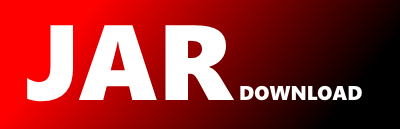
com.pulumi.gitlab.kotlin.UserImpersonationTokenArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.UserImpersonationTokenArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The `gitlab.UserImpersonationToken` resource allows to manage impersonation tokens of users.
* Requires administrator access. Token values are returned once. You are only able to create impersonation tokens to impersonate the user and perform both API calls and Git reads and writes. The user can’t see these tokens in their profile settings page.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/users.html#create-an-impersonation-token)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const _this = new gitlab.UserImpersonationToken("this", {
* userId: 12345,
* name: "token_name",
* scopes: ["api"],
* expiresAt: "2024-08-27",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* this = gitlab.UserImpersonationToken("this",
* user_id=12345,
* name="token_name",
* scopes=["api"],
* expires_at="2024-08-27")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var @this = new GitLab.UserImpersonationToken("this", new()
* {
* UserId = 12345,
* Name = "token_name",
* Scopes = new[]
* {
* "api",
* },
* ExpiresAt = "2024-08-27",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewUserImpersonationToken(ctx, "this", &gitlab.UserImpersonationTokenArgs{
* UserId: pulumi.Int(12345),
* Name: pulumi.String("token_name"),
* Scopes: pulumi.StringArray{
* pulumi.String("api"),
* },
* ExpiresAt: pulumi.String("2024-08-27"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.UserImpersonationToken;
* import com.pulumi.gitlab.UserImpersonationTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var this_ = new UserImpersonationToken("this", UserImpersonationTokenArgs.builder()
* .userId(12345)
* .name("token_name")
* .scopes("api")
* .expiresAt("2024-08-27")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* this:
* type: gitlab:UserImpersonationToken
* properties:
* userId: 12345
* name: token_name
* scopes:
* - api
* expiresAt: 2024-08-27
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_user_impersonation_token`. For example:
* terraform
* import {
* to = gitlab_user_impersonation_token.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* A GitLab User Impersonation Token can be imported using a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/userImpersonationToken:UserImpersonationToken example "12345:1"
* ```
* NOTE: the `token` resource attribute is not available for imported resources as this information cannot be read from the GitLab API.
* @property expiresAt Expiration date of the impersonation token in ISO format (YYYY-MM-DD).
* @property name The name of the impersonation token.
* @property scopes Array of scopes of the impersonation token. valid values are: `api`, `read_user`, `read_api`, `read_repository`, `write_repository`, `read_registry`, `write_registry`, `sudo`, `admin_mode`, `create_runner`, `manage_runner`, `ai_features`, `k8s_proxy`, `read_service_ping`
* @property userId The ID of the user.
*/
public data class UserImpersonationTokenArgs(
public val expiresAt: Output? = null,
public val name: Output? = null,
public val scopes: Output>? = null,
public val userId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.UserImpersonationTokenArgs =
com.pulumi.gitlab.UserImpersonationTokenArgs.builder()
.expiresAt(expiresAt?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.scopes(scopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.userId(userId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserImpersonationTokenArgs].
*/
@PulumiTagMarker
public class UserImpersonationTokenArgsBuilder internal constructor() {
private var expiresAt: Output? = null
private var name: Output? = null
private var scopes: Output>? = null
private var userId: Output? = null
/**
* @param value Expiration date of the impersonation token in ISO format (YYYY-MM-DD).
*/
@JvmName("kjjmjbgeelllrsyb")
public suspend fun expiresAt(`value`: Output) {
this.expiresAt = value
}
/**
* @param value The name of the impersonation token.
*/
@JvmName("bykrkakurrcwmqve")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Array of scopes of the impersonation token. valid values are: `api`, `read_user`, `read_api`, `read_repository`, `write_repository`, `read_registry`, `write_registry`, `sudo`, `admin_mode`, `create_runner`, `manage_runner`, `ai_features`, `k8s_proxy`, `read_service_ping`
*/
@JvmName("rbjfymupfunsafgx")
public suspend fun scopes(`value`: Output>) {
this.scopes = value
}
@JvmName("mqviilndvtvovcyh")
public suspend fun scopes(vararg values: Output) {
this.scopes = Output.all(values.asList())
}
/**
* @param values Array of scopes of the impersonation token. valid values are: `api`, `read_user`, `read_api`, `read_repository`, `write_repository`, `read_registry`, `write_registry`, `sudo`, `admin_mode`, `create_runner`, `manage_runner`, `ai_features`, `k8s_proxy`, `read_service_ping`
*/
@JvmName("kiyxqdbekpsenoae")
public suspend fun scopes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy