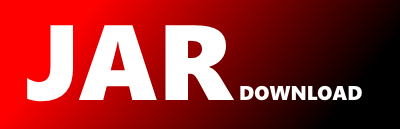
com.pulumi.googlenative.apigateway.v1.kotlin.inputs.ApigatewayApiConfigGrpcServiceDefinitionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.apigateway.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.apigateway.v1.inputs.ApigatewayApiConfigGrpcServiceDefinitionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A gRPC service definition.
* @property fileDescriptorSet Input only. File descriptor set, generated by protoc. To generate, use protoc with imports and source info included. For an example test.proto file, the following command would put the value in a new file named out.pb. $ protoc --include_imports --include_source_info test.proto -o out.pb
* @property source Optional. Uncompiled proto files associated with the descriptor set, used for display purposes (server-side compilation is not supported). These should match the inputs to 'protoc' command used to generate file_descriptor_set.
*/
public data class ApigatewayApiConfigGrpcServiceDefinitionArgs(
public val fileDescriptorSet: Output? = null,
public val source: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.apigateway.v1.inputs.ApigatewayApiConfigGrpcServiceDefinitionArgs =
com.pulumi.googlenative.apigateway.v1.inputs.ApigatewayApiConfigGrpcServiceDefinitionArgs.builder()
.fileDescriptorSet(fileDescriptorSet?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.source(
source?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ApigatewayApiConfigGrpcServiceDefinitionArgs].
*/
@PulumiTagMarker
public class ApigatewayApiConfigGrpcServiceDefinitionArgsBuilder internal constructor() {
private var fileDescriptorSet: Output? = null
private var source: Output>? = null
/**
* @param value Input only. File descriptor set, generated by protoc. To generate, use protoc with imports and source info included. For an example test.proto file, the following command would put the value in a new file named out.pb. $ protoc --include_imports --include_source_info test.proto -o out.pb
*/
@JvmName("gvbidmhhetycxmkm")
public suspend fun fileDescriptorSet(`value`: Output) {
this.fileDescriptorSet = value
}
/**
* @param value Optional. Uncompiled proto files associated with the descriptor set, used for display purposes (server-side compilation is not supported). These should match the inputs to 'protoc' command used to generate file_descriptor_set.
*/
@JvmName("xqwnpnvpxxxaqmrj")
public suspend fun source(`value`: Output>) {
this.source = value
}
@JvmName("ilykjugitvelutsa")
public suspend fun source(vararg values: Output) {
this.source = Output.all(values.asList())
}
/**
* @param values Optional. Uncompiled proto files associated with the descriptor set, used for display purposes (server-side compilation is not supported). These should match the inputs to 'protoc' command used to generate file_descriptor_set.
*/
@JvmName("dbonmeniavewdhwj")
public suspend fun source(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy