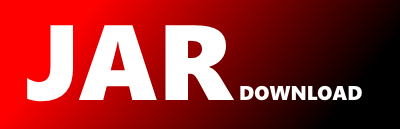
com.pulumi.googlenative.cloudasset.v1.kotlin.Feed.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudasset.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.cloudasset.v1.kotlin.outputs.ExprResponse
import com.pulumi.googlenative.cloudasset.v1.kotlin.outputs.FeedOutputConfigResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.cloudasset.v1.kotlin.outputs.ExprResponse.Companion.toKotlin as exprResponseToKotlin
import com.pulumi.googlenative.cloudasset.v1.kotlin.outputs.FeedOutputConfigResponse.Companion.toKotlin as feedOutputConfigResponseToKotlin
/**
* Builder for [Feed].
*/
@PulumiTagMarker
public class FeedResourceBuilder internal constructor() {
public var name: String? = null
public var args: FeedArgs = FeedArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FeedArgsBuilder.() -> Unit) {
val builder = FeedArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Feed {
val builtJavaResource = com.pulumi.googlenative.cloudasset.v1.Feed(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Feed(builtJavaResource)
}
}
/**
* Creates a feed in a parent project/folder/organization to listen to its asset updates.
* Auto-naming is currently not supported for this resource.
*/
public class Feed internal constructor(
override val javaResource: com.pulumi.googlenative.cloudasset.v1.Feed,
) : KotlinCustomResource(javaResource, FeedMapper) {
/**
* A list of the full names of the assets to receive updates. You must specify either or both of asset_names and asset_types. Only asset updates matching specified asset_names or asset_types are exported to the feed. Example: `//compute.googleapis.com/projects/my_project_123/zones/zone1/instances/instance1`. For a list of the full names for supported asset types, see [Resource name format](/asset-inventory/docs/resource-name-format).
*/
public val assetNames: Output>
get() = javaResource.assetNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* A list of types of the assets to receive updates. You must specify either or both of asset_names and asset_types. Only asset updates matching specified asset_names or asset_types are exported to the feed. Example: `"compute.googleapis.com/Disk"` For a list of all supported asset types, see [Supported asset types](/asset-inventory/docs/supported-asset-types).
*/
public val assetTypes: Output>
get() = javaResource.assetTypes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* A condition which determines whether an asset update should be published. If specified, an asset will be returned only when the expression evaluates to true. When set, `expression` field in the `Expr` must be a valid [CEL expression] (https://github.com/google/cel-spec) on a TemporalAsset with name `temporal_asset`. Example: a Feed with expression ("temporal_asset.deleted == true") will only publish Asset deletions. Other fields of `Expr` are optional. See our [user guide](https://cloud.google.com/asset-inventory/docs/monitoring-asset-changes-with-condition) for detailed instructions.
*/
public val condition: Output
get() = javaResource.condition().applyValue({ args0 ->
args0.let({ args0 ->
exprResponseToKotlin(args0)
})
})
/**
* Asset content type. If not specified, no content but the asset name and type will be returned.
*/
public val contentType: Output
get() = javaResource.contentType().applyValue({ args0 -> args0 })
/**
* Feed output configuration defining where the asset updates are published to.
*/
public val feedOutputConfig: Output
get() = javaResource.feedOutputConfig().applyValue({ args0 ->
args0.let({ args0 ->
feedOutputConfigResponseToKotlin(args0)
})
})
/**
* The format will be projects/{project_number}/feeds/{client-assigned_feed_identifier} or folders/{folder_number}/feeds/{client-assigned_feed_identifier} or organizations/{organization_number}/feeds/{client-assigned_feed_identifier} The client-assigned feed identifier must be unique within the parent project/folder/organization.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A list of relationship types to output, for example: `INSTANCE_TO_INSTANCEGROUP`. This field should only be specified if content_type=RELATIONSHIP. * If specified: it outputs specified relationship updates on the [asset_names] or the [asset_types]. It returns an error if any of the [relationship_types] doesn't belong to the supported relationship types of the [asset_names] or [asset_types], or any of the [asset_names] or the [asset_types] doesn't belong to the source types of the [relationship_types]. * Otherwise: it outputs the supported relationships of the types of [asset_names] and [asset_types] or returns an error if any of the [asset_names] or the [asset_types] has no replationship support. See [Introduction to Cloud Asset Inventory](https://cloud.google.com/asset-inventory/docs/overview) for all supported asset types and relationship types.
*/
public val relationshipTypes: Output>
get() = javaResource.relationshipTypes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
public val v1Id: Output
get() = javaResource.v1Id().applyValue({ args0 -> args0 })
public val v1Id1: Output
get() = javaResource.v1Id1().applyValue({ args0 -> args0 })
}
public object FeedMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.cloudasset.v1.Feed::class == javaResource::class
override fun map(javaResource: Resource): Feed = Feed(
javaResource as
com.pulumi.googlenative.cloudasset.v1.Feed,
)
}
/**
* @see [Feed].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Feed].
*/
public suspend fun feed(name: String, block: suspend FeedResourceBuilder.() -> Unit): Feed {
val builder = FeedResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Feed].
* @param name The _unique_ name of the resulting resource.
*/
public fun feed(name: String): Feed {
val builder = FeedResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy