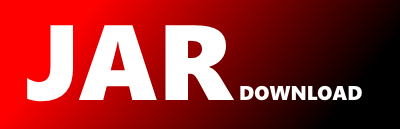
com.pulumi.googlenative.cloudidentity.v1.kotlin.GroupArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudidentity.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudidentity.v1.GroupArgs.builder
import com.pulumi.googlenative.cloudidentity.v1.kotlin.inputs.DynamicGroupMetadataArgs
import com.pulumi.googlenative.cloudidentity.v1.kotlin.inputs.DynamicGroupMetadataArgsBuilder
import com.pulumi.googlenative.cloudidentity.v1.kotlin.inputs.EntityKeyArgs
import com.pulumi.googlenative.cloudidentity.v1.kotlin.inputs.EntityKeyArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a Group.
* Auto-naming is currently not supported for this resource.
* @property description An extended description to help users determine the purpose of a `Group`. Must not be longer than 4,096 characters.
* @property displayName The display name of the `Group`.
* @property dynamicGroupMetadata Optional. Dynamic group metadata like queries and status.
* @property groupKey The `EntityKey` of the `Group`.
* @property initialGroupConfig Optional. The initial configuration option for the `Group`.
* @property labels One or more label entries that apply to the Group. Currently supported labels contain a key with an empty value. Google Groups are the default type of group and have a label with a key of `cloudidentity.googleapis.com/groups.discussion_forum` and an empty value. Existing Google Groups can have an additional label with a key of `cloudidentity.googleapis.com/groups.security` and an empty value added to them. **This is an immutable change and the security label cannot be removed once added.** Dynamic groups have a label with a key of `cloudidentity.googleapis.com/groups.dynamic`. Identity-mapped groups for Cloud Search have a label with a key of `system/groups/external` and an empty value.
* @property parent Immutable. The resource name of the entity under which this `Group` resides in the Cloud Identity resource hierarchy. Must be of the form `identitysources/{identity_source}` for external [identity-mapped groups](https://support.google.com/a/answer/9039510) or `customers/{customer_id}` for Google Groups. The `customer_id` must begin with "C" (for example, 'C046psxkn'). [Find your customer ID.] (https://support.google.com/cloudidentity/answer/10070793)
*/
public data class GroupArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val dynamicGroupMetadata: Output? = null,
public val groupKey: Output? = null,
public val initialGroupConfig: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy