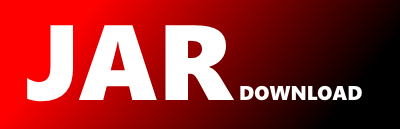
com.pulumi.googlenative.cloudresourcemanager.v1.kotlin.LienArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudresourcemanager.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudresourcemanager.v1.LienArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Create a Lien which applies to the resource denoted by the `parent` field. Callers of this method will require permission on the `parent` resource. For example, applying to `projects/1234` requires permission `resourcemanager.projects.updateLiens`. NOTE: Some resources may limit the number of Liens which may be applied.
* @property createTime The creation time of this Lien.
* @property name A system-generated unique identifier for this Lien. Example: `liens/1234abcd`
* @property origin A stable, user-visible/meaningful string identifying the origin of the Lien, intended to be inspected programmatically. Maximum length of 200 characters. Example: 'compute.googleapis.com'
* @property parent A reference to the resource this Lien is attached to. The server will validate the parent against those for which Liens are supported. Example: `projects/1234`
* @property reason Concise user-visible strings indicating why an action cannot be performed on a resource. Maximum length of 200 characters. Example: 'Holds production API key'
* @property restrictions The types of operations which should be blocked as a result of this Lien. Each value should correspond to an IAM permission. The server will validate the permissions against those for which Liens are supported. An empty list is meaningless and will be rejected. Example: ['resourcemanager.projects.delete']
*/
public data class LienArgs(
public val createTime: Output? = null,
public val name: Output? = null,
public val origin: Output? = null,
public val parent: Output? = null,
public val reason: Output? = null,
public val restrictions: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudresourcemanager.v1.LienArgs =
com.pulumi.googlenative.cloudresourcemanager.v1.LienArgs.builder()
.createTime(createTime?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.origin(origin?.applyValue({ args0 -> args0 }))
.parent(parent?.applyValue({ args0 -> args0 }))
.reason(reason?.applyValue({ args0 -> args0 }))
.restrictions(restrictions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [LienArgs].
*/
@PulumiTagMarker
public class LienArgsBuilder internal constructor() {
private var createTime: Output? = null
private var name: Output? = null
private var origin: Output? = null
private var parent: Output? = null
private var reason: Output? = null
private var restrictions: Output>? = null
/**
* @param value The creation time of this Lien.
*/
@JvmName("aesdugqastrrucqh")
public suspend fun createTime(`value`: Output) {
this.createTime = value
}
/**
* @param value A system-generated unique identifier for this Lien. Example: `liens/1234abcd`
*/
@JvmName("bnpidxfvhxtfwpmj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A stable, user-visible/meaningful string identifying the origin of the Lien, intended to be inspected programmatically. Maximum length of 200 characters. Example: 'compute.googleapis.com'
*/
@JvmName("ywjminnnfxdswwyp")
public suspend fun origin(`value`: Output) {
this.origin = value
}
/**
* @param value A reference to the resource this Lien is attached to. The server will validate the parent against those for which Liens are supported. Example: `projects/1234`
*/
@JvmName("hysrhsndgdqnwogv")
public suspend fun parent(`value`: Output) {
this.parent = value
}
/**
* @param value Concise user-visible strings indicating why an action cannot be performed on a resource. Maximum length of 200 characters. Example: 'Holds production API key'
*/
@JvmName("ifrfeadjqdocbysl")
public suspend fun reason(`value`: Output) {
this.reason = value
}
/**
* @param value The types of operations which should be blocked as a result of this Lien. Each value should correspond to an IAM permission. The server will validate the permissions against those for which Liens are supported. An empty list is meaningless and will be rejected. Example: ['resourcemanager.projects.delete']
*/
@JvmName("cnckdxdidqdfpltf")
public suspend fun restrictions(`value`: Output>) {
this.restrictions = value
}
@JvmName("btjgyjdcwacvibbb")
public suspend fun restrictions(vararg values: Output) {
this.restrictions = Output.all(values.asList())
}
/**
* @param values The types of operations which should be blocked as a result of this Lien. Each value should correspond to an IAM permission. The server will validate the permissions against those for which Liens are supported. An empty list is meaningless and will be rejected. Example: ['resourcemanager.projects.delete']
*/
@JvmName("ebsjfpvcvtwafjqn")
public suspend fun restrictions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy