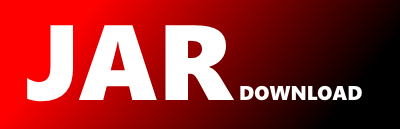
com.pulumi.gitlab.kotlin.inputs.ProjectContainerExpirationPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.inputs.ProjectContainerExpirationPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property cadence The cadence of the policy. Valid values are: `1d`, `7d`, `14d`, `1month`, `3month`.
* @property enabled If true, the policy is enabled.
* @property keepN The number of images to keep.
* @property nameRegex The regular expression to match image names to delete.
* @property nameRegexDelete The regular expression to match image names to delete.
* @property nameRegexKeep The regular expression to match image names to keep.
* @property nextRunAt The next time the policy will run.
* @property olderThan The number of days to keep images.
*/
public data class ProjectContainerExpirationPolicyArgs(
public val cadence: Output? = null,
public val enabled: Output? = null,
public val keepN: Output? = null,
@Deprecated(
message = """
`name_regex` has been deprecated. Use `name_regex_delete` instead.
""",
)
public val nameRegex: Output? = null,
public val nameRegexDelete: Output? = null,
public val nameRegexKeep: Output? = null,
public val nextRunAt: Output? = null,
public val olderThan: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.inputs.ProjectContainerExpirationPolicyArgs =
com.pulumi.gitlab.inputs.ProjectContainerExpirationPolicyArgs.builder()
.cadence(cadence?.applyValue({ args0 -> args0 }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.keepN(keepN?.applyValue({ args0 -> args0 }))
.nameRegex(nameRegex?.applyValue({ args0 -> args0 }))
.nameRegexDelete(nameRegexDelete?.applyValue({ args0 -> args0 }))
.nameRegexKeep(nameRegexKeep?.applyValue({ args0 -> args0 }))
.nextRunAt(nextRunAt?.applyValue({ args0 -> args0 }))
.olderThan(olderThan?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectContainerExpirationPolicyArgs].
*/
@PulumiTagMarker
public class ProjectContainerExpirationPolicyArgsBuilder internal constructor() {
private var cadence: Output? = null
private var enabled: Output? = null
private var keepN: Output? = null
private var nameRegex: Output? = null
private var nameRegexDelete: Output? = null
private var nameRegexKeep: Output? = null
private var nextRunAt: Output? = null
private var olderThan: Output? = null
/**
* @param value The cadence of the policy. Valid values are: `1d`, `7d`, `14d`, `1month`, `3month`.
*/
@JvmName("pqakjinyycnfbyik")
public suspend fun cadence(`value`: Output) {
this.cadence = value
}
/**
* @param value If true, the policy is enabled.
*/
@JvmName("awsuwbwqadcdhiny")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value The number of images to keep.
*/
@JvmName("ecekhhtutepydvho")
public suspend fun keepN(`value`: Output) {
this.keepN = value
}
/**
* @param value The regular expression to match image names to delete.
*/
@Deprecated(
message = """
`name_regex` has been deprecated. Use `name_regex_delete` instead.
""",
)
@JvmName("nlvadrjeytqnlgts")
public suspend fun nameRegex(`value`: Output) {
this.nameRegex = value
}
/**
* @param value The regular expression to match image names to delete.
*/
@JvmName("sbcrmwvbwcmxuflu")
public suspend fun nameRegexDelete(`value`: Output) {
this.nameRegexDelete = value
}
/**
* @param value The regular expression to match image names to keep.
*/
@JvmName("yoayrttfayvcmrdx")
public suspend fun nameRegexKeep(`value`: Output) {
this.nameRegexKeep = value
}
/**
* @param value The next time the policy will run.
*/
@JvmName("liakycfutkfdkybq")
public suspend fun nextRunAt(`value`: Output) {
this.nextRunAt = value
}
/**
* @param value The number of days to keep images.
*/
@JvmName("uhkvicuaogfxslut")
public suspend fun olderThan(`value`: Output) {
this.olderThan = value
}
/**
* @param value The cadence of the policy. Valid values are: `1d`, `7d`, `14d`, `1month`, `3month`.
*/
@JvmName("cxeumcrxwwriwbxe")
public suspend fun cadence(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cadence = mapped
}
/**
* @param value If true, the policy is enabled.
*/
@JvmName("flcywwhfhcpipcuf")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value The number of images to keep.
*/
@JvmName("hlvlgcvdibslgfjy")
public suspend fun keepN(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keepN = mapped
}
/**
* @param value The regular expression to match image names to delete.
*/
@Deprecated(
message = """
`name_regex` has been deprecated. Use `name_regex_delete` instead.
""",
)
@JvmName("ybamxiifnnubmkai")
public suspend fun nameRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nameRegex = mapped
}
/**
* @param value The regular expression to match image names to delete.
*/
@JvmName("uvnbpspdxtibioey")
public suspend fun nameRegexDelete(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nameRegexDelete = mapped
}
/**
* @param value The regular expression to match image names to keep.
*/
@JvmName("djrpyacmpqkgvwtu")
public suspend fun nameRegexKeep(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nameRegexKeep = mapped
}
/**
* @param value The next time the policy will run.
*/
@JvmName("atideuintloikdxj")
public suspend fun nextRunAt(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nextRunAt = mapped
}
/**
* @param value The number of days to keep images.
*/
@JvmName("aixgukqsmbapbdbk")
public suspend fun olderThan(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.olderThan = mapped
}
internal fun build(): ProjectContainerExpirationPolicyArgs = ProjectContainerExpirationPolicyArgs(
cadence = cadence,
enabled = enabled,
keepN = keepN,
nameRegex = nameRegex,
nameRegexDelete = nameRegexDelete,
nameRegexKeep = nameRegexKeep,
nextRunAt = nextRunAt,
olderThan = olderThan,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy