the users' list by `id`, `name`, `username`, `created_at` or `updated_at`. (Requires administrator privileges)
* @property search Search users by username, name or email.
* @property sort Sort users' list in asc or desc order. (Requires administrator privileges)
*/
public data class GetUsersPlainArgs(
public val active: Boolean? = null,
public val blocked: Boolean? = null,
public val createdAfter: String? = null,
public val createdBefore: String? = null,
public val externProvider: String? = null,
public val externUid: String? = null,
public val orderBy: String? = null,
public val search: String? = null,
public val sort: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.inputs.GetUsersPlainArgs =
com.pulumi.gitlab.inputs.GetUsersPlainArgs.builder()
.active(active?.let({ args0 -> args0 }))
.blocked(blocked?.let({ args0 -> args0 }))
.createdAfter(createdAfter?.let({ args0 -> args0 }))
.createdBefore(createdBefore?.let({ args0 -> args0 }))
.externProvider(externProvider?.let({ args0 -> args0 }))
.externUid(externUid?.let({ args0 -> args0 }))
.orderBy(orderBy?.let({ args0 -> args0 }))
.search(search?.let({ args0 -> args0 }))
.sort(sort?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetUsersPlainArgs].
*/
@PulumiTagMarker
public class GetUsersPlainArgsBuilder internal constructor() {
private var active: Boolean? = null
private var blocked: Boolean? = null
private var createdAfter: String? = null
private var createdBefore: String? = null
private var externProvider: String? = null
private var externUid: String? = null
private var orderBy: String? = null
private var search: String? = null
private var sort: String? = null
/**
* @param value Filter users that are active.
*/
@JvmName("dsrbtvvuaaebvfqh")
public suspend fun active(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.active = mapped
}
/**
* @param value Filter users that are blocked.
*/
@JvmName("gwoojibxmhustmar")
public suspend fun blocked(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.blocked = mapped
}
/**
* @param value Search for users created after a specific date. (Requires administrator privileges)
*/
@JvmName("fxtxfsafxvsthbce")
public suspend fun createdAfter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.createdAfter = mapped
}
/**
* @param value Search for users created before a specific date. (Requires administrator privileges)
*/
@JvmName("nulircnobwhcuthl")
public suspend fun createdBefore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.createdBefore = mapped
}
/**
* @param value Lookup users by external provider. (Requires administrator privileges)
*/
@JvmName("ikuhtxdcsgufxtmm")
public suspend fun externProvider(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.externProvider = mapped
}
/**
* @param value Lookup users by external UID. (Requires administrator privileges)
*/
@JvmName("uydvduxtajlvrmhk")
public suspend fun externUid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.externUid = mapped
}
/**
* @param value Order the users' list by `id`, `name`, `username`, `created_at` or `updated_at`. (Requires administrator privileges)
*/
@JvmName("mfitnarehvjummcl")
public suspend fun orderBy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.orderBy = mapped
}
/**
* @param value Search users by username, name or email.
*/
@JvmName("lhpikeivqnstjbef")
public suspend fun search(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.search = mapped
}
/**
* @param value Sort users' list in asc or desc order. (Requires administrator privileges)
*/
@JvmName("supwhhooihuagkpq")
public suspend fun sort(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.sort = mapped
}
internal fun build(): GetUsersPlainArgs = GetUsersPlainArgs(
active = active,
blocked = blocked,
createdAfter = createdAfter,
createdBefore = createdBefore,
externProvider = externProvider,
externUid = externUid,
orderBy = orderBy,
search = search,
sort = sort,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy