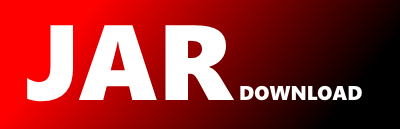
com.pulumi.googlenative.cloudfunctions.v2.kotlin.Cloudfunctions_v2Functions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudfunctions.v2.kotlin
import com.pulumi.googlenative.cloudfunctions.v2.Cloudfunctions_v2Functions.getFunctionIamPolicyPlain
import com.pulumi.googlenative.cloudfunctions.v2.Cloudfunctions_v2Functions.getFunctionPlain
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionIamPolicyPlainArgs
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionIamPolicyPlainArgsBuilder
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionPlainArgs
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionPlainArgsBuilder
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.outputs.GetFunctionIamPolicyResult
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.outputs.GetFunctionResult
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.outputs.GetFunctionIamPolicyResult.Companion.toKotlin as getFunctionIamPolicyResultToKotlin
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.outputs.GetFunctionResult.Companion.toKotlin as getFunctionResultToKotlin
public object Cloudfunctions_v2Functions {
/**
* Returns a function with the given name from the requested project.
* @param argument null
* @return null
*/
public suspend fun getFunction(argument: GetFunctionPlainArgs): GetFunctionResult =
getFunctionResultToKotlin(getFunctionPlain(argument.toJava()).await())
/**
* @see [getFunction].
* @param functionId
* @param location
* @param project
* @return null
*/
public suspend fun getFunction(
functionId: String,
location: String,
project: String? = null,
): GetFunctionResult {
val argument = GetFunctionPlainArgs(
functionId = functionId,
location = location,
project = project,
)
return getFunctionResultToKotlin(getFunctionPlain(argument.toJava()).await())
}
/**
* @see [getFunction].
* @param argument Builder for [com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionPlainArgs].
* @return null
*/
public suspend fun getFunction(argument: suspend GetFunctionPlainArgsBuilder.() -> Unit): GetFunctionResult {
val builder = GetFunctionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFunctionResultToKotlin(getFunctionPlain(builtArgument.toJava()).await())
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
* @param argument null
* @return null
*/
public suspend fun getFunctionIamPolicy(argument: GetFunctionIamPolicyPlainArgs): GetFunctionIamPolicyResult =
getFunctionIamPolicyResultToKotlin(getFunctionIamPolicyPlain(argument.toJava()).await())
/**
* @see [getFunctionIamPolicy].
* @param functionId
* @param location
* @param optionsRequestedPolicyVersion
* @param project
* @return null
*/
public suspend fun getFunctionIamPolicy(
functionId: String,
location: String,
optionsRequestedPolicyVersion: Int? = null,
project: String? = null,
): GetFunctionIamPolicyResult {
val argument = GetFunctionIamPolicyPlainArgs(
functionId = functionId,
location = location,
optionsRequestedPolicyVersion = optionsRequestedPolicyVersion,
project = project,
)
return getFunctionIamPolicyResultToKotlin(getFunctionIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getFunctionIamPolicy].
* @param argument Builder for [com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.GetFunctionIamPolicyPlainArgs].
* @return null
*/
public suspend fun getFunctionIamPolicy(argument: suspend GetFunctionIamPolicyPlainArgsBuilder.() -> Unit): GetFunctionIamPolicyResult {
val builder = GetFunctionIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFunctionIamPolicyResultToKotlin(getFunctionIamPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy