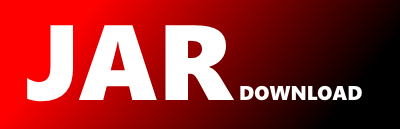
com.pulumi.googlenative.cloudfunctions.v2.kotlin.FunctionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudfunctions.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudfunctions.v2.FunctionArgs.builder
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.enums.FunctionEnvironment
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.BuildConfigArgs
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.BuildConfigArgsBuilder
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.EventTriggerArgs
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.EventTriggerArgsBuilder
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.ServiceConfigArgs
import com.pulumi.googlenative.cloudfunctions.v2.kotlin.inputs.ServiceConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a new function. If a function with the given name already exists in the specified project, the long running operation will return `ALREADY_EXISTS` error.
* @property buildConfig Describes the Build step of the function that builds a container from the given source.
* @property description User-provided description of a function.
* @property environment Describe whether the function is 1st Gen or 2nd Gen.
* @property eventTrigger An Eventarc trigger managed by Google Cloud Functions that fires events in response to a condition in another service.
* @property functionId The ID to use for the function, which will become the final component of the function's resource name. This value should be 4-63 characters, and valid characters are /a-z-/.
* @property kmsKeyName [Preview] Resource name of a KMS crypto key (managed by the user) used to encrypt/decrypt function resources. It must match the pattern `projects/{project}/locations/{location}/keyRings/{key_ring}/cryptoKeys/{crypto_key}`.
* @property labels Labels associated with this Cloud Function.
* @property location
* @property name A user-defined name of the function. Function names must be unique globally and match pattern `projects/*/locations/*/functions/*`
* @property project
* @property serviceConfig Describes the Service being deployed. Currently deploys services to Cloud Run (fully managed).
* */*/*/
*/
public data class FunctionArgs(
public val buildConfig: Output? = null,
public val description: Output? = null,
public val environment: Output? = null,
public val eventTrigger: Output? = null,
public val functionId: Output? = null,
public val kmsKeyName: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy