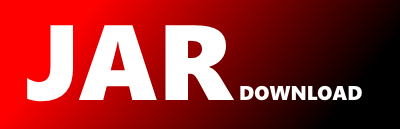
com.pulumi.googlenative.cloudbuild.v1.kotlin.inputs.SourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudbuild.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudbuild.v1.inputs.SourceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Location of the source in a supported storage service.
* @property gitSource If provided, get the source from this Git repository.
* @property repoSource If provided, get the source from this location in a Cloud Source Repository.
* @property storageSource If provided, get the source from this location in Google Cloud Storage.
* @property storageSourceManifest If provided, get the source from this manifest in Google Cloud Storage. This feature is in Preview; see description [here](https://github.com/GoogleCloudPlatform/cloud-builders/tree/master/gcs-fetcher).
*/
public data class SourceArgs(
public val gitSource: Output? = null,
public val repoSource: Output? = null,
public val storageSource: Output? = null,
public val storageSourceManifest: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudbuild.v1.inputs.SourceArgs =
com.pulumi.googlenative.cloudbuild.v1.inputs.SourceArgs.builder()
.gitSource(gitSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.repoSource(repoSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.storageSource(storageSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.storageSourceManifest(
storageSourceManifest?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [SourceArgs].
*/
@PulumiTagMarker
public class SourceArgsBuilder internal constructor() {
private var gitSource: Output? = null
private var repoSource: Output? = null
private var storageSource: Output? = null
private var storageSourceManifest: Output? = null
/**
* @param value If provided, get the source from this Git repository.
*/
@JvmName("akiqkojnlykehkcb")
public suspend fun gitSource(`value`: Output) {
this.gitSource = value
}
/**
* @param value If provided, get the source from this location in a Cloud Source Repository.
*/
@JvmName("klukadrdtidifkhe")
public suspend fun repoSource(`value`: Output) {
this.repoSource = value
}
/**
* @param value If provided, get the source from this location in Google Cloud Storage.
*/
@JvmName("fghbddaaoexafvcf")
public suspend fun storageSource(`value`: Output) {
this.storageSource = value
}
/**
* @param value If provided, get the source from this manifest in Google Cloud Storage. This feature is in Preview; see description [here](https://github.com/GoogleCloudPlatform/cloud-builders/tree/master/gcs-fetcher).
*/
@JvmName("yqtoaqcjhbscxtsc")
public suspend fun storageSourceManifest(`value`: Output) {
this.storageSourceManifest = value
}
/**
* @param value If provided, get the source from this Git repository.
*/
@JvmName("nttjfeaxopnrysac")
public suspend fun gitSource(`value`: GitSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gitSource = mapped
}
/**
* @param argument If provided, get the source from this Git repository.
*/
@JvmName("sugaqweyvitfqsym")
public suspend fun gitSource(argument: suspend GitSourceArgsBuilder.() -> Unit) {
val toBeMapped = GitSourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.gitSource = mapped
}
/**
* @param value If provided, get the source from this location in a Cloud Source Repository.
*/
@JvmName("qdwbxajmvigbbrax")
public suspend fun repoSource(`value`: RepoSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repoSource = mapped
}
/**
* @param argument If provided, get the source from this location in a Cloud Source Repository.
*/
@JvmName("nfcvodcrcjecbeat")
public suspend fun repoSource(argument: suspend RepoSourceArgsBuilder.() -> Unit) {
val toBeMapped = RepoSourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.repoSource = mapped
}
/**
* @param value If provided, get the source from this location in Google Cloud Storage.
*/
@JvmName("wnmujtgxlcpesnrw")
public suspend fun storageSource(`value`: StorageSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageSource = mapped
}
/**
* @param argument If provided, get the source from this location in Google Cloud Storage.
*/
@JvmName("gwnsxodmdskrjpkm")
public suspend fun storageSource(argument: suspend StorageSourceArgsBuilder.() -> Unit) {
val toBeMapped = StorageSourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.storageSource = mapped
}
/**
* @param value If provided, get the source from this manifest in Google Cloud Storage. This feature is in Preview; see description [here](https://github.com/GoogleCloudPlatform/cloud-builders/tree/master/gcs-fetcher).
*/
@JvmName("iaqbexgjbnnkubsb")
public suspend fun storageSourceManifest(`value`: StorageSourceManifestArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageSourceManifest = mapped
}
/**
* @param argument If provided, get the source from this manifest in Google Cloud Storage. This feature is in Preview; see description [here](https://github.com/GoogleCloudPlatform/cloud-builders/tree/master/gcs-fetcher).
*/
@JvmName("wojoxxvxfqsyrwxy")
public suspend fun storageSourceManifest(argument: suspend StorageSourceManifestArgsBuilder.() -> Unit) {
val toBeMapped = StorageSourceManifestArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.storageSourceManifest = mapped
}
internal fun build(): SourceArgs = SourceArgs(
gitSource = gitSource,
repoSource = repoSource,
storageSource = storageSource,
storageSourceManifest = storageSourceManifest,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy