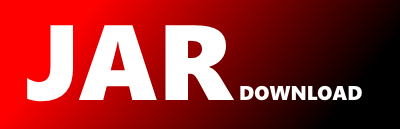
com.pulumi.googlenative.bigquery.v2.kotlin.Bigquery_v2Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.bigquery.v2.kotlin
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getDatasetPlain
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getJobPlain
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getRoutinePlain
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getRowAccessPolicyIamPolicyPlain
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getTableIamPolicyPlain
import com.pulumi.googlenative.bigquery.v2.Bigquery_v2Functions.getTablePlain
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetDatasetPlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetDatasetPlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetJobPlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetJobPlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRoutinePlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRoutinePlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRowAccessPolicyIamPolicyPlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRowAccessPolicyIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTableIamPolicyPlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTableIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTablePlainArgs
import com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTablePlainArgsBuilder
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetDatasetResult
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetJobResult
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetRoutineResult
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetRowAccessPolicyIamPolicyResult
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetTableIamPolicyResult
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetTableResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetDatasetResult.Companion.toKotlin as getDatasetResultToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetJobResult.Companion.toKotlin as getJobResultToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetRoutineResult.Companion.toKotlin as getRoutineResultToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetRowAccessPolicyIamPolicyResult.Companion.toKotlin as getRowAccessPolicyIamPolicyResultToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetTableIamPolicyResult.Companion.toKotlin as getTableIamPolicyResultToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.GetTableResult.Companion.toKotlin as getTableResultToKotlin
public object Bigquery_v2Functions {
/**
* Returns the dataset specified by datasetID.
* @param argument null
* @return null
*/
public suspend fun getDataset(argument: GetDatasetPlainArgs): GetDatasetResult =
getDatasetResultToKotlin(getDatasetPlain(argument.toJava()).await())
/**
* @see [getDataset].
* @param datasetId
* @param project
* @return null
*/
public suspend fun getDataset(datasetId: String, project: String? = null): GetDatasetResult {
val argument = GetDatasetPlainArgs(
datasetId = datasetId,
project = project,
)
return getDatasetResultToKotlin(getDatasetPlain(argument.toJava()).await())
}
/**
* @see [getDataset].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetDatasetPlainArgs].
* @return null
*/
public suspend fun getDataset(argument: suspend GetDatasetPlainArgsBuilder.() -> Unit): GetDatasetResult {
val builder = GetDatasetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDatasetResultToKotlin(getDatasetPlain(builtArgument.toJava()).await())
}
/**
* Returns information about a specific job. Job information is available for a six month period after creation. Requires that you're the person who ran the job, or have the Is Owner project role.
* @param argument null
* @return null
*/
public suspend fun getJob(argument: GetJobPlainArgs): GetJobResult =
getJobResultToKotlin(getJobPlain(argument.toJava()).await())
/**
* @see [getJob].
* @param jobId
* @param location
* @param project
* @return null
*/
public suspend fun getJob(
jobId: String,
location: String? = null,
project: String? = null,
): GetJobResult {
val argument = GetJobPlainArgs(
jobId = jobId,
location = location,
project = project,
)
return getJobResultToKotlin(getJobPlain(argument.toJava()).await())
}
/**
* @see [getJob].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetJobPlainArgs].
* @return null
*/
public suspend fun getJob(argument: suspend GetJobPlainArgsBuilder.() -> Unit): GetJobResult {
val builder = GetJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getJobResultToKotlin(getJobPlain(builtArgument.toJava()).await())
}
/**
* Gets the specified routine resource by routine ID.
* @param argument null
* @return null
*/
public suspend fun getRoutine(argument: GetRoutinePlainArgs): GetRoutineResult =
getRoutineResultToKotlin(getRoutinePlain(argument.toJava()).await())
/**
* @see [getRoutine].
* @param datasetId
* @param project
* @param readMask
* @param routineId
* @return null
*/
public suspend fun getRoutine(
datasetId: String,
project: String? = null,
readMask: String? = null,
routineId: String,
): GetRoutineResult {
val argument = GetRoutinePlainArgs(
datasetId = datasetId,
project = project,
readMask = readMask,
routineId = routineId,
)
return getRoutineResultToKotlin(getRoutinePlain(argument.toJava()).await())
}
/**
* @see [getRoutine].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRoutinePlainArgs].
* @return null
*/
public suspend fun getRoutine(argument: suspend GetRoutinePlainArgsBuilder.() -> Unit): GetRoutineResult {
val builder = GetRoutinePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRoutineResultToKotlin(getRoutinePlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getRowAccessPolicyIamPolicy(argument: GetRowAccessPolicyIamPolicyPlainArgs): GetRowAccessPolicyIamPolicyResult =
getRowAccessPolicyIamPolicyResultToKotlin(getRowAccessPolicyIamPolicyPlain(argument.toJava()).await())
/**
* @see [getRowAccessPolicyIamPolicy].
* @param datasetId
* @param project
* @param rowAccessPolicyId
* @param tableId
* @return null
*/
public suspend fun getRowAccessPolicyIamPolicy(
datasetId: String,
project: String? = null,
rowAccessPolicyId: String,
tableId: String,
): GetRowAccessPolicyIamPolicyResult {
val argument = GetRowAccessPolicyIamPolicyPlainArgs(
datasetId = datasetId,
project = project,
rowAccessPolicyId = rowAccessPolicyId,
tableId = tableId,
)
return getRowAccessPolicyIamPolicyResultToKotlin(getRowAccessPolicyIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getRowAccessPolicyIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetRowAccessPolicyIamPolicyPlainArgs].
* @return null
*/
public suspend fun getRowAccessPolicyIamPolicy(argument: suspend GetRowAccessPolicyIamPolicyPlainArgsBuilder.() -> Unit): GetRowAccessPolicyIamPolicyResult {
val builder = GetRowAccessPolicyIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRowAccessPolicyIamPolicyResultToKotlin(getRowAccessPolicyIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets the specified table resource by table ID. This method does not return the data in the table, it only returns the table resource, which describes the structure of this table.
* @param argument null
* @return null
*/
public suspend fun getTable(argument: GetTablePlainArgs): GetTableResult =
getTableResultToKotlin(getTablePlain(argument.toJava()).await())
/**
* @see [getTable].
* @param datasetId
* @param project
* @param selectedFields
* @param tableId
* @param view
* @return null
*/
public suspend fun getTable(
datasetId: String,
project: String? = null,
selectedFields: String? = null,
tableId: String,
view: String? = null,
): GetTableResult {
val argument = GetTablePlainArgs(
datasetId = datasetId,
project = project,
selectedFields = selectedFields,
tableId = tableId,
view = view,
)
return getTableResultToKotlin(getTablePlain(argument.toJava()).await())
}
/**
* @see [getTable].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTablePlainArgs].
* @return null
*/
public suspend fun getTable(argument: suspend GetTablePlainArgsBuilder.() -> Unit): GetTableResult {
val builder = GetTablePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTableResultToKotlin(getTablePlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getTableIamPolicy(argument: GetTableIamPolicyPlainArgs): GetTableIamPolicyResult =
getTableIamPolicyResultToKotlin(getTableIamPolicyPlain(argument.toJava()).await())
/**
* @see [getTableIamPolicy].
* @param datasetId
* @param project
* @param tableId
* @return null
*/
public suspend fun getTableIamPolicy(
datasetId: String,
project: String? = null,
tableId: String,
): GetTableIamPolicyResult {
val argument = GetTableIamPolicyPlainArgs(
datasetId = datasetId,
project = project,
tableId = tableId,
)
return getTableIamPolicyResultToKotlin(getTableIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getTableIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.bigquery.v2.kotlin.inputs.GetTableIamPolicyPlainArgs].
* @return null
*/
public suspend fun getTableIamPolicy(argument: suspend GetTableIamPolicyPlainArgsBuilder.() -> Unit): GetTableIamPolicyResult {
val builder = GetTableIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTableIamPolicyResultToKotlin(getTableIamPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy