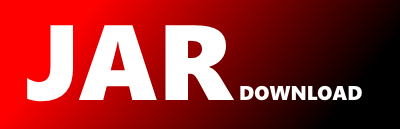
com.pulumi.googlenative.cloudchannel.v1.kotlin.CustomerRepricingConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudchannel.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudchannel.v1.CustomerRepricingConfigArgs.builder
import com.pulumi.googlenative.cloudchannel.v1.kotlin.inputs.GoogleCloudChannelV1RepricingConfigArgs
import com.pulumi.googlenative.cloudchannel.v1.kotlin.inputs.GoogleCloudChannelV1RepricingConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a CustomerRepricingConfig. Call this method to set modifications for a specific customer's bill. You can only create configs if the RepricingConfig.effective_invoice_month is a future month. If needed, you can create a config for the current month, with some restrictions. When creating a config for a future month, make sure there are no existing configs for that RepricingConfig.effective_invoice_month. The following restrictions are for creating configs in the current month. * This functionality is reserved for recovering from an erroneous config, and should not be used for regular business cases. * The new config will not modify exports used with other configs. Changes to the config may be immediate, but may take up to 24 hours. * There is a limit of ten configs for any RepricingConfig.EntitlementGranularity.entitlement or RepricingConfig.effective_invoice_month. * The contained CustomerRepricingConfig.repricing_config vaule must be different from the value used in the current config for a RepricingConfig.EntitlementGranularity.entitlement. Possible Error Codes: * PERMISSION_DENIED: If the account making the request and the account being queried are different. * INVALID_ARGUMENT: Missing or invalid required parameters in the request. Also displays if the updated config is for the current month or past months. * NOT_FOUND: The CustomerRepricingConfig specified does not exist or is not associated with the given account. * INTERNAL: Any non-user error related to technical issues in the backend. In this case, contact Cloud Channel support. Return Value: If successful, the updated CustomerRepricingConfig resource, otherwise returns an error.
* Auto-naming is currently not supported for this resource.
* @property accountId
* @property customerId
* @property repricingConfig The configuration for bill modifications made by a reseller before sending it to customers.
*/
public data class CustomerRepricingConfigArgs(
public val accountId: Output? = null,
public val customerId: Output? = null,
public val repricingConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudchannel.v1.CustomerRepricingConfigArgs =
com.pulumi.googlenative.cloudchannel.v1.CustomerRepricingConfigArgs.builder()
.accountId(accountId?.applyValue({ args0 -> args0 }))
.customerId(customerId?.applyValue({ args0 -> args0 }))
.repricingConfig(
repricingConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [CustomerRepricingConfigArgs].
*/
@PulumiTagMarker
public class CustomerRepricingConfigArgsBuilder internal constructor() {
private var accountId: Output? = null
private var customerId: Output? = null
private var repricingConfig: Output? = null
/**
* @param value
*/
@JvmName("alaihnixnmwlbvai")
public suspend fun accountId(`value`: Output) {
this.accountId = value
}
/**
* @param value
*/
@JvmName("vdxbkqvwqdlyssmk")
public suspend fun customerId(`value`: Output) {
this.customerId = value
}
/**
* @param value The configuration for bill modifications made by a reseller before sending it to customers.
*/
@JvmName("pafidwqkghwioejg")
public suspend fun repricingConfig(`value`: Output) {
this.repricingConfig = value
}
/**
* @param value
*/
@JvmName("vyddtvjuxkrejlsd")
public suspend fun accountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountId = mapped
}
/**
* @param value
*/
@JvmName("qmxwmlkacbeujumu")
public suspend fun customerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customerId = mapped
}
/**
* @param value The configuration for bill modifications made by a reseller before sending it to customers.
*/
@JvmName("nlgdwbyigmjdocik")
public suspend fun repricingConfig(`value`: GoogleCloudChannelV1RepricingConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repricingConfig = mapped
}
/**
* @param argument The configuration for bill modifications made by a reseller before sending it to customers.
*/
@JvmName("xayferrjpxnhfxih")
public suspend fun repricingConfig(argument: suspend GoogleCloudChannelV1RepricingConfigArgsBuilder.() -> Unit) {
val toBeMapped = GoogleCloudChannelV1RepricingConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.repricingConfig = mapped
}
internal fun build(): CustomerRepricingConfigArgs = CustomerRepricingConfigArgs(
accountId = accountId,
customerId = customerId,
repricingConfig = repricingConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy