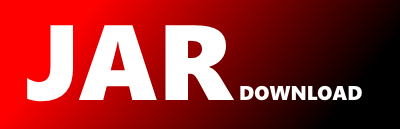
com.pulumi.googlenative.apigee.v1.kotlin.Alias.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.apigee.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.apigee.v1.kotlin.outputs.GoogleCloudApigeeV1CertificateResponse
import com.pulumi.googlenative.apigee.v1.kotlin.outputs.GoogleCloudApigeeV1CertificateResponse.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Alias].
*/
@PulumiTagMarker
public class AliasResourceBuilder internal constructor() {
public var name: String? = null
public var args: AliasArgs = AliasArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AliasArgsBuilder.() -> Unit) {
val builder = AliasArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Alias {
val builtJavaResource = com.pulumi.googlenative.apigee.v1.Alias(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Alias(builtJavaResource)
}
}
/**
* Creates an alias from a key/certificate pair. The structure of the request is controlled by the `format` query parameter: - `keycertfile` - Separate PEM-encoded key and certificate files are uploaded. Set `Content-Type: multipart/form-data` and include the `keyFile`, `certFile`, and `password` (if keys are encrypted) fields in the request body. If uploading to a truststore, omit `keyFile`. - `pkcs12` - A PKCS12 file is uploaded. Set `Content-Type: multipart/form-data`, provide the file in the `file` field, and include the `password` field if the file is encrypted in the request body. - `selfsignedcert` - A new private key and certificate are generated. Set `Content-Type: application/json` and include CertificateGenerationSpec in the request body.
* Auto-naming is currently not supported for this resource.
*/
public class Alias internal constructor(
override val javaResource: com.pulumi.googlenative.apigee.v1.Alias,
) : KotlinCustomResource(javaResource, AliasMapper) {
/**
* Alias for the key/certificate pair. Values must match the regular expression `[\w\s-.]{1,255}`. This must be provided for all formats except `selfsignedcert`; self-signed certs may specify the alias in either this parameter or the JSON body.
*/
public val alias: Output
get() = javaResource.alias().applyValue({ args0 -> args0 })
/**
* Chain of certificates under this alias.
*/
public val certsInfo: Output
get() = javaResource.certsInfo().applyValue({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
public val environmentId: Output
get() = javaResource.environmentId().applyValue({ args0 -> args0 })
/**
* Required. Format of the data. Valid values include: `selfsignedcert`, `keycertfile`, or `pkcs12`
*/
public val format: Output
get() = javaResource.format().applyValue({ args0 -> args0 })
/**
* Flag that specifies whether to ignore expiry validation. If set to `true`, no expiry validation will be performed.
*/
public val ignoreExpiryValidation: Output?
get() = javaResource.ignoreExpiryValidation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag that specifies whether to ignore newline validation. If set to `true`, no error is thrown when the file contains a certificate chain with no newline between each certificate. Defaults to `false`.
*/
public val ignoreNewlineValidation: Output?
get() = javaResource.ignoreNewlineValidation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val keystoreId: Output
get() = javaResource.keystoreId().applyValue({ args0 -> args0 })
public val organizationId: Output
get() = javaResource.organizationId().applyValue({ args0 -> args0 })
/**
* DEPRECATED: For improved security, specify the password in the request body instead of using the query parameter. To specify the password in the request body, set `Content-type: multipart/form-data` part with name `password`. Password for the private key file, if required.
*/
public val password: Output?
get() = javaResource.password().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Type of alias.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object AliasMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.apigee.v1.Alias::class == javaResource::class
override fun map(javaResource: Resource): Alias = Alias(
javaResource as
com.pulumi.googlenative.apigee.v1.Alias,
)
}
/**
* @see [Alias].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Alias].
*/
public suspend fun alias(name: String, block: suspend AliasResourceBuilder.() -> Unit): Alias {
val builder = AliasResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Alias].
* @param name The _unique_ name of the resulting resource.
*/
public fun alias(name: String): Alias {
val builder = AliasResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy