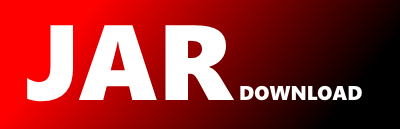
com.pulumi.googlenative.cloudiot.v1.kotlin.Registry.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudiot.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.EventNotificationConfigResponse
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.HttpConfigResponse
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.MqttConfigResponse
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.RegistryCredentialResponse
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.StateNotificationConfigResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.EventNotificationConfigResponse.Companion.toKotlin as eventNotificationConfigResponseToKotlin
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.HttpConfigResponse.Companion.toKotlin as httpConfigResponseToKotlin
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.MqttConfigResponse.Companion.toKotlin as mqttConfigResponseToKotlin
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.RegistryCredentialResponse.Companion.toKotlin as registryCredentialResponseToKotlin
import com.pulumi.googlenative.cloudiot.v1.kotlin.outputs.StateNotificationConfigResponse.Companion.toKotlin as stateNotificationConfigResponseToKotlin
/**
* Builder for [Registry].
*/
@PulumiTagMarker
public class RegistryResourceBuilder internal constructor() {
public var name: String? = null
public var args: RegistryArgs = RegistryArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RegistryArgsBuilder.() -> Unit) {
val builder = RegistryArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Registry {
val builtJavaResource = com.pulumi.googlenative.cloudiot.v1.Registry(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Registry(builtJavaResource)
}
}
/**
* Creates a device registry that contains devices.
*/
public class Registry internal constructor(
override val javaResource: com.pulumi.googlenative.cloudiot.v1.Registry,
) : KotlinCustomResource(javaResource, RegistryMapper) {
/**
* The credentials used to verify the device credentials. No more than 10 credentials can be bound to a single registry at a time. The verification process occurs at the time of device creation or update. If this field is empty, no verification is performed. Otherwise, the credentials of a newly created device or added credentials of an updated device should be signed with one of these registry credentials. Note, however, that existing devices will never be affected by modifications to this list of credentials: after a device has been successfully created in a registry, it should be able to connect even if its registry credentials are revoked, deleted, or modified.
*/
public val credentials: Output>
get() = javaResource.credentials().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
registryCredentialResponseToKotlin(args0)
})
})
})
/**
* The configuration for notification of telemetry events received from the device. All telemetry events that were successfully published by the device and acknowledged by Cloud IoT Core are guaranteed to be delivered to Cloud Pub/Sub. If multiple configurations match a message, only the first matching configuration is used. If you try to publish a device telemetry event using MQTT without specifying a Cloud Pub/Sub topic for the device's registry, the connection closes automatically. If you try to do so using an HTTP connection, an error is returned. Up to 10 configurations may be provided.
*/
public val eventNotificationConfigs: Output>
get() = javaResource.eventNotificationConfigs().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> eventNotificationConfigResponseToKotlin(args0) })
})
})
/**
* The DeviceService (HTTP) configuration for this device registry.
*/
public val httpConfig: Output
get() = javaResource.httpConfig().applyValue({ args0 ->
args0.let({ args0 ->
httpConfigResponseToKotlin(args0)
})
})
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* **Beta Feature** The default logging verbosity for activity from devices in this registry. The verbosity level can be overridden by Device.log_level.
*/
public val logLevel: Output
get() = javaResource.logLevel().applyValue({ args0 -> args0 })
/**
* The MQTT configuration for this device registry.
*/
public val mqttConfig: Output
get() = javaResource.mqttConfig().applyValue({ args0 ->
args0.let({ args0 ->
mqttConfigResponseToKotlin(args0)
})
})
/**
* The resource path name. For example, `projects/example-project/locations/us-central1/registries/my-registry`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The configuration for notification of new states received from the device. State updates are guaranteed to be stored in the state history, but notifications to Cloud Pub/Sub are not guaranteed. For example, if permissions are misconfigured or the specified topic doesn't exist, no notification will be published but the state will still be stored in Cloud IoT Core.
*/
public val stateNotificationConfig: Output
get() = javaResource.stateNotificationConfig().applyValue({ args0 ->
args0.let({ args0 ->
stateNotificationConfigResponseToKotlin(args0)
})
})
}
public object RegistryMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.cloudiot.v1.Registry::class == javaResource::class
override fun map(javaResource: Resource): Registry = Registry(
javaResource as
com.pulumi.googlenative.cloudiot.v1.Registry,
)
}
/**
* @see [Registry].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Registry].
*/
public suspend fun registry(name: String, block: suspend RegistryResourceBuilder.() -> Unit): Registry {
val builder = RegistryResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Registry].
* @param name The _unique_ name of the resulting resource.
*/
public fun registry(name: String): Registry {
val builder = RegistryResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy