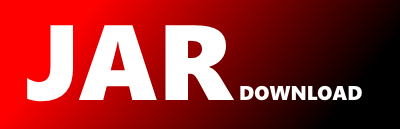
com.pulumi.googlenative.bigquery.v2.kotlin.inputs.ArgumentArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.bigquery.v2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.bigquery.v2.inputs.ArgumentArgs.builder
import com.pulumi.googlenative.bigquery.v2.kotlin.enums.ArgumentArgumentKind
import com.pulumi.googlenative.bigquery.v2.kotlin.enums.ArgumentMode
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Input/output argument of a function or a stored procedure.
* @property argumentKind Optional. Defaults to FIXED_TYPE.
* @property dataType Required unless argument_kind = ANY_TYPE.
* @property mode Optional. Specifies whether the argument is input or output. Can be set for procedures only.
* @property name Optional. The name of this argument. Can be absent for function return argument.
*/
public data class ArgumentArgs(
public val argumentKind: Output? = null,
public val dataType: Output? = null,
public val mode: Output? = null,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.bigquery.v2.inputs.ArgumentArgs =
com.pulumi.googlenative.bigquery.v2.inputs.ArgumentArgs.builder()
.argumentKind(argumentKind?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dataType(dataType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.mode(mode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ArgumentArgs].
*/
@PulumiTagMarker
public class ArgumentArgsBuilder internal constructor() {
private var argumentKind: Output? = null
private var dataType: Output? = null
private var mode: Output? = null
private var name: Output? = null
/**
* @param value Optional. Defaults to FIXED_TYPE.
*/
@JvmName("hyuhqqdwhrqlpipb")
public suspend fun argumentKind(`value`: Output) {
this.argumentKind = value
}
/**
* @param value Required unless argument_kind = ANY_TYPE.
*/
@JvmName("qmjbfrcmdpbalgyn")
public suspend fun dataType(`value`: Output) {
this.dataType = value
}
/**
* @param value Optional. Specifies whether the argument is input or output. Can be set for procedures only.
*/
@JvmName("uwiqvqxrxwbhpjed")
public suspend fun mode(`value`: Output) {
this.mode = value
}
/**
* @param value Optional. The name of this argument. Can be absent for function return argument.
*/
@JvmName("mdkxbiyjkxtmprse")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Optional. Defaults to FIXED_TYPE.
*/
@JvmName("vgdcpfhkhifuorit")
public suspend fun argumentKind(`value`: ArgumentArgumentKind?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.argumentKind = mapped
}
/**
* @param value Required unless argument_kind = ANY_TYPE.
*/
@JvmName("ljvpoajfuuylanek")
public suspend fun dataType(`value`: StandardSqlDataTypeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataType = mapped
}
/**
* @param argument Required unless argument_kind = ANY_TYPE.
*/
@JvmName("xjxieycnficuydeo")
public suspend fun dataType(argument: suspend StandardSqlDataTypeArgsBuilder.() -> Unit) {
val toBeMapped = StandardSqlDataTypeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.dataType = mapped
}
/**
* @param value Optional. Specifies whether the argument is input or output. Can be set for procedures only.
*/
@JvmName("jprqtjiibsbvkoii")
public suspend fun mode(`value`: ArgumentMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mode = mapped
}
/**
* @param value Optional. The name of this argument. Can be absent for function return argument.
*/
@JvmName("okptnkpewpbqhrml")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): ArgumentArgs = ArgumentArgs(
argumentKind = argumentKind,
dataType = dataType,
mode = mode,
name = name,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy