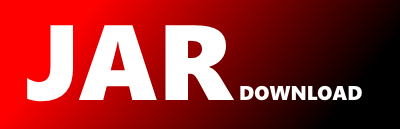
com.pulumi.googlenative.accesscontextmanager.v1.kotlin.AccessPolicyIamBinding.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.accesscontextmanager.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.iam.v1.kotlin.outputs.Condition
import com.pulumi.googlenative.iam.v1.kotlin.outputs.Condition.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AccessPolicyIamBinding].
*/
@PulumiTagMarker
public class AccessPolicyIamBindingResourceBuilder internal constructor() {
public var name: String? = null
public var args: AccessPolicyIamBindingArgs = AccessPolicyIamBindingArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AccessPolicyIamBindingArgsBuilder.() -> Unit) {
val builder = AccessPolicyIamBindingArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AccessPolicyIamBinding {
val builtJavaResource =
com.pulumi.googlenative.accesscontextmanager.v1.AccessPolicyIamBinding(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AccessPolicyIamBinding(builtJavaResource)
}
}
/**
* Sets the IAM policy for the specified Access Context Manager access policy. This method replaces the existing IAM policy on the access policy. The IAM policy controls the set of users who can perform specific operations on the Access Context Manager access policy.
*/
public class AccessPolicyIamBinding internal constructor(
override val javaResource: com.pulumi.googlenative.accesscontextmanager.v1.AccessPolicyIamBinding,
) : KotlinCustomResource(javaResource, AccessPolicyIamBindingMapper) {
/**
* An IAM Condition for a given binding. See https://cloud.google.com/iam/docs/conditions-overview for additional details.
*/
public val condition: Output?
get() = javaResource.condition().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
}).orElse(null)
})
/**
* The etag of the resource's IAM policy.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* Specifies the principals requesting access for a Google Cloud resource. `members` can have the following values: * `allUsers`: A special identifier that represents anyone who is on the internet; with or without a Google account. * `allAuthenticatedUsers`: A special identifier that represents anyone who is authenticated with a Google account or a service account. Does not include identities that come from external identity providers (IdPs) through identity federation. * `user:{emailid}`: An email address that represents a specific Google account. For example, `[email protected]` . * `serviceAccount:{emailid}`: An email address that represents a Google service account. For example, `[email protected]`. * `serviceAccount:{projectid}.svc.id.goog[{namespace}/{kubernetes-sa}]`: An identifier for a [Kubernetes service account](https://cloud.google.com/kubernetes-engine/docs/how-to/kubernetes-service-accounts). For example, `my-project.svc.id.goog[my-namespace/my-kubernetes-sa]`. * `group:{emailid}`: An email address that represents a Google group. For example, `[email protected]`. * `domain:{domain}`: The G Suite domain (primary) that represents all the users of that domain. For example, `google.com` or `example.com`. * `deleted:user:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a user that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the user is recovered, this value reverts to `user:{emailid}` and the recovered user retains the role in the binding. * `deleted:serviceAccount:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a service account that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the service account is undeleted, this value reverts to `serviceAccount:{emailid}` and the undeleted service account retains the role in the binding. * `deleted:group:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a Google group that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the group is recovered, this value reverts to `group:{emailid}` and the recovered group retains the role in the binding.
*/
public val members: Output>
get() = javaResource.members().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The name of the resource to manage IAM policies for.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The project in which the resource belongs. If it is not provided, a default will be supplied.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Role that is assigned to the list of `members`, or principals. For example, `roles/viewer`, `roles/editor`, or `roles/owner`.
*/
public val role: Output
get() = javaResource.role().applyValue({ args0 -> args0 })
}
public object AccessPolicyIamBindingMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.accesscontextmanager.v1.AccessPolicyIamBinding::class == javaResource::class
override fun map(javaResource: Resource): AccessPolicyIamBinding =
AccessPolicyIamBinding(
javaResource as
com.pulumi.googlenative.accesscontextmanager.v1.AccessPolicyIamBinding,
)
}
/**
* @see [AccessPolicyIamBinding].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AccessPolicyIamBinding].
*/
public suspend fun accessPolicyIamBinding(
name: String,
block: suspend AccessPolicyIamBindingResourceBuilder.() -> Unit,
): AccessPolicyIamBinding {
val builder = AccessPolicyIamBindingResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AccessPolicyIamBinding].
* @param name The _unique_ name of the resulting resource.
*/
public fun accessPolicyIamBinding(name: String): AccessPolicyIamBinding {
val builder = AccessPolicyIamBindingResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy