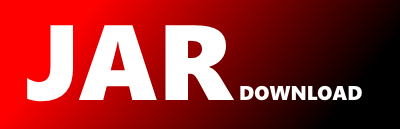
com.pulumi.googlenative.cloudbuild.v1.kotlin.GithubEnterpriseConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudbuild.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudbuild.v1.GithubEnterpriseConfigArgs.builder
import com.pulumi.googlenative.cloudbuild.v1.kotlin.inputs.GitHubEnterpriseSecretsArgs
import com.pulumi.googlenative.cloudbuild.v1.kotlin.inputs.GitHubEnterpriseSecretsArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Create an association between a GCP project and a GitHub Enterprise server.
* @property appId The GitHub app id of the Cloud Build app on the GitHub Enterprise server.
* @property displayName Name to display for this config.
* @property gheConfigId Optional. The ID to use for the GithubEnterpriseConfig, which will become the final component of the GithubEnterpriseConfig's resource name. ghe_config_id must meet the following requirements: + They must contain only alphanumeric characters and dashes. + They can be 1-64 characters long. + They must begin and end with an alphanumeric character
* @property hostUrl The URL of the github enterprise host the configuration is for.
* @property location
* @property name Optional. The full resource name for the GitHubEnterpriseConfig For example: "projects/{$project_id}/locations/{$location_id}/githubEnterpriseConfigs/{$config_id}"
* @property peeredNetwork Optional. The network to be used when reaching out to the GitHub Enterprise server. The VPC network must be enabled for private service connection. This should be set if the GitHub Enterprise server is hosted on-premises and not reachable by public internet. If this field is left empty, no network peering will occur and calls to the GitHub Enterprise server will be made over the public internet. Must be in the format `projects/{project}/global/networks/{network}`, where {project} is a project number or id and {network} is the name of a VPC network in the project.
* @property project
* @property projectId ID of the project.
* @property secrets Names of secrets in Secret Manager.
* @property sslCa Optional. SSL certificate to use for requests to GitHub Enterprise.
* @property webhookKey The key that should be attached to webhook calls to the ReceiveWebhook endpoint.
*/
public data class GithubEnterpriseConfigArgs(
public val appId: Output? = null,
public val displayName: Output? = null,
public val gheConfigId: Output? = null,
public val hostUrl: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val peeredNetwork: Output? = null,
public val project: Output? = null,
public val projectId: Output? = null,
public val secrets: Output? = null,
public val sslCa: Output? = null,
public val webhookKey: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudbuild.v1.GithubEnterpriseConfigArgs =
com.pulumi.googlenative.cloudbuild.v1.GithubEnterpriseConfigArgs.builder()
.appId(appId?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.gheConfigId(gheConfigId?.applyValue({ args0 -> args0 }))
.hostUrl(hostUrl?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.peeredNetwork(peeredNetwork?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.projectId(projectId?.applyValue({ args0 -> args0 }))
.secrets(secrets?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sslCa(sslCa?.applyValue({ args0 -> args0 }))
.webhookKey(webhookKey?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GithubEnterpriseConfigArgs].
*/
@PulumiTagMarker
public class GithubEnterpriseConfigArgsBuilder internal constructor() {
private var appId: Output? = null
private var displayName: Output? = null
private var gheConfigId: Output? = null
private var hostUrl: Output? = null
private var location: Output? = null
private var name: Output? = null
private var peeredNetwork: Output? = null
private var project: Output? = null
private var projectId: Output? = null
private var secrets: Output? = null
private var sslCa: Output? = null
private var webhookKey: Output? = null
/**
* @param value The GitHub app id of the Cloud Build app on the GitHub Enterprise server.
*/
@JvmName("erlnlykyqsesckfh")
public suspend fun appId(`value`: Output) {
this.appId = value
}
/**
* @param value Name to display for this config.
*/
@JvmName("bpexpcuaaffolvgo")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Optional. The ID to use for the GithubEnterpriseConfig, which will become the final component of the GithubEnterpriseConfig's resource name. ghe_config_id must meet the following requirements: + They must contain only alphanumeric characters and dashes. + They can be 1-64 characters long. + They must begin and end with an alphanumeric character
*/
@JvmName("vmdykewbgttivhbl")
public suspend fun gheConfigId(`value`: Output) {
this.gheConfigId = value
}
/**
* @param value The URL of the github enterprise host the configuration is for.
*/
@JvmName("cnowdyqxcahevvqh")
public suspend fun hostUrl(`value`: Output) {
this.hostUrl = value
}
/**
* @param value
*/
@JvmName("oubnrximawqhkwow")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Optional. The full resource name for the GitHubEnterpriseConfig For example: "projects/{$project_id}/locations/{$location_id}/githubEnterpriseConfigs/{$config_id}"
*/
@JvmName("ipdakvuomruspvfk")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Optional. The network to be used when reaching out to the GitHub Enterprise server. The VPC network must be enabled for private service connection. This should be set if the GitHub Enterprise server is hosted on-premises and not reachable by public internet. If this field is left empty, no network peering will occur and calls to the GitHub Enterprise server will be made over the public internet. Must be in the format `projects/{project}/global/networks/{network}`, where {project} is a project number or id and {network} is the name of a VPC network in the project.
*/
@JvmName("amhafdhkmgsreijw")
public suspend fun peeredNetwork(`value`: Output) {
this.peeredNetwork = value
}
/**
* @param value
*/
@JvmName("uqekakqjpfiydlhc")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value ID of the project.
*/
@JvmName("bfoylxprjorwibvx")
public suspend fun projectId(`value`: Output) {
this.projectId = value
}
/**
* @param value Names of secrets in Secret Manager.
*/
@JvmName("sketufboyvwlswod")
public suspend fun secrets(`value`: Output) {
this.secrets = value
}
/**
* @param value Optional. SSL certificate to use for requests to GitHub Enterprise.
*/
@JvmName("ubsyjsvnroyexbjw")
public suspend fun sslCa(`value`: Output) {
this.sslCa = value
}
/**
* @param value The key that should be attached to webhook calls to the ReceiveWebhook endpoint.
*/
@JvmName("erdtxuuvseoyootq")
public suspend fun webhookKey(`value`: Output) {
this.webhookKey = value
}
/**
* @param value The GitHub app id of the Cloud Build app on the GitHub Enterprise server.
*/
@JvmName("mxwaithoxgvtmlsi")
public suspend fun appId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.appId = mapped
}
/**
* @param value Name to display for this config.
*/
@JvmName("fhnpagwdprnbmrnm")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value Optional. The ID to use for the GithubEnterpriseConfig, which will become the final component of the GithubEnterpriseConfig's resource name. ghe_config_id must meet the following requirements: + They must contain only alphanumeric characters and dashes. + They can be 1-64 characters long. + They must begin and end with an alphanumeric character
*/
@JvmName("kcbkpferrdtipkjs")
public suspend fun gheConfigId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gheConfigId = mapped
}
/**
* @param value The URL of the github enterprise host the configuration is for.
*/
@JvmName("abilmfwsfofjdyqy")
public suspend fun hostUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostUrl = mapped
}
/**
* @param value
*/
@JvmName("yqolxgnmlpxubntd")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Optional. The full resource name for the GitHubEnterpriseConfig For example: "projects/{$project_id}/locations/{$location_id}/githubEnterpriseConfigs/{$config_id}"
*/
@JvmName("xcubpfelqofwoygk")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Optional. The network to be used when reaching out to the GitHub Enterprise server. The VPC network must be enabled for private service connection. This should be set if the GitHub Enterprise server is hosted on-premises and not reachable by public internet. If this field is left empty, no network peering will occur and calls to the GitHub Enterprise server will be made over the public internet. Must be in the format `projects/{project}/global/networks/{network}`, where {project} is a project number or id and {network} is the name of a VPC network in the project.
*/
@JvmName("fabipdadhaepmcwm")
public suspend fun peeredNetwork(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peeredNetwork = mapped
}
/**
* @param value
*/
@JvmName("fwmkfghtvwqdoctg")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value ID of the project.
*/
@JvmName("jwxvjfpfaobduoot")
public suspend fun projectId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.projectId = mapped
}
/**
* @param value Names of secrets in Secret Manager.
*/
@JvmName("otnpacvlftgnemdd")
public suspend fun secrets(`value`: GitHubEnterpriseSecretsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secrets = mapped
}
/**
* @param argument Names of secrets in Secret Manager.
*/
@JvmName("qyrdhtbpoxjmjjas")
public suspend fun secrets(argument: suspend GitHubEnterpriseSecretsArgsBuilder.() -> Unit) {
val toBeMapped = GitHubEnterpriseSecretsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secrets = mapped
}
/**
* @param value Optional. SSL certificate to use for requests to GitHub Enterprise.
*/
@JvmName("iatlmughldepicwl")
public suspend fun sslCa(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslCa = mapped
}
/**
* @param value The key that should be attached to webhook calls to the ReceiveWebhook endpoint.
*/
@JvmName("dlkjyusroljroygl")
public suspend fun webhookKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webhookKey = mapped
}
internal fun build(): GithubEnterpriseConfigArgs = GithubEnterpriseConfigArgs(
appId = appId,
displayName = displayName,
gheConfigId = gheConfigId,
hostUrl = hostUrl,
location = location,
name = name,
peeredNetwork = peeredNetwork,
project = project,
projectId = projectId,
secrets = secrets,
sslCa = sslCa,
webhookKey = webhookKey,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy