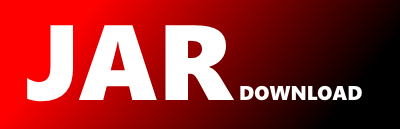
com.pulumi.googlenative.compute.beta.kotlin.RegionSslCertificateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.compute.beta.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.compute.beta.RegionSslCertificateArgs.builder
import com.pulumi.googlenative.compute.beta.kotlin.enums.RegionSslCertificateType
import com.pulumi.googlenative.compute.beta.kotlin.inputs.SslCertificateManagedSslCertificateArgs
import com.pulumi.googlenative.compute.beta.kotlin.inputs.SslCertificateManagedSslCertificateArgsBuilder
import com.pulumi.googlenative.compute.beta.kotlin.inputs.SslCertificateSelfManagedSslCertificateArgs
import com.pulumi.googlenative.compute.beta.kotlin.inputs.SslCertificateSelfManagedSslCertificateArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Creates a SslCertificate resource in the specified project and region using the data included in the request
* @property certificate A value read into memory from a certificate file. The certificate file must be in PEM format. The certificate chain must be no greater than 5 certs long. The chain must include at least one intermediate cert.
* @property description An optional description of this resource. Provide this property when you create the resource.
* @property managed Configuration and status of a managed SSL certificate.
* @property name Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
* @property privateKey A value read into memory from a write-only private key file. The private key file must be in PEM format. For security, only insert requests include this field.
* @property project
* @property region
* @property requestId An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
* @property selfManaged Configuration and status of a self-managed SSL certificate.
* @property type (Optional) Specifies the type of SSL certificate, either "SELF_MANAGED" or "MANAGED". If not specified, the certificate is self-managed and the fields certificate and private_key are used.
*/
public data class RegionSslCertificateArgs(
public val certificate: Output? = null,
public val description: Output? = null,
public val managed: Output? = null,
public val name: Output? = null,
public val privateKey: Output? = null,
public val project: Output? = null,
public val region: Output? = null,
public val requestId: Output? = null,
public val selfManaged: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.compute.beta.RegionSslCertificateArgs =
com.pulumi.googlenative.compute.beta.RegionSslCertificateArgs.builder()
.certificate(certificate?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.managed(managed?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.privateKey(privateKey?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.region(region?.applyValue({ args0 -> args0 }))
.requestId(requestId?.applyValue({ args0 -> args0 }))
.selfManaged(selfManaged?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RegionSslCertificateArgs].
*/
@PulumiTagMarker
public class RegionSslCertificateArgsBuilder internal constructor() {
private var certificate: Output? = null
private var description: Output? = null
private var managed: Output? = null
private var name: Output? = null
private var privateKey: Output? = null
private var project: Output? = null
private var region: Output? = null
private var requestId: Output? = null
private var selfManaged: Output? = null
private var type: Output? = null
/**
* @param value A value read into memory from a certificate file. The certificate file must be in PEM format. The certificate chain must be no greater than 5 certs long. The chain must include at least one intermediate cert.
*/
@JvmName("posgbgabvixlfear")
public suspend fun certificate(`value`: Output) {
this.certificate = value
}
/**
* @param value An optional description of this resource. Provide this property when you create the resource.
*/
@JvmName("ngfgiaqehxdlealb")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Configuration and status of a managed SSL certificate.
*/
@JvmName("umrwltqchwadyich")
public suspend fun managed(`value`: Output) {
this.managed = value
}
/**
* @param value Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*/
@JvmName("ouirstsntqubevto")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A value read into memory from a write-only private key file. The private key file must be in PEM format. For security, only insert requests include this field.
*/
@JvmName("ltkvedlyogmosuay")
public suspend fun privateKey(`value`: Output) {
this.privateKey = value
}
/**
* @param value
*/
@JvmName("rdmcototqwybrbhl")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value
*/
@JvmName("btpvqjjpvcpuqcfe")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*/
@JvmName("rigmbwaxepynqdap")
public suspend fun requestId(`value`: Output) {
this.requestId = value
}
/**
* @param value Configuration and status of a self-managed SSL certificate.
*/
@JvmName("nsarhpxnrdlmdpcy")
public suspend fun selfManaged(`value`: Output) {
this.selfManaged = value
}
/**
* @param value (Optional) Specifies the type of SSL certificate, either "SELF_MANAGED" or "MANAGED". If not specified, the certificate is self-managed and the fields certificate and private_key are used.
*/
@JvmName("gnuyhwifbwpojgrc")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value A value read into memory from a certificate file. The certificate file must be in PEM format. The certificate chain must be no greater than 5 certs long. The chain must include at least one intermediate cert.
*/
@JvmName("mimbddajtetbdeya")
public suspend fun certificate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificate = mapped
}
/**
* @param value An optional description of this resource. Provide this property when you create the resource.
*/
@JvmName("uwnovmfivdywymux")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Configuration and status of a managed SSL certificate.
*/
@JvmName("cirjryqboejceqow")
public suspend fun managed(`value`: SslCertificateManagedSslCertificateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managed = mapped
}
/**
* @param argument Configuration and status of a managed SSL certificate.
*/
@JvmName("trdlxtwiqvbfsxmr")
public suspend fun managed(argument: suspend SslCertificateManagedSslCertificateArgsBuilder.() -> Unit) {
val toBeMapped = SslCertificateManagedSslCertificateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.managed = mapped
}
/**
* @param value Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*/
@JvmName("smhqmvlqufbidajn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value A value read into memory from a write-only private key file. The private key file must be in PEM format. For security, only insert requests include this field.
*/
@JvmName("tlmnfisbrfhximki")
public suspend fun privateKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateKey = mapped
}
/**
* @param value
*/
@JvmName("nldvvjxceayjtowv")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value
*/
@JvmName("tivhnhvwweqwidmq")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*/
@JvmName("orcqiwniycqifwkg")
public suspend fun requestId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestId = mapped
}
/**
* @param value Configuration and status of a self-managed SSL certificate.
*/
@JvmName("dwmuhairrgfujohe")
public suspend fun selfManaged(`value`: SslCertificateSelfManagedSslCertificateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selfManaged = mapped
}
/**
* @param argument Configuration and status of a self-managed SSL certificate.
*/
@JvmName("atgrilnxnkposxoe")
public suspend fun selfManaged(argument: suspend SslCertificateSelfManagedSslCertificateArgsBuilder.() -> Unit) {
val toBeMapped = SslCertificateSelfManagedSslCertificateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.selfManaged = mapped
}
/**
* @param value (Optional) Specifies the type of SSL certificate, either "SELF_MANAGED" or "MANAGED". If not specified, the certificate is self-managed and the fields certificate and private_key are used.
*/
@JvmName("ncnlpxubcsaeoqct")
public suspend fun type(`value`: RegionSslCertificateType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): RegionSslCertificateArgs = RegionSslCertificateArgs(
certificate = certificate,
description = description,
managed = managed,
name = name,
privateKey = privateKey,
project = project,
region = region,
requestId = requestId,
selfManaged = selfManaged,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy