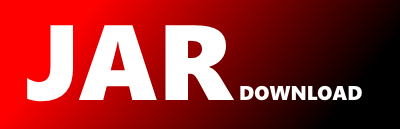
com.pulumi.googlenative.apigee.v1.kotlin.DebugSessionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.apigee.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.apigee.v1.DebugSessionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a debug session for a deployed API Proxy revision.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property apiId
* @property count Optional. The number of request to be traced. Min = 1, Max = 15, Default = 10.
* @property environmentId
* @property filter Optional. A conditional statement which is evaluated against the request message to determine if it should be traced. Syntax matches that of on API Proxy bundle flow Condition.
* @property name A unique ID for this DebugSession.
* @property organizationId
* @property revisionId
* @property timeout Optional. The time in seconds after which this DebugSession should end. This value will override the value in query param, if both are provided.
* @property tracesize Optional. The maximum number of bytes captured from the response payload. Min = 0, Max = 5120, Default = 5120.
* @property validity Optional. The length of time, in seconds, that this debug session is valid, starting from when it's received in the control plane. Min = 1, Max = 15, Default = 10.
*/
public data class DebugSessionArgs(
public val apiId: Output? = null,
public val count: Output? = null,
public val environmentId: Output? = null,
public val filter: Output? = null,
public val name: Output? = null,
public val organizationId: Output? = null,
public val revisionId: Output? = null,
public val timeout: Output? = null,
public val tracesize: Output? = null,
public val validity: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.apigee.v1.DebugSessionArgs =
com.pulumi.googlenative.apigee.v1.DebugSessionArgs.builder()
.apiId(apiId?.applyValue({ args0 -> args0 }))
.count(count?.applyValue({ args0 -> args0 }))
.environmentId(environmentId?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.organizationId(organizationId?.applyValue({ args0 -> args0 }))
.revisionId(revisionId?.applyValue({ args0 -> args0 }))
.timeout(timeout?.applyValue({ args0 -> args0 }))
.tracesize(tracesize?.applyValue({ args0 -> args0 }))
.validity(validity?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DebugSessionArgs].
*/
@PulumiTagMarker
public class DebugSessionArgsBuilder internal constructor() {
private var apiId: Output? = null
private var count: Output? = null
private var environmentId: Output? = null
private var filter: Output? = null
private var name: Output? = null
private var organizationId: Output? = null
private var revisionId: Output? = null
private var timeout: Output? = null
private var tracesize: Output? = null
private var validity: Output? = null
/**
* @param value
*/
@JvmName("eiswrsyithcekpxh")
public suspend fun apiId(`value`: Output) {
this.apiId = value
}
/**
* @param value Optional. The number of request to be traced. Min = 1, Max = 15, Default = 10.
*/
@JvmName("xfetysssdmgqcymu")
public suspend fun count(`value`: Output) {
this.count = value
}
/**
* @param value
*/
@JvmName("vmdjrmbhguxnetox")
public suspend fun environmentId(`value`: Output) {
this.environmentId = value
}
/**
* @param value Optional. A conditional statement which is evaluated against the request message to determine if it should be traced. Syntax matches that of on API Proxy bundle flow Condition.
*/
@JvmName("arjqejkpplpkhwlp")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value A unique ID for this DebugSession.
*/
@JvmName("ydgwwshgfpmrpgwd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("diigqewquftiwabb")
public suspend fun organizationId(`value`: Output) {
this.organizationId = value
}
/**
* @param value
*/
@JvmName("fryaewkjoadnxbin")
public suspend fun revisionId(`value`: Output) {
this.revisionId = value
}
/**
* @param value Optional. The time in seconds after which this DebugSession should end. This value will override the value in query param, if both are provided.
*/
@JvmName("sgrlholcswchlvcd")
public suspend fun timeout(`value`: Output) {
this.timeout = value
}
/**
* @param value Optional. The maximum number of bytes captured from the response payload. Min = 0, Max = 5120, Default = 5120.
*/
@JvmName("mogooaqymujjiwha")
public suspend fun tracesize(`value`: Output) {
this.tracesize = value
}
/**
* @param value Optional. The length of time, in seconds, that this debug session is valid, starting from when it's received in the control plane. Min = 1, Max = 15, Default = 10.
*/
@JvmName("snrkvcirkowupktg")
public suspend fun validity(`value`: Output) {
this.validity = value
}
/**
* @param value
*/
@JvmName("dbjxrdswhgaihmkd")
public suspend fun apiId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiId = mapped
}
/**
* @param value Optional. The number of request to be traced. Min = 1, Max = 15, Default = 10.
*/
@JvmName("cvnrfwbkhqyyrrrv")
public suspend fun count(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.count = mapped
}
/**
* @param value
*/
@JvmName("qailbmucrlxdqjox")
public suspend fun environmentId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environmentId = mapped
}
/**
* @param value Optional. A conditional statement which is evaluated against the request message to determine if it should be traced. Syntax matches that of on API Proxy bundle flow Condition.
*/
@JvmName("oknnxroktqfcjhlm")
public suspend fun filter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filter = mapped
}
/**
* @param value A unique ID for this DebugSession.
*/
@JvmName("cfhreqcjomrujkos")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("rgptrdqtxkyqtrxo")
public suspend fun organizationId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.organizationId = mapped
}
/**
* @param value
*/
@JvmName("scxhnskwfqasgmim")
public suspend fun revisionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.revisionId = mapped
}
/**
* @param value Optional. The time in seconds after which this DebugSession should end. This value will override the value in query param, if both are provided.
*/
@JvmName("rqgrjndesmothimr")
public suspend fun timeout(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeout = mapped
}
/**
* @param value Optional. The maximum number of bytes captured from the response payload. Min = 0, Max = 5120, Default = 5120.
*/
@JvmName("yuhdkpeacdvwvivj")
public suspend fun tracesize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tracesize = mapped
}
/**
* @param value Optional. The length of time, in seconds, that this debug session is valid, starting from when it's received in the control plane. Min = 1, Max = 15, Default = 10.
*/
@JvmName("vwhfudqbjrvnfpxi")
public suspend fun validity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validity = mapped
}
internal fun build(): DebugSessionArgs = DebugSessionArgs(
apiId = apiId,
count = count,
environmentId = environmentId,
filter = filter,
name = name,
organizationId = organizationId,
revisionId = revisionId,
timeout = timeout,
tracesize = tracesize,
validity = validity,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy