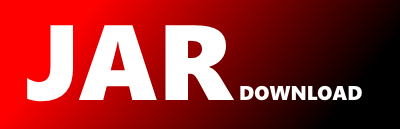
com.pulumi.googlenative.apigee.v1.kotlin.SharedflowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.apigee.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.apigee.v1.SharedflowArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Uploads a ZIP-formatted shared flow configuration bundle to an organization. If the shared flow already exists, this creates a new revision of it. If the shared flow does not exist, this creates it. Once imported, the shared flow revision must be deployed before it can be accessed at runtime. The size limit of a shared flow bundle is 15 MB.
* @property action Required. Must be set to either `import` or `validate`.
* @property contentType The HTTP Content-Type header value specifying the content type of the body.
* @property data The HTTP request/response body as raw binary.
* @property extensions Application specific response metadata. Must be set in the first response for streaming APIs.
* @property name Required. The name to give the shared flow
* @property organizationId
*/
public data class SharedflowArgs(
public val action: Output? = null,
public val contentType: Output? = null,
public val `data`: Output? = null,
public val extensions: Output>>? = null,
public val name: Output? = null,
public val organizationId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.apigee.v1.SharedflowArgs =
com.pulumi.googlenative.apigee.v1.SharedflowArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.contentType(contentType?.applyValue({ args0 -> args0 }))
.`data`(`data`?.applyValue({ args0 -> args0 }))
.extensions(
extensions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.key.to(args0.value)
}).toMap()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.organizationId(organizationId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SharedflowArgs].
*/
@PulumiTagMarker
public class SharedflowArgsBuilder internal constructor() {
private var action: Output? = null
private var contentType: Output? = null
private var `data`: Output? = null
private var extensions: Output>>? = null
private var name: Output? = null
private var organizationId: Output? = null
/**
* @param value Required. Must be set to either `import` or `validate`.
*/
@JvmName("qblicbkbdccusaah")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value The HTTP Content-Type header value specifying the content type of the body.
*/
@JvmName("jebcbsfcclrwdtbl")
public suspend fun contentType(`value`: Output) {
this.contentType = value
}
/**
* @param value The HTTP request/response body as raw binary.
*/
@JvmName("mhsnbyupikshpktv")
public suspend fun `data`(`value`: Output) {
this.`data` = value
}
/**
* @param value Application specific response metadata. Must be set in the first response for streaming APIs.
*/
@JvmName("dukglkgajoslqpfy")
public suspend fun extensions(`value`: Output>>) {
this.extensions = value
}
@JvmName("wedqooxmrfpgeujc")
public suspend fun extensions(vararg values: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy