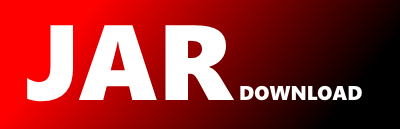
com.pulumi.googlenative.bigquery.v2.kotlin.Table.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.bigquery.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.CloneDefinitionResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ClusteringResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.EncryptionConfigurationResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ExternalDataConfigurationResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.MaterializedViewDefinitionResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ModelDefinitionResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RangePartitioningResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.SnapshotDefinitionResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StreamingbufferResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableConstraintsResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableReferenceResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableSchemaResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TimePartitioningResponse
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ViewDefinitionResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.CloneDefinitionResponse.Companion.toKotlin as cloneDefinitionResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ClusteringResponse.Companion.toKotlin as clusteringResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.EncryptionConfigurationResponse.Companion.toKotlin as encryptionConfigurationResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ExternalDataConfigurationResponse.Companion.toKotlin as externalDataConfigurationResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.MaterializedViewDefinitionResponse.Companion.toKotlin as materializedViewDefinitionResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ModelDefinitionResponse.Companion.toKotlin as modelDefinitionResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.RangePartitioningResponse.Companion.toKotlin as rangePartitioningResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.SnapshotDefinitionResponse.Companion.toKotlin as snapshotDefinitionResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.StreamingbufferResponse.Companion.toKotlin as streamingbufferResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableConstraintsResponse.Companion.toKotlin as tableConstraintsResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableReferenceResponse.Companion.toKotlin as tableReferenceResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TableSchemaResponse.Companion.toKotlin as tableSchemaResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.TimePartitioningResponse.Companion.toKotlin as timePartitioningResponseToKotlin
import com.pulumi.googlenative.bigquery.v2.kotlin.outputs.ViewDefinitionResponse.Companion.toKotlin as viewDefinitionResponseToKotlin
/**
* Builder for [Table].
*/
@PulumiTagMarker
public class TableResourceBuilder internal constructor() {
public var name: String? = null
public var args: TableArgs = TableArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TableArgsBuilder.() -> Unit) {
val builder = TableArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Table {
val builtJavaResource = com.pulumi.googlenative.bigquery.v2.Table(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Table(builtJavaResource)
}
}
/**
* Creates a new, empty table in the dataset.
* Auto-naming is currently not supported for this resource.
*/
public class Table internal constructor(
override val javaResource: com.pulumi.googlenative.bigquery.v2.Table,
) : KotlinCustomResource(javaResource, TableMapper) {
/**
* Clone definition.
*/
public val cloneDefinition: Output
get() = javaResource.cloneDefinition().applyValue({ args0 ->
args0.let({ args0 ->
cloneDefinitionResponseToKotlin(args0)
})
})
/**
* [Beta] Clustering specification for the table. Must be specified with partitioning, data in the table will be first partitioned and subsequently clustered.
*/
public val clustering: Output
get() = javaResource.clustering().applyValue({ args0 ->
args0.let({ args0 ->
clusteringResponseToKotlin(args0)
})
})
/**
* The time when this table was created, in milliseconds since the epoch.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
public val datasetId: Output
get() = javaResource.datasetId().applyValue({ args0 -> args0 })
/**
* The default collation of the table.
*/
public val defaultCollation: Output
get() = javaResource.defaultCollation().applyValue({ args0 -> args0 })
/**
* The default rounding mode of the table.
*/
public val defaultRoundingMode: Output
get() = javaResource.defaultRoundingMode().applyValue({ args0 -> args0 })
/**
* [Optional] A user-friendly description of this table.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Custom encryption configuration (e.g., Cloud KMS keys).
*/
public val encryptionConfiguration: Output
get() = javaResource.encryptionConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
encryptionConfigurationResponseToKotlin(args0)
})
})
/**
* A hash of the table metadata. Used to ensure there were no concurrent modifications to the resource when attempting an update. Not guaranteed to change when the table contents or the fields numRows, numBytes, numLongTermBytes or lastModifiedTime change.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* [Optional] The time when this table expires, in milliseconds since the epoch. If not present, the table will persist indefinitely. Expired tables will be deleted and their storage reclaimed. The defaultTableExpirationMs property of the encapsulating dataset can be used to set a default expirationTime on newly created tables.
*/
public val expirationTime: Output
get() = javaResource.expirationTime().applyValue({ args0 -> args0 })
/**
* [Optional] Describes the data format, location, and other properties of a table stored outside of BigQuery. By defining these properties, the data source can then be queried as if it were a standard BigQuery table.
*/
public val externalDataConfiguration: Output
get() = javaResource.externalDataConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
externalDataConfigurationResponseToKotlin(args0)
})
})
/**
* [Optional] A descriptive name for this table.
*/
public val friendlyName: Output
get() = javaResource.friendlyName().applyValue({ args0 -> args0 })
/**
* The type of the resource.
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
/**
* The labels associated with this table. You can use these to organize and group your tables. Label keys and values can be no longer than 63 characters, can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. Label values are optional. Label keys must start with a letter and each label in the list must have a different key.
*/
public val labels: Output