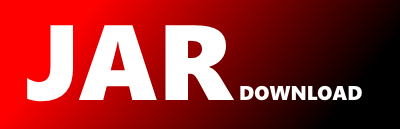
com.pulumi.googlenative.clouddeploy.v1.kotlin.inputs.CanaryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.clouddeploy.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.clouddeploy.v1.inputs.CanaryArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Canary represents the canary deployment strategy.
* @property canaryDeployment Configures the progressive based deployment for a Target.
* @property customCanaryDeployment Configures the progressive based deployment for a Target, but allows customizing at the phase level where a phase represents each of the percentage deployments.
* @property runtimeConfig Optional. Runtime specific configurations for the deployment strategy. The runtime configuration is used to determine how Cloud Deploy will split traffic to enable a progressive deployment.
*/
public data class CanaryArgs(
public val canaryDeployment: Output? = null,
public val customCanaryDeployment: Output? = null,
public val runtimeConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.clouddeploy.v1.inputs.CanaryArgs =
com.pulumi.googlenative.clouddeploy.v1.inputs.CanaryArgs.builder()
.canaryDeployment(canaryDeployment?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.customCanaryDeployment(
customCanaryDeployment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.runtimeConfig(runtimeConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CanaryArgs].
*/
@PulumiTagMarker
public class CanaryArgsBuilder internal constructor() {
private var canaryDeployment: Output? = null
private var customCanaryDeployment: Output? = null
private var runtimeConfig: Output? = null
/**
* @param value Configures the progressive based deployment for a Target.
*/
@JvmName("psnggxksqfeugmpe")
public suspend fun canaryDeployment(`value`: Output) {
this.canaryDeployment = value
}
/**
* @param value Configures the progressive based deployment for a Target, but allows customizing at the phase level where a phase represents each of the percentage deployments.
*/
@JvmName("mamiwxxeocakoxau")
public suspend fun customCanaryDeployment(`value`: Output) {
this.customCanaryDeployment = value
}
/**
* @param value Optional. Runtime specific configurations for the deployment strategy. The runtime configuration is used to determine how Cloud Deploy will split traffic to enable a progressive deployment.
*/
@JvmName("hqfdurjctvmahmho")
public suspend fun runtimeConfig(`value`: Output) {
this.runtimeConfig = value
}
/**
* @param value Configures the progressive based deployment for a Target.
*/
@JvmName("ttidbbeglnlokugb")
public suspend fun canaryDeployment(`value`: CanaryDeploymentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.canaryDeployment = mapped
}
/**
* @param argument Configures the progressive based deployment for a Target.
*/
@JvmName("nfydfaspyrllntmq")
public suspend fun canaryDeployment(argument: suspend CanaryDeploymentArgsBuilder.() -> Unit) {
val toBeMapped = CanaryDeploymentArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.canaryDeployment = mapped
}
/**
* @param value Configures the progressive based deployment for a Target, but allows customizing at the phase level where a phase represents each of the percentage deployments.
*/
@JvmName("gghjboqqexpbpwni")
public suspend fun customCanaryDeployment(`value`: CustomCanaryDeploymentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customCanaryDeployment = mapped
}
/**
* @param argument Configures the progressive based deployment for a Target, but allows customizing at the phase level where a phase represents each of the percentage deployments.
*/
@JvmName("vsabaxwhwkfqvttl")
public suspend fun customCanaryDeployment(argument: suspend CustomCanaryDeploymentArgsBuilder.() -> Unit) {
val toBeMapped = CustomCanaryDeploymentArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.customCanaryDeployment = mapped
}
/**
* @param value Optional. Runtime specific configurations for the deployment strategy. The runtime configuration is used to determine how Cloud Deploy will split traffic to enable a progressive deployment.
*/
@JvmName("rqsdowlknmtjwyvn")
public suspend fun runtimeConfig(`value`: RuntimeConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.runtimeConfig = mapped
}
/**
* @param argument Optional. Runtime specific configurations for the deployment strategy. The runtime configuration is used to determine how Cloud Deploy will split traffic to enable a progressive deployment.
*/
@JvmName("crxgbttkmxuywdrw")
public suspend fun runtimeConfig(argument: suspend RuntimeConfigArgsBuilder.() -> Unit) {
val toBeMapped = RuntimeConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.runtimeConfig = mapped
}
internal fun build(): CanaryArgs = CanaryArgs(
canaryDeployment = canaryDeployment,
customCanaryDeployment = customCanaryDeployment,
runtimeConfig = runtimeConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy