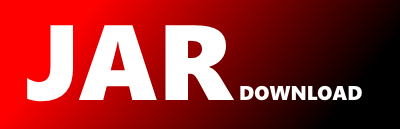
com.pulumi.googlenative.appengine.v1.kotlin.AuthorizedCertificate.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.appengine.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.appengine.v1.kotlin.outputs.CertificateRawDataResponse
import com.pulumi.googlenative.appengine.v1.kotlin.outputs.ManagedCertificateResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.appengine.v1.kotlin.outputs.CertificateRawDataResponse.Companion.toKotlin as certificateRawDataResponseToKotlin
import com.pulumi.googlenative.appengine.v1.kotlin.outputs.ManagedCertificateResponse.Companion.toKotlin as managedCertificateResponseToKotlin
/**
* Builder for [AuthorizedCertificate].
*/
@PulumiTagMarker
public class AuthorizedCertificateResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthorizedCertificateArgs = AuthorizedCertificateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthorizedCertificateArgsBuilder.() -> Unit) {
val builder = AuthorizedCertificateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthorizedCertificate {
val builtJavaResource =
com.pulumi.googlenative.appengine.v1.AuthorizedCertificate(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthorizedCertificate(builtJavaResource)
}
}
/**
* Uploads the specified SSL certificate.
* Auto-naming is currently not supported for this resource.
*/
public class AuthorizedCertificate internal constructor(
override val javaResource: com.pulumi.googlenative.appengine.v1.AuthorizedCertificate,
) : KotlinCustomResource(javaResource, AuthorizedCertificateMapper) {
public val appId: Output
get() = javaResource.appId().applyValue({ args0 -> args0 })
/**
* The SSL certificate serving the AuthorizedCertificate resource. This must be obtained independently from a certificate authority.
*/
public val certificateRawData: Output
get() = javaResource.certificateRawData().applyValue({ args0 ->
args0.let({ args0 ->
certificateRawDataResponseToKotlin(args0)
})
})
/**
* The user-specified display name of the certificate. This is not guaranteed to be unique. Example: My Certificate.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Aggregate count of the domain mappings with this certificate mapped. This count includes domain mappings on applications for which the user does not have VIEWER permissions.Only returned by GET or LIST requests when specifically requested by the view=FULL_CERTIFICATE option.
*/
public val domainMappingsCount: Output
get() = javaResource.domainMappingsCount().applyValue({ args0 -> args0 })
/**
* Topmost applicable domains of this certificate. This certificate applies to these domains and their subdomains. Example: example.com.
*/
public val domainNames: Output>
get() = javaResource.domainNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The time when this certificate expires. To update the renewal time on this certificate, upload an SSL certificate with a different expiration time using AuthorizedCertificates.UpdateAuthorizedCertificate.
*/
public val expireTime: Output
get() = javaResource.expireTime().applyValue({ args0 -> args0 })
/**
* Only applicable if this certificate is managed by App Engine. Managed certificates are tied to the lifecycle of a DomainMapping and cannot be updated or deleted via the AuthorizedCertificates API. If this certificate is manually administered by the user, this field will be empty.
*/
public val managedCertificate: Output
get() = javaResource.managedCertificate().applyValue({ args0 ->
args0.let({ args0 ->
managedCertificateResponseToKotlin(args0)
})
})
/**
* Full path to the AuthorizedCertificate resource in the API. Example: apps/myapp/authorizedCertificates/12345.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The full paths to user visible Domain Mapping resources that have this certificate mapped. Example: apps/myapp/domainMappings/example.com.This may not represent the full list of mapped domain mappings if the user does not have VIEWER permissions on all of the applications that have this certificate mapped. See domain_mappings_count for a complete count.Only returned by GET or LIST requests when specifically requested by the view=FULL_CERTIFICATE option.
*/
public val visibleDomainMappings: Output>
get() = javaResource.visibleDomainMappings().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
}
public object AuthorizedCertificateMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.appengine.v1.AuthorizedCertificate::class == javaResource::class
override fun map(javaResource: Resource): AuthorizedCertificate =
AuthorizedCertificate(
javaResource as
com.pulumi.googlenative.appengine.v1.AuthorizedCertificate,
)
}
/**
* @see [AuthorizedCertificate].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthorizedCertificate].
*/
public suspend fun authorizedCertificate(
name: String,
block: suspend AuthorizedCertificateResourceBuilder.() -> Unit,
): AuthorizedCertificate {
val builder = AuthorizedCertificateResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthorizedCertificate].
* @param name The _unique_ name of the resulting resource.
*/
public fun authorizedCertificate(name: String): AuthorizedCertificate {
val builder = AuthorizedCertificateResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy