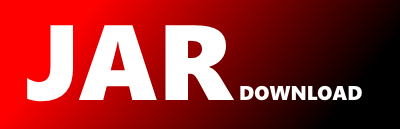
com.pulumi.googlenative.appengine.v1beta.kotlin.IngressRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.appengine.v1beta.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.appengine.v1beta.IngressRuleArgs.builder
import com.pulumi.googlenative.appengine.v1beta.kotlin.enums.IngressRuleAction
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates a firewall rule for the application.
* Auto-naming is currently not supported for this resource.
* @property action The action to take on matched requests.
* @property appId
* @property description An optional string description of this rule. This field has a maximum length of 400 characters.
* @property priority A positive integer between 1, Int32.MaxValue-1 that defines the order of rule evaluation. Rules with the lowest priority are evaluated first.A default rule at priority Int32.MaxValue matches all IPv4 and IPv6 traffic when no previous rule matches. Only the action of this rule can be modified by the user.
* @property sourceRange IP address or range, defined using CIDR notation, of requests that this rule applies to. You can use the wildcard character "*" to match all IPs equivalent to "0/0" and "::/0" together. Examples: 192.168.1.1 or 192.168.0.0/16 or 2001:db8::/32 or 2001:0db8:0000:0042:0000:8a2e:0370:7334. Truncation will be silently performed on addresses which are not properly truncated. For example, 1.2.3.4/24 is accepted as the same address as 1.2.3.0/24. Similarly, for IPv6, 2001:db8::1/32 is accepted as the same address as 2001:db8::/32.
*/
public data class IngressRuleArgs(
public val action: Output? = null,
public val appId: Output? = null,
public val description: Output? = null,
public val priority: Output? = null,
public val sourceRange: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.appengine.v1beta.IngressRuleArgs =
com.pulumi.googlenative.appengine.v1beta.IngressRuleArgs.builder()
.action(action?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.appId(appId?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.priority(priority?.applyValue({ args0 -> args0 }))
.sourceRange(sourceRange?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [IngressRuleArgs].
*/
@PulumiTagMarker
public class IngressRuleArgsBuilder internal constructor() {
private var action: Output? = null
private var appId: Output? = null
private var description: Output? = null
private var priority: Output? = null
private var sourceRange: Output? = null
/**
* @param value The action to take on matched requests.
*/
@JvmName("jtmgfdexiuwucvco")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value
*/
@JvmName("knevboaqtkhysmyb")
public suspend fun appId(`value`: Output) {
this.appId = value
}
/**
* @param value An optional string description of this rule. This field has a maximum length of 400 characters.
*/
@JvmName("eswvftdwjlrmnamo")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A positive integer between 1, Int32.MaxValue-1 that defines the order of rule evaluation. Rules with the lowest priority are evaluated first.A default rule at priority Int32.MaxValue matches all IPv4 and IPv6 traffic when no previous rule matches. Only the action of this rule can be modified by the user.
*/
@JvmName("ubljbaqrhbicmogi")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value IP address or range, defined using CIDR notation, of requests that this rule applies to. You can use the wildcard character "*" to match all IPs equivalent to "0/0" and "::/0" together. Examples: 192.168.1.1 or 192.168.0.0/16 or 2001:db8::/32 or 2001:0db8:0000:0042:0000:8a2e:0370:7334. Truncation will be silently performed on addresses which are not properly truncated. For example, 1.2.3.4/24 is accepted as the same address as 1.2.3.0/24. Similarly, for IPv6, 2001:db8::1/32 is accepted as the same address as 2001:db8::/32.
*/
@JvmName("xwgwuoifsrwbthxi")
public suspend fun sourceRange(`value`: Output) {
this.sourceRange = value
}
/**
* @param value The action to take on matched requests.
*/
@JvmName("iesjbfufghivdwsv")
public suspend fun action(`value`: IngressRuleAction?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value
*/
@JvmName("xxpcohpalswsspyf")
public suspend fun appId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.appId = mapped
}
/**
* @param value An optional string description of this rule. This field has a maximum length of 400 characters.
*/
@JvmName("nskqwkblmgfuhmxx")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value A positive integer between 1, Int32.MaxValue-1 that defines the order of rule evaluation. Rules with the lowest priority are evaluated first.A default rule at priority Int32.MaxValue matches all IPv4 and IPv6 traffic when no previous rule matches. Only the action of this rule can be modified by the user.
*/
@JvmName("cyonyhwauwkrhkdj")
public suspend fun priority(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.priority = mapped
}
/**
* @param value IP address or range, defined using CIDR notation, of requests that this rule applies to. You can use the wildcard character "*" to match all IPs equivalent to "0/0" and "::/0" together. Examples: 192.168.1.1 or 192.168.0.0/16 or 2001:db8::/32 or 2001:0db8:0000:0042:0000:8a2e:0370:7334. Truncation will be silently performed on addresses which are not properly truncated. For example, 1.2.3.4/24 is accepted as the same address as 1.2.3.0/24. Similarly, for IPv6, 2001:db8::1/32 is accepted as the same address as 2001:db8::/32.
*/
@JvmName("gtluiqlnikeajljk")
public suspend fun sourceRange(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRange = mapped
}
internal fun build(): IngressRuleArgs = IngressRuleArgs(
action = action,
appId = appId,
description = description,
priority = priority,
sourceRange = sourceRange,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy