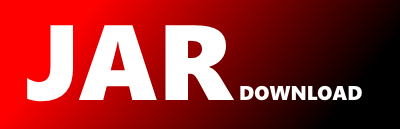
com.pulumi.googlenative.cloudkms.v1.kotlin.CryptoKeyVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudkms.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudkms.v1.CryptoKeyVersionArgs.builder
import com.pulumi.googlenative.cloudkms.v1.kotlin.enums.CryptoKeyVersionState
import com.pulumi.googlenative.cloudkms.v1.kotlin.inputs.ExternalProtectionLevelOptionsArgs
import com.pulumi.googlenative.cloudkms.v1.kotlin.inputs.ExternalProtectionLevelOptionsArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Create a new CryptoKeyVersion in a CryptoKey. The server will assign the next sequential id. If unset, state will be set to ENABLED.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property cryptoKeyId
* @property externalProtectionLevelOptions ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
* @property keyRingId
* @property location
* @property project
* @property state The current state of the CryptoKeyVersion.
*/
public data class CryptoKeyVersionArgs(
public val cryptoKeyId: Output? = null,
public val externalProtectionLevelOptions: Output? = null,
public val keyRingId: Output? = null,
public val location: Output? = null,
public val project: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudkms.v1.CryptoKeyVersionArgs =
com.pulumi.googlenative.cloudkms.v1.CryptoKeyVersionArgs.builder()
.cryptoKeyId(cryptoKeyId?.applyValue({ args0 -> args0 }))
.externalProtectionLevelOptions(
externalProtectionLevelOptions?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.keyRingId(keyRingId?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CryptoKeyVersionArgs].
*/
@PulumiTagMarker
public class CryptoKeyVersionArgsBuilder internal constructor() {
private var cryptoKeyId: Output? = null
private var externalProtectionLevelOptions: Output? = null
private var keyRingId: Output? = null
private var location: Output? = null
private var project: Output? = null
private var state: Output? = null
/**
* @param value
*/
@JvmName("omudfwbfhlyieyxs")
public suspend fun cryptoKeyId(`value`: Output) {
this.cryptoKeyId = value
}
/**
* @param value ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
*/
@JvmName("ipfnqxrkixxddldy")
public suspend fun externalProtectionLevelOptions(`value`: Output) {
this.externalProtectionLevelOptions = value
}
/**
* @param value
*/
@JvmName("jqxfstlniauklhcc")
public suspend fun keyRingId(`value`: Output) {
this.keyRingId = value
}
/**
* @param value
*/
@JvmName("taqeahkosvvdydtr")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value
*/
@JvmName("abvkxkytqkanbvio")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The current state of the CryptoKeyVersion.
*/
@JvmName("vnhmrbdtvdmwchok")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value
*/
@JvmName("xvcfasgtwjwspknt")
public suspend fun cryptoKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cryptoKeyId = mapped
}
/**
* @param value ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
*/
@JvmName("lpspvpubmvwovvhh")
public suspend fun externalProtectionLevelOptions(`value`: ExternalProtectionLevelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalProtectionLevelOptions = mapped
}
/**
* @param argument ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
*/
@JvmName("qnxmtprwbmavqtue")
public suspend fun externalProtectionLevelOptions(argument: suspend ExternalProtectionLevelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = ExternalProtectionLevelOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.externalProtectionLevelOptions = mapped
}
/**
* @param value
*/
@JvmName("akmnqehrotvfwsrj")
public suspend fun keyRingId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyRingId = mapped
}
/**
* @param value
*/
@JvmName("pnuibwrbpgrpdpxu")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value
*/
@JvmName("vhlvqebcohsdqmhy")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The current state of the CryptoKeyVersion.
*/
@JvmName("dmgtitiiwnkgbwhs")
public suspend fun state(`value`: CryptoKeyVersionState?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): CryptoKeyVersionArgs = CryptoKeyVersionArgs(
cryptoKeyId = cryptoKeyId,
externalProtectionLevelOptions = externalProtectionLevelOptions,
keyRingId = keyRingId,
location = location,
project = project,
state = state,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy