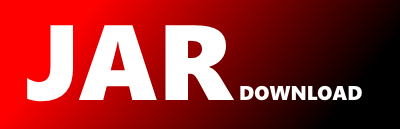
com.pulumi.gitlab.kotlin.inputs.GetGroupProvisionedUsersPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin.inputs
import com.pulumi.gitlab.inputs.GetGroupProvisionedUsersPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getGroupProvisionedUsers.
* @property active Return only active provisioned users.
* @property blocked Return only blocked provisioned users.
* @property createdAfter Return only provisioned users created on or after the specified date. Expected in ISO 8601 format (YYYY-MM-DDTHH:MM:SSZ).
* @property createdBefore Return only provisioned users created on or before the specified date. Expected in ISO 8601 format (YYYY-MM-DDTHH:MM:SSZ).
* @property id The ID or URL-encoded path of the group.
* @property provisionedUsers The list of provisioned users.
* @property search The search query to filter the provisioned users.
* @property username The username of the provisioned user.
*/
public data class GetGroupProvisionedUsersPlainArgs(
public val active: Boolean? = null,
public val blocked: Boolean? = null,
public val createdAfter: String? = null,
public val createdBefore: String? = null,
public val id: String,
public val provisionedUsers: List? = null,
public val search: String? = null,
public val username: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.inputs.GetGroupProvisionedUsersPlainArgs =
com.pulumi.gitlab.inputs.GetGroupProvisionedUsersPlainArgs.builder()
.active(active?.let({ args0 -> args0 }))
.blocked(blocked?.let({ args0 -> args0 }))
.createdAfter(createdAfter?.let({ args0 -> args0 }))
.createdBefore(createdBefore?.let({ args0 -> args0 }))
.id(id.let({ args0 -> args0 }))
.provisionedUsers(
provisionedUsers?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.search(search?.let({ args0 -> args0 }))
.username(username?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetGroupProvisionedUsersPlainArgs].
*/
@PulumiTagMarker
public class GetGroupProvisionedUsersPlainArgsBuilder internal constructor() {
private var active: Boolean? = null
private var blocked: Boolean? = null
private var createdAfter: String? = null
private var createdBefore: String? = null
private var id: String? = null
private var provisionedUsers: List? = null
private var search: String? = null
private var username: String? = null
/**
* @param value Return only active provisioned users.
*/
@JvmName("vawsgdgcmaqtmqgf")
public suspend fun active(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.active = mapped
}
/**
* @param value Return only blocked provisioned users.
*/
@JvmName("ptklygfordpkxati")
public suspend fun blocked(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.blocked = mapped
}
/**
* @param value Return only provisioned users created on or after the specified date. Expected in ISO 8601 format (YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("twqahfhxvukiybee")
public suspend fun createdAfter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.createdAfter = mapped
}
/**
* @param value Return only provisioned users created on or before the specified date. Expected in ISO 8601 format (YYYY-MM-DDTHH:MM:SSZ).
*/
@JvmName("tovbwepeurvhjloa")
public suspend fun createdBefore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.createdBefore = mapped
}
/**
* @param value The ID or URL-encoded path of the group.
*/
@JvmName("tnckvmjbjooaexbg")
public suspend fun id(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.id = mapped
}
/**
* @param value The list of provisioned users.
*/
@JvmName("ftjddcfaectjajbq")
public suspend fun provisionedUsers(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.provisionedUsers = mapped
}
/**
* @param argument The list of provisioned users.
*/
@JvmName("wdkpwidstjghgxab")
public suspend fun provisionedUsers(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetGroupProvisionedUsersProvisionedUserBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.provisionedUsers = mapped
}
/**
* @param argument The list of provisioned users.
*/
@JvmName("lxagijprqdantuix")
public suspend fun provisionedUsers(vararg argument: suspend GetGroupProvisionedUsersProvisionedUserBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetGroupProvisionedUsersProvisionedUserBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.provisionedUsers = mapped
}
/**
* @param argument The list of provisioned users.
*/
@JvmName("mvgkcwkyiddgjcax")
public suspend fun provisionedUsers(argument: suspend GetGroupProvisionedUsersProvisionedUserBuilder.() -> Unit) {
val toBeMapped = listOf(
GetGroupProvisionedUsersProvisionedUserBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.provisionedUsers = mapped
}
/**
* @param values The list of provisioned users.
*/
@JvmName("sdithaauelykgpxj")
public suspend fun provisionedUsers(vararg values: GetGroupProvisionedUsersProvisionedUser) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.provisionedUsers = mapped
}
/**
* @param value The search query to filter the provisioned users.
*/
@JvmName("wummlufbovnrscul")
public suspend fun search(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.search = mapped
}
/**
* @param value The username of the provisioned user.
*/
@JvmName("muyhswexecddtvgt")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.username = mapped
}
internal fun build(): GetGroupProvisionedUsersPlainArgs = GetGroupProvisionedUsersPlainArgs(
active = active,
blocked = blocked,
createdAfter = createdAfter,
createdBefore = createdBefore,
id = id ?: throw PulumiNullFieldException("id"),
provisionedUsers = provisionedUsers,
search = search,
username = username,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy