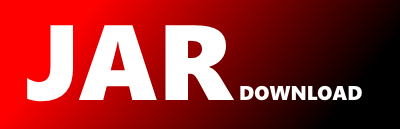
com.pulumi.googlenative.cloudsearch.v1.kotlin.inputs.FacetOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.cloudsearch.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.cloudsearch.v1.inputs.FacetOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Specifies operators to return facet results for. There will be one FacetResult for every source_name/object_type/operator_name combination.
* @property integerFacetingOptions If set, describes integer faceting options for the given integer property. The corresponding integer property in the schema should be marked isFacetable. The number of buckets returned would be minimum of this and num_facet_buckets.
* @property numFacetBuckets Maximum number of facet buckets that should be returned for this facet. Defaults to 10. Maximum value is 100.
* @property objectType If object_type is set, only those objects of that type will be used to compute facets. If empty, then all objects will be used to compute facets.
* @property operatorName The name of the operator chosen for faceting. @see cloudsearch.SchemaPropertyOptions
* @property sourceName Source name to facet on. Format: datasources/{source_id} If empty, all data sources will be used.
*/
public data class FacetOptionsArgs(
public val integerFacetingOptions: Output? = null,
public val numFacetBuckets: Output? = null,
public val objectType: Output? = null,
public val operatorName: Output? = null,
public val sourceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.cloudsearch.v1.inputs.FacetOptionsArgs =
com.pulumi.googlenative.cloudsearch.v1.inputs.FacetOptionsArgs.builder()
.integerFacetingOptions(
integerFacetingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.numFacetBuckets(numFacetBuckets?.applyValue({ args0 -> args0 }))
.objectType(objectType?.applyValue({ args0 -> args0 }))
.operatorName(operatorName?.applyValue({ args0 -> args0 }))
.sourceName(sourceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FacetOptionsArgs].
*/
@PulumiTagMarker
public class FacetOptionsArgsBuilder internal constructor() {
private var integerFacetingOptions: Output? = null
private var numFacetBuckets: Output? = null
private var objectType: Output? = null
private var operatorName: Output? = null
private var sourceName: Output? = null
/**
* @param value If set, describes integer faceting options for the given integer property. The corresponding integer property in the schema should be marked isFacetable. The number of buckets returned would be minimum of this and num_facet_buckets.
*/
@JvmName("mhocoylisuucawuu")
public suspend fun integerFacetingOptions(`value`: Output) {
this.integerFacetingOptions = value
}
/**
* @param value Maximum number of facet buckets that should be returned for this facet. Defaults to 10. Maximum value is 100.
*/
@JvmName("xscyleipgfowlhxr")
public suspend fun numFacetBuckets(`value`: Output) {
this.numFacetBuckets = value
}
/**
* @param value If object_type is set, only those objects of that type will be used to compute facets. If empty, then all objects will be used to compute facets.
*/
@JvmName("rwgawtcpbmgvvwgo")
public suspend fun objectType(`value`: Output) {
this.objectType = value
}
/**
* @param value The name of the operator chosen for faceting. @see cloudsearch.SchemaPropertyOptions
*/
@JvmName("ljlkrcdwditetnfk")
public suspend fun operatorName(`value`: Output) {
this.operatorName = value
}
/**
* @param value Source name to facet on. Format: datasources/{source_id} If empty, all data sources will be used.
*/
@JvmName("mvgigseqkkfxjsuh")
public suspend fun sourceName(`value`: Output) {
this.sourceName = value
}
/**
* @param value If set, describes integer faceting options for the given integer property. The corresponding integer property in the schema should be marked isFacetable. The number of buckets returned would be minimum of this and num_facet_buckets.
*/
@JvmName("qrwxlfrvynwpsxby")
public suspend fun integerFacetingOptions(`value`: IntegerFacetingOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integerFacetingOptions = mapped
}
/**
* @param argument If set, describes integer faceting options for the given integer property. The corresponding integer property in the schema should be marked isFacetable. The number of buckets returned would be minimum of this and num_facet_buckets.
*/
@JvmName("xobamjbuoiovenkv")
public suspend fun integerFacetingOptions(argument: suspend IntegerFacetingOptionsArgsBuilder.() -> Unit) {
val toBeMapped = IntegerFacetingOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.integerFacetingOptions = mapped
}
/**
* @param value Maximum number of facet buckets that should be returned for this facet. Defaults to 10. Maximum value is 100.
*/
@JvmName("tcjnsjvngvggedfk")
public suspend fun numFacetBuckets(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.numFacetBuckets = mapped
}
/**
* @param value If object_type is set, only those objects of that type will be used to compute facets. If empty, then all objects will be used to compute facets.
*/
@JvmName("lkfycqcpfvtajqbk")
public suspend fun objectType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.objectType = mapped
}
/**
* @param value The name of the operator chosen for faceting. @see cloudsearch.SchemaPropertyOptions
*/
@JvmName("vwuwnycmwicgdpfo")
public suspend fun operatorName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operatorName = mapped
}
/**
* @param value Source name to facet on. Format: datasources/{source_id} If empty, all data sources will be used.
*/
@JvmName("txfllfjnvosgvxmv")
public suspend fun sourceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceName = mapped
}
internal fun build(): FacetOptionsArgs = FacetOptionsArgs(
integerFacetingOptions = integerFacetingOptions,
numFacetBuckets = numFacetBuckets,
objectType = objectType,
operatorName = operatorName,
sourceName = sourceName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy