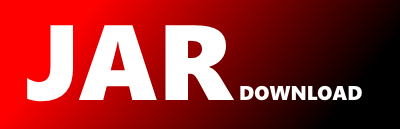
com.pulumi.googlenative.bigquery.v2.kotlin.Routine.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.bigquery.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ArgumentResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RemoteFunctionOptionsResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RoutineReferenceResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.SparkOptionsResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StandardSqlDataTypeResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StandardSqlTableTypeResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ArgumentResponse.Companion.toKotlin as argumentResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RemoteFunctionOptionsResponse.Companion.toKotlin as remoteFunctionOptionsResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RoutineReferenceResponse.Companion.toKotlin as routineReferenceResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.SparkOptionsResponse.Companion.toKotlin as sparkOptionsResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StandardSqlDataTypeResponse.Companion.toKotlin as standardSqlDataTypeResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StandardSqlTableTypeResponse.Companion.toKotlin as standardSqlTableTypeResponseToKotlin
/**
* Builder for [Routine].
*/
@PulumiTagMarker
public class RoutineResourceBuilder internal constructor() {
public var name: String? = null
public var args: RoutineArgs = RoutineArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RoutineArgsBuilder.() -> Unit) {
val builder = RoutineArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Routine {
val builtJavaResource = com.pulumi.googlenative.bigquery.v2.Routine(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Routine(builtJavaResource)
}
}
/**
* Creates a new routine in the dataset.
* Auto-naming is currently not supported for this resource.
*/
public class Routine internal constructor(
override val javaResource: com.pulumi.googlenative.bigquery.v2.Routine,
) : KotlinCustomResource(javaResource, RoutineMapper) {
/**
* Optional.
*/
public val arguments: Output>
get() = javaResource.arguments().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
argumentResponseToKotlin(args0)
})
})
})
/**
* The time when this routine was created, in milliseconds since the epoch.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
public val datasetId: Output
get() = javaResource.datasetId().applyValue({ args0 -> args0 })
/**
* The body of the routine. For functions, this is the expression in the AS clause. If language=SQL, it is the substring inside (but excluding) the parentheses. For example, for the function created with the following statement: `CREATE FUNCTION JoinLines(x string, y string) as (concat(x, "\n", y))` The definition_body is `concat(x, "\n", y)` (\n is not replaced with linebreak). If language=JAVASCRIPT, it is the evaluated string in the AS clause. For example, for the function created with the following statement: `CREATE FUNCTION f() RETURNS STRING LANGUAGE js AS 'return "\n";\n'` The definition_body is `return "\n";\n` Note that both \n are replaced with linebreaks.
*/
public val definitionBody: Output
get() = javaResource.definitionBody().applyValue({ args0 -> args0 })
/**
* Optional. The description of the routine, if defined.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Optional. The determinism level of the JavaScript UDF, if defined.
*/
public val determinismLevel: Output
get() = javaResource.determinismLevel().applyValue({ args0 -> args0 })
/**
* A hash of this resource.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* Optional. If language = "JAVASCRIPT", this field stores the path of the imported JAVASCRIPT libraries.
*/
public val importedLibraries: Output>
get() = javaResource.importedLibraries().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Optional. Defaults to "SQL" if remote_function_options field is absent, not set otherwise.
*/
public val language: Output
get() = javaResource.language().applyValue({ args0 -> args0 })
/**
* The time when this routine was last modified, in milliseconds since the epoch.
*/
public val lastModifiedTime: Output
get() = javaResource.lastModifiedTime().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Optional. Remote function specific options.
*/
public val remoteFunctionOptions: Output
get() = javaResource.remoteFunctionOptions().applyValue({ args0 ->
args0.let({ args0 ->
remoteFunctionOptionsResponseToKotlin(args0)
})
})
/**
* Optional. Can be set only if routine_type = "TABLE_VALUED_FUNCTION". If absent, the return table type is inferred from definition_body at query time in each query that references this routine. If present, then the columns in the evaluated table result will be cast to match the column types specified in return table type, at query time.
*/
public val returnTableType: Output
get() = javaResource.returnTableType().applyValue({ args0 ->
args0.let({ args0 ->
standardSqlTableTypeResponseToKotlin(args0)
})
})
/**
* Optional if language = "SQL"; required otherwise. Cannot be set if routine_type = "TABLE_VALUED_FUNCTION". If absent, the return type is inferred from definition_body at query time in each query that references this routine. If present, then the evaluated result will be cast to the specified returned type at query time. For example, for the functions created with the following statements: * `CREATE FUNCTION Add(x FLOAT64, y FLOAT64) RETURNS FLOAT64 AS (x + y);` * `CREATE FUNCTION Increment(x FLOAT64) AS (Add(x, 1));` * `CREATE FUNCTION Decrement(x FLOAT64) RETURNS FLOAT64 AS (Add(x, -1));` The return_type is `{type_kind: "FLOAT64"}` for `Add` and `Decrement`, and is absent for `Increment` (inferred as FLOAT64 at query time). Suppose the function `Add` is replaced by `CREATE OR REPLACE FUNCTION Add(x INT64, y INT64) AS (x + y);` Then the inferred return type of `Increment` is automatically changed to INT64 at query time, while the return type of `Decrement` remains FLOAT64.
*/
public val returnType: Output
get() = javaResource.returnType().applyValue({ args0 ->
args0.let({ args0 ->
standardSqlDataTypeResponseToKotlin(args0)
})
})
/**
* Reference describing the ID of this routine.
*/
public val routineReference: Output
get() = javaResource.routineReference().applyValue({ args0 ->
args0.let({ args0 ->
routineReferenceResponseToKotlin(args0)
})
})
/**
* The type of routine.
*/
public val routineType: Output
get() = javaResource.routineType().applyValue({ args0 -> args0 })
/**
* Optional. Spark specific options.
*/
public val sparkOptions: Output
get() = javaResource.sparkOptions().applyValue({ args0 ->
args0.let({ args0 ->
sparkOptionsResponseToKotlin(args0)
})
})
/**
* Optional. Can be set for procedures only. If true (default), the definition body will be validated in the creation and the updates of the procedure. For procedures with an argument of ANY TYPE, the definition body validtion is not supported at creation/update time, and thus this field must be set to false explicitly.
*/
public val strictMode: Output
get() = javaResource.strictMode().applyValue({ args0 -> args0 })
}
public object RoutineMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.bigquery.v2.Routine::class == javaResource::class
override fun map(javaResource: Resource): Routine = Routine(
javaResource as
com.pulumi.googlenative.bigquery.v2.Routine,
)
}
/**
* @see [Routine].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Routine].
*/
public suspend fun routine(name: String, block: suspend RoutineResourceBuilder.() -> Unit): Routine {
val builder = RoutineResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Routine].
* @param name The _unique_ name of the resulting resource.
*/
public fun routine(name: String): Routine {
val builder = RoutineResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy