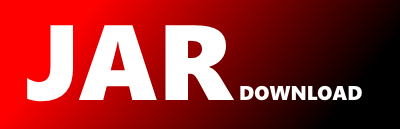
com.pulumi.googlenative.appengine.v1.kotlin.ApplicationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.appengine.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.appengine.v1.ApplicationArgs.builder
import com.pulumi.googlenative.appengine.v1.kotlin.enums.ApplicationDatabaseType
import com.pulumi.googlenative.appengine.v1.kotlin.enums.ApplicationServingStatus
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.FeatureSettingsArgs
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.FeatureSettingsArgsBuilder
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.IdentityAwareProxyArgs
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.IdentityAwareProxyArgsBuilder
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.UrlDispatchRuleArgs
import com.pulumi.googlenative.appengine.v1.kotlin.inputs.UrlDispatchRuleArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Creates an App Engine application for a Google Cloud Platform project. Required fields: id - The ID of the target Cloud Platform project. location - The region (https://cloud.google.com/appengine/docs/locations) where you want the App Engine application located.For more information about App Engine applications, see Managing Projects, Applications, and Billing (https://cloud.google.com/appengine/docs/standard/python/console/).
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property authDomain Google Apps authentication domain that controls which users can access this application.Defaults to open access for any Google Account.
* @property databaseType The type of the Cloud Firestore or Cloud Datastore database associated with this application.
* @property defaultCookieExpiration Cookie expiration policy for this application.
* @property dispatchRules HTTP path dispatch rules for requests to the application that do not explicitly target a service or version. Rules are order-dependent. Up to 20 dispatch rules can be supported.
* @property featureSettings The feature specific settings to be used in the application.
* @property iap
* @property id Identifier of the Application resource. This identifier is equivalent to the project ID of the Google Cloud Platform project where you want to deploy your application. Example: myapp.
* @property location Location from which this application runs. Application instances run out of the data centers in the specified location, which is also where all of the application's end user content is stored.Defaults to us-central.View the list of supported locations (https://cloud.google.com/appengine/docs/locations).
* @property project
* @property serviceAccount The service account associated with the application. This is the app-level default identity. If no identity provided during create version, Admin API will fallback to this one.
* @property servingStatus Serving status of this application.
*/
public data class ApplicationArgs(
public val authDomain: Output? = null,
public val databaseType: Output? = null,
public val defaultCookieExpiration: Output? = null,
public val dispatchRules: Output>? = null,
public val featureSettings: Output? = null,
public val iap: Output? = null,
public val id: Output? = null,
public val location: Output? = null,
public val project: Output? = null,
public val serviceAccount: Output? = null,
public val servingStatus: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.appengine.v1.ApplicationArgs =
com.pulumi.googlenative.appengine.v1.ApplicationArgs.builder()
.authDomain(authDomain?.applyValue({ args0 -> args0 }))
.databaseType(databaseType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.defaultCookieExpiration(defaultCookieExpiration?.applyValue({ args0 -> args0 }))
.dispatchRules(
dispatchRules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.featureSettings(featureSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.iap(iap?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.id(id?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.serviceAccount(serviceAccount?.applyValue({ args0 -> args0 }))
.servingStatus(servingStatus?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ApplicationArgs].
*/
@PulumiTagMarker
public class ApplicationArgsBuilder internal constructor() {
private var authDomain: Output? = null
private var databaseType: Output? = null
private var defaultCookieExpiration: Output? = null
private var dispatchRules: Output>? = null
private var featureSettings: Output? = null
private var iap: Output? = null
private var id: Output? = null
private var location: Output? = null
private var project: Output? = null
private var serviceAccount: Output? = null
private var servingStatus: Output? = null
/**
* @param value Google Apps authentication domain that controls which users can access this application.Defaults to open access for any Google Account.
*/
@JvmName("ulhkmjxfsxjvqtxg")
public suspend fun authDomain(`value`: Output) {
this.authDomain = value
}
/**
* @param value The type of the Cloud Firestore or Cloud Datastore database associated with this application.
*/
@JvmName("cawwqihbipcemash")
public suspend fun databaseType(`value`: Output) {
this.databaseType = value
}
/**
* @param value Cookie expiration policy for this application.
*/
@JvmName("goenblmyenpaknte")
public suspend fun defaultCookieExpiration(`value`: Output) {
this.defaultCookieExpiration = value
}
/**
* @param value HTTP path dispatch rules for requests to the application that do not explicitly target a service or version. Rules are order-dependent. Up to 20 dispatch rules can be supported.
*/
@JvmName("fxmpvaxamymefcuh")
public suspend fun dispatchRules(`value`: Output>) {
this.dispatchRules = value
}
@JvmName("becjkkquxfajuwga")
public suspend fun dispatchRules(vararg values: Output) {
this.dispatchRules = Output.all(values.asList())
}
/**
* @param values HTTP path dispatch rules for requests to the application that do not explicitly target a service or version. Rules are order-dependent. Up to 20 dispatch rules can be supported.
*/
@JvmName("mssplkkvgbqgwnrw")
public suspend fun dispatchRules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy