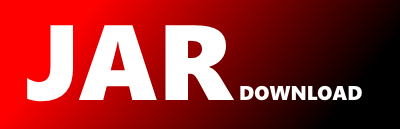
com.pulumi.gitlab.kotlin.UserImpersonationToken.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [UserImpersonationToken].
*/
@PulumiTagMarker
public class UserImpersonationTokenResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserImpersonationTokenArgs = UserImpersonationTokenArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserImpersonationTokenArgsBuilder.() -> Unit) {
val builder = UserImpersonationTokenArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): UserImpersonationToken {
val builtJavaResource = com.pulumi.gitlab.UserImpersonationToken(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return UserImpersonationToken(builtJavaResource)
}
}
/**
* The `gitlab.UserImpersonationToken` resource allows to manage impersonation tokens of users.
* Requires administrator access. Token values are returned once. You are only able to create impersonation tokens to impersonate the user and perform both API calls and Git reads and writes. The user can’t see these tokens in their profile settings page.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/users.html#create-an-impersonation-token)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const _this = new gitlab.UserImpersonationToken("this", {
* userId: 12345,
* name: "token_name",
* scopes: ["api"],
* expiresAt: "2024-08-27",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* this = gitlab.UserImpersonationToken("this",
* user_id=12345,
* name="token_name",
* scopes=["api"],
* expires_at="2024-08-27")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var @this = new GitLab.UserImpersonationToken("this", new()
* {
* UserId = 12345,
* Name = "token_name",
* Scopes = new[]
* {
* "api",
* },
* ExpiresAt = "2024-08-27",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewUserImpersonationToken(ctx, "this", &gitlab.UserImpersonationTokenArgs{
* UserId: pulumi.Int(12345),
* Name: pulumi.String("token_name"),
* Scopes: pulumi.StringArray{
* pulumi.String("api"),
* },
* ExpiresAt: pulumi.String("2024-08-27"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.UserImpersonationToken;
* import com.pulumi.gitlab.UserImpersonationTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var this_ = new UserImpersonationToken("this", UserImpersonationTokenArgs.builder()
* .userId(12345)
* .name("token_name")
* .scopes("api")
* .expiresAt("2024-08-27")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* this:
* type: gitlab:UserImpersonationToken
* properties:
* userId: 12345
* name: token_name
* scopes:
* - api
* expiresAt: 2024-08-27
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_user_impersonation_token`. For example:
* terraform
* import {
* to = gitlab_user_impersonation_token.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* A GitLab User Impersonation Token can be imported using a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/userImpersonationToken:UserImpersonationToken example "12345:1"
* ```
* NOTE: the `token` resource attribute is not available for imported resources as this information cannot be read from the GitLab API.
*/
public class UserImpersonationToken internal constructor(
override val javaResource: com.pulumi.gitlab.UserImpersonationToken,
) : KotlinCustomResource(javaResource, UserImpersonationTokenMapper) {
/**
* True if the token is active.
*/
public val active: Output
get() = javaResource.active().applyValue({ args0 -> args0 })
/**
* Time the token has been created, RFC3339 format.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* Expiration date of the impersonation token in ISO format (YYYY-MM-DD).
*/
public val expiresAt: Output
get() = javaResource.expiresAt().applyValue({ args0 -> args0 })
/**
* True as the token is always an impersonation token.
*/
public val impersonation: Output
get() = javaResource.impersonation().applyValue({ args0 -> args0 })
/**
* The name of the impersonation token.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* True if the token is revoked.
*/
public val revoked: Output
get() = javaResource.revoked().applyValue({ args0 -> args0 })
/**
* Array of scopes of the impersonation token. valid values are: `api`, `read_user`, `read_api`, `read_repository`, `write_repository`, `read_registry`, `write_registry`, `sudo`, `admin_mode`, `create_runner`, `manage_runner`, `ai_features`, `k8s_proxy`, `read_service_ping`
*/
public val scopes: Output>
get() = javaResource.scopes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The token of the user impersonation token. **Note**: the token is not available for imported resources.
*/
public val token: Output
get() = javaResource.token().applyValue({ args0 -> args0 })
/**
* ID of the impersonation token.
*/
public val tokenId: Output
get() = javaResource.tokenId().applyValue({ args0 -> args0 })
/**
* The ID of the user.
*/
public val userId: Output
get() = javaResource.userId().applyValue({ args0 -> args0 })
}
public object UserImpersonationTokenMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.UserImpersonationToken::class == javaResource::class
override fun map(javaResource: Resource): UserImpersonationToken =
UserImpersonationToken(javaResource as com.pulumi.gitlab.UserImpersonationToken)
}
/**
* @see [UserImpersonationToken].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [UserImpersonationToken].
*/
public suspend fun userImpersonationToken(
name: String,
block: suspend UserImpersonationTokenResourceBuilder.() -> Unit,
): UserImpersonationToken {
val builder = UserImpersonationTokenResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [UserImpersonationToken].
* @param name The _unique_ name of the resulting resource.
*/
public fun userImpersonationToken(name: String): UserImpersonationToken {
val builder = UserImpersonationTokenResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy