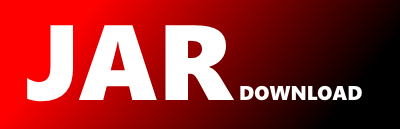
com.pulumi.gitlab.kotlin.inputs.GetGroupProvisionedUsersProvisionedUser.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin.inputs
import com.pulumi.gitlab.inputs.GetGroupProvisionedUsersProvisionedUser.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property avatarUrl The avatar URL of the provisioned user.
* @property bio The bio of the provisioned user.
* @property bot Whether the provisioned user is a bot.
* @property confirmedAt The confirmation date of the provisioned user.
* @property createdAt The creation date of the provisioned user.
* @property email The email of the provisioned user.
* @property external Whether the provisioned user is external.
* @property id The ID of the provisioned user.
* @property jobTitle The job title of the provisioned user.
* @property lastActivityOn The last activity date of the provisioned user.
* @property lastSignInAt The last sign-in date of the provisioned user.
* @property linkedin The LinkedIn ID of the provisioned user.
* @property location The location of the provisioned user.
* @property name The name of the provisioned user.
* @property organization The organization of the provisioned user.
* @property privateProfile Whether the provisioned user has a private profile.
* @property pronouns The pronouns of the provisioned user.
* @property publicEmail The public email of the provisioned user.
* @property skype The Skype ID of the provisioned user.
* @property state The state of the provisioned user.
* @property twitter The Twitter ID of the provisioned user.
* @property twoFactorEnabled Whether two-factor authentication is enabled for the provisioned user.
* @property username The username of the provisioned user.
* @property webUrl The web URL of the provisioned user.
* @property websiteUrl The website URL of the provisioned user.
*/
public data class GetGroupProvisionedUsersProvisionedUser(
public val avatarUrl: String,
public val bio: String,
public val bot: Boolean,
public val confirmedAt: String,
public val createdAt: String,
public val email: String,
public val `external`: Boolean,
public val id: String,
public val jobTitle: String,
public val lastActivityOn: String,
public val lastSignInAt: String,
public val linkedin: String,
public val location: String,
public val name: String,
public val organization: String,
public val privateProfile: Boolean,
public val pronouns: String,
public val publicEmail: String,
public val skype: String,
public val state: String,
public val twitter: String,
public val twoFactorEnabled: Boolean,
public val username: String,
public val webUrl: String,
public val websiteUrl: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.inputs.GetGroupProvisionedUsersProvisionedUser =
com.pulumi.gitlab.inputs.GetGroupProvisionedUsersProvisionedUser.builder()
.avatarUrl(avatarUrl.let({ args0 -> args0 }))
.bio(bio.let({ args0 -> args0 }))
.bot(bot.let({ args0 -> args0 }))
.confirmedAt(confirmedAt.let({ args0 -> args0 }))
.createdAt(createdAt.let({ args0 -> args0 }))
.email(email.let({ args0 -> args0 }))
.`external`(`external`.let({ args0 -> args0 }))
.id(id.let({ args0 -> args0 }))
.jobTitle(jobTitle.let({ args0 -> args0 }))
.lastActivityOn(lastActivityOn.let({ args0 -> args0 }))
.lastSignInAt(lastSignInAt.let({ args0 -> args0 }))
.linkedin(linkedin.let({ args0 -> args0 }))
.location(location.let({ args0 -> args0 }))
.name(name.let({ args0 -> args0 }))
.organization(organization.let({ args0 -> args0 }))
.privateProfile(privateProfile.let({ args0 -> args0 }))
.pronouns(pronouns.let({ args0 -> args0 }))
.publicEmail(publicEmail.let({ args0 -> args0 }))
.skype(skype.let({ args0 -> args0 }))
.state(state.let({ args0 -> args0 }))
.twitter(twitter.let({ args0 -> args0 }))
.twoFactorEnabled(twoFactorEnabled.let({ args0 -> args0 }))
.username(username.let({ args0 -> args0 }))
.webUrl(webUrl.let({ args0 -> args0 }))
.websiteUrl(websiteUrl.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetGroupProvisionedUsersProvisionedUser].
*/
@PulumiTagMarker
public class GetGroupProvisionedUsersProvisionedUserBuilder internal constructor() {
private var avatarUrl: String? = null
private var bio: String? = null
private var bot: Boolean? = null
private var confirmedAt: String? = null
private var createdAt: String? = null
private var email: String? = null
private var `external`: Boolean? = null
private var id: String? = null
private var jobTitle: String? = null
private var lastActivityOn: String? = null
private var lastSignInAt: String? = null
private var linkedin: String? = null
private var location: String? = null
private var name: String? = null
private var organization: String? = null
private var privateProfile: Boolean? = null
private var pronouns: String? = null
private var publicEmail: String? = null
private var skype: String? = null
private var state: String? = null
private var twitter: String? = null
private var twoFactorEnabled: Boolean? = null
private var username: String? = null
private var webUrl: String? = null
private var websiteUrl: String? = null
/**
* @param value The avatar URL of the provisioned user.
*/
@JvmName("nytpcspkwautvlny")
public suspend fun avatarUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.avatarUrl = mapped
}
/**
* @param value The bio of the provisioned user.
*/
@JvmName("yinhripkjjlahpge")
public suspend fun bio(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.bio = mapped
}
/**
* @param value Whether the provisioned user is a bot.
*/
@JvmName("snteajyiymnrveyx")
public suspend fun bot(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.bot = mapped
}
/**
* @param value The confirmation date of the provisioned user.
*/
@JvmName("lfukuybkrhcbcanv")
public suspend fun confirmedAt(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.confirmedAt = mapped
}
/**
* @param value The creation date of the provisioned user.
*/
@JvmName("gbeoejujdsoudejn")
public suspend fun createdAt(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.createdAt = mapped
}
/**
* @param value The email of the provisioned user.
*/
@JvmName("dfbhwawitglatxqt")
public suspend fun email(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.email = mapped
}
/**
* @param value Whether the provisioned user is external.
*/
@JvmName("xbdwrlfhgauihilq")
public suspend fun `external`(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.`external` = mapped
}
/**
* @param value The ID of the provisioned user.
*/
@JvmName("yvyjobcmoyrmguiv")
public suspend fun id(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.id = mapped
}
/**
* @param value The job title of the provisioned user.
*/
@JvmName("lmewtwbrnksnckis")
public suspend fun jobTitle(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.jobTitle = mapped
}
/**
* @param value The last activity date of the provisioned user.
*/
@JvmName("vocasyqiwcmfmwqm")
public suspend fun lastActivityOn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.lastActivityOn = mapped
}
/**
* @param value The last sign-in date of the provisioned user.
*/
@JvmName("audrqvdxhclhpqtu")
public suspend fun lastSignInAt(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.lastSignInAt = mapped
}
/**
* @param value The LinkedIn ID of the provisioned user.
*/
@JvmName("esrrroumwdqyjacv")
public suspend fun linkedin(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.linkedin = mapped
}
/**
* @param value The location of the provisioned user.
*/
@JvmName("ynqssnihvaofgumv")
public suspend fun location(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.location = mapped
}
/**
* @param value The name of the provisioned user.
*/
@JvmName("oheiexmoatkciaqq")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.name = mapped
}
/**
* @param value The organization of the provisioned user.
*/
@JvmName("jjfxwwwguqshfmbd")
public suspend fun organization(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.organization = mapped
}
/**
* @param value Whether the provisioned user has a private profile.
*/
@JvmName("apjyeutejrdqoiyp")
public suspend fun privateProfile(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.privateProfile = mapped
}
/**
* @param value The pronouns of the provisioned user.
*/
@JvmName("bypcapdhloeqadne")
public suspend fun pronouns(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.pronouns = mapped
}
/**
* @param value The public email of the provisioned user.
*/
@JvmName("ykgajujtjfdjfnwx")
public suspend fun publicEmail(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.publicEmail = mapped
}
/**
* @param value The Skype ID of the provisioned user.
*/
@JvmName("vghkvtxaqqipgtai")
public suspend fun skype(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.skype = mapped
}
/**
* @param value The state of the provisioned user.
*/
@JvmName("wbyrdikgibintjld")
public suspend fun state(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.state = mapped
}
/**
* @param value The Twitter ID of the provisioned user.
*/
@JvmName("invsoihpiwjdhtjn")
public suspend fun twitter(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.twitter = mapped
}
/**
* @param value Whether two-factor authentication is enabled for the provisioned user.
*/
@JvmName("yskqjxlpmmbgadpi")
public suspend fun twoFactorEnabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.twoFactorEnabled = mapped
}
/**
* @param value The username of the provisioned user.
*/
@JvmName("vwddcptwdbwjgtcv")
public suspend fun username(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.username = mapped
}
/**
* @param value The web URL of the provisioned user.
*/
@JvmName("dmatsfptdqvfmxyb")
public suspend fun webUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.webUrl = mapped
}
/**
* @param value The website URL of the provisioned user.
*/
@JvmName("jnobksmfktvtxlfj")
public suspend fun websiteUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.websiteUrl = mapped
}
internal fun build(): GetGroupProvisionedUsersProvisionedUser =
GetGroupProvisionedUsersProvisionedUser(
avatarUrl = avatarUrl ?: throw PulumiNullFieldException("avatarUrl"),
bio = bio ?: throw PulumiNullFieldException("bio"),
bot = bot ?: throw PulumiNullFieldException("bot"),
confirmedAt = confirmedAt ?: throw PulumiNullFieldException("confirmedAt"),
createdAt = createdAt ?: throw PulumiNullFieldException("createdAt"),
email = email ?: throw PulumiNullFieldException("email"),
`external` = `external` ?: throw PulumiNullFieldException("external"),
id = id ?: throw PulumiNullFieldException("id"),
jobTitle = jobTitle ?: throw PulumiNullFieldException("jobTitle"),
lastActivityOn = lastActivityOn ?: throw PulumiNullFieldException("lastActivityOn"),
lastSignInAt = lastSignInAt ?: throw PulumiNullFieldException("lastSignInAt"),
linkedin = linkedin ?: throw PulumiNullFieldException("linkedin"),
location = location ?: throw PulumiNullFieldException("location"),
name = name ?: throw PulumiNullFieldException("name"),
organization = organization ?: throw PulumiNullFieldException("organization"),
privateProfile = privateProfile ?: throw PulumiNullFieldException("privateProfile"),
pronouns = pronouns ?: throw PulumiNullFieldException("pronouns"),
publicEmail = publicEmail ?: throw PulumiNullFieldException("publicEmail"),
skype = skype ?: throw PulumiNullFieldException("skype"),
state = state ?: throw PulumiNullFieldException("state"),
twitter = twitter ?: throw PulumiNullFieldException("twitter"),
twoFactorEnabled = twoFactorEnabled ?: throw PulumiNullFieldException("twoFactorEnabled"),
username = username ?: throw PulumiNullFieldException("username"),
webUrl = webUrl ?: throw PulumiNullFieldException("webUrl"),
websiteUrl = websiteUrl ?: throw PulumiNullFieldException("websiteUrl"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy