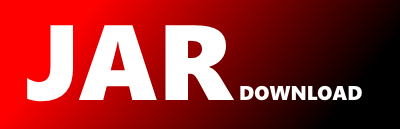
com.pulumi.googlenative.appengine.v1.kotlin.inputs.UrlMapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.appengine.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.appengine.v1.inputs.UrlMapArgs.builder
import com.pulumi.googlenative.appengine.v1.kotlin.enums.UrlMapAuthFailAction
import com.pulumi.googlenative.appengine.v1.kotlin.enums.UrlMapLogin
import com.pulumi.googlenative.appengine.v1.kotlin.enums.UrlMapRedirectHttpResponseCode
import com.pulumi.googlenative.appengine.v1.kotlin.enums.UrlMapSecurityLevel
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* URL pattern and description of how the URL should be handled. App Engine can handle URLs by executing application code or by serving static files uploaded with the version, such as images, CSS, or JavaScript.
* @property apiEndpoint Uses API Endpoints to handle requests.
* @property authFailAction Action to take when users access resources that require authentication. Defaults to redirect.
* @property login Level of login required to access this resource. Not supported for Node.js in the App Engine standard environment.
* @property redirectHttpResponseCode 30x code to use when performing redirects for the secure field. Defaults to 302.
* @property script Executes a script to handle the requests that match this URL pattern. Only the auto value is supported for Node.js in the App Engine standard environment, for example "script": "auto".
* @property securityLevel Security (HTTPS) enforcement for this URL.
* @property staticFiles Returns the contents of a file, such as an image, as the response.
* @property urlRegex URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings. All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
public data class UrlMapArgs(
public val apiEndpoint: Output? = null,
public val authFailAction: Output? = null,
public val login: Output? = null,
public val redirectHttpResponseCode: Output? = null,
public val script: Output? = null,
public val securityLevel: Output? = null,
public val staticFiles: Output? = null,
public val urlRegex: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.appengine.v1.inputs.UrlMapArgs =
com.pulumi.googlenative.appengine.v1.inputs.UrlMapArgs.builder()
.apiEndpoint(apiEndpoint?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.authFailAction(authFailAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.login(login?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.redirectHttpResponseCode(
redirectHttpResponseCode?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.script(script?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securityLevel(securityLevel?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.staticFiles(staticFiles?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.urlRegex(urlRegex?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UrlMapArgs].
*/
@PulumiTagMarker
public class UrlMapArgsBuilder internal constructor() {
private var apiEndpoint: Output? = null
private var authFailAction: Output? = null
private var login: Output? = null
private var redirectHttpResponseCode: Output? = null
private var script: Output? = null
private var securityLevel: Output? = null
private var staticFiles: Output? = null
private var urlRegex: Output? = null
/**
* @param value Uses API Endpoints to handle requests.
*/
@JvmName("lmxytobgdwcjscqy")
public suspend fun apiEndpoint(`value`: Output) {
this.apiEndpoint = value
}
/**
* @param value Action to take when users access resources that require authentication. Defaults to redirect.
*/
@JvmName("objhiibtsieuuyqt")
public suspend fun authFailAction(`value`: Output) {
this.authFailAction = value
}
/**
* @param value Level of login required to access this resource. Not supported for Node.js in the App Engine standard environment.
*/
@JvmName("xurilihfjrvdcygj")
public suspend fun login(`value`: Output) {
this.login = value
}
/**
* @param value 30x code to use when performing redirects for the secure field. Defaults to 302.
*/
@JvmName("ojaywcmrelgewfhu")
public suspend fun redirectHttpResponseCode(`value`: Output) {
this.redirectHttpResponseCode = value
}
/**
* @param value Executes a script to handle the requests that match this URL pattern. Only the auto value is supported for Node.js in the App Engine standard environment, for example "script": "auto".
*/
@JvmName("usgisymudmkefgqc")
public suspend fun script(`value`: Output) {
this.script = value
}
/**
* @param value Security (HTTPS) enforcement for this URL.
*/
@JvmName("kbsapitrwammjkuc")
public suspend fun securityLevel(`value`: Output) {
this.securityLevel = value
}
/**
* @param value Returns the contents of a file, such as an image, as the response.
*/
@JvmName("qbxenaljccuowpcd")
public suspend fun staticFiles(`value`: Output) {
this.staticFiles = value
}
/**
* @param value URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings. All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
@JvmName("diuldifgciwtvqux")
public suspend fun urlRegex(`value`: Output) {
this.urlRegex = value
}
/**
* @param value Uses API Endpoints to handle requests.
*/
@JvmName("rgfnkoffpuyedrqu")
public suspend fun apiEndpoint(`value`: ApiEndpointHandlerArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiEndpoint = mapped
}
/**
* @param argument Uses API Endpoints to handle requests.
*/
@JvmName("itmlillhmqtgrsma")
public suspend fun apiEndpoint(argument: suspend ApiEndpointHandlerArgsBuilder.() -> Unit) {
val toBeMapped = ApiEndpointHandlerArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.apiEndpoint = mapped
}
/**
* @param value Action to take when users access resources that require authentication. Defaults to redirect.
*/
@JvmName("hporfkvothljuons")
public suspend fun authFailAction(`value`: UrlMapAuthFailAction?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authFailAction = mapped
}
/**
* @param value Level of login required to access this resource. Not supported for Node.js in the App Engine standard environment.
*/
@JvmName("mkymxxdamdvfrjfu")
public suspend fun login(`value`: UrlMapLogin?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.login = mapped
}
/**
* @param value 30x code to use when performing redirects for the secure field. Defaults to 302.
*/
@JvmName("wpxnwmmpgakcgdoq")
public suspend fun redirectHttpResponseCode(`value`: UrlMapRedirectHttpResponseCode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redirectHttpResponseCode = mapped
}
/**
* @param value Executes a script to handle the requests that match this URL pattern. Only the auto value is supported for Node.js in the App Engine standard environment, for example "script": "auto".
*/
@JvmName("xkdrdpyxhrrsxmhl")
public suspend fun script(`value`: ScriptHandlerArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.script = mapped
}
/**
* @param argument Executes a script to handle the requests that match this URL pattern. Only the auto value is supported for Node.js in the App Engine standard environment, for example "script": "auto".
*/
@JvmName("xnoboxpcalnyjvie")
public suspend fun script(argument: suspend ScriptHandlerArgsBuilder.() -> Unit) {
val toBeMapped = ScriptHandlerArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.script = mapped
}
/**
* @param value Security (HTTPS) enforcement for this URL.
*/
@JvmName("igvllyqrakkpcagf")
public suspend fun securityLevel(`value`: UrlMapSecurityLevel?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityLevel = mapped
}
/**
* @param value Returns the contents of a file, such as an image, as the response.
*/
@JvmName("ypmaxldesrmvuqjw")
public suspend fun staticFiles(`value`: StaticFilesHandlerArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.staticFiles = mapped
}
/**
* @param argument Returns the contents of a file, such as an image, as the response.
*/
@JvmName("ryqlknqepjjhjicy")
public suspend fun staticFiles(argument: suspend StaticFilesHandlerArgsBuilder.() -> Unit) {
val toBeMapped = StaticFilesHandlerArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.staticFiles = mapped
}
/**
* @param value URL prefix. Uses regular expression syntax, which means regexp special characters must be escaped, but should not contain groupings. All URLs that begin with this prefix are handled by this handler, using the portion of the URL after the prefix as part of the file path.
*/
@JvmName("yatdsfuinufowicx")
public suspend fun urlRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.urlRegex = mapped
}
internal fun build(): UrlMapArgs = UrlMapArgs(
apiEndpoint = apiEndpoint,
authFailAction = authFailAction,
login = login,
redirectHttpResponseCode = redirectHttpResponseCode,
script = script,
securityLevel = securityLevel,
staticFiles = staticFiles,
urlRegex = urlRegex,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy