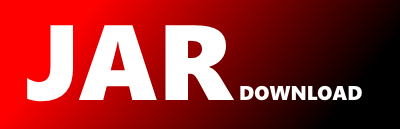
com.pulumi.googlenative.compute.v1.kotlin.Image.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.compute.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.compute.v1.kotlin.outputs.CustomerEncryptionKeyResponse
import com.pulumi.googlenative.compute.v1.kotlin.outputs.DeprecationStatusResponse
import com.pulumi.googlenative.compute.v1.kotlin.outputs.GuestOsFeatureResponse
import com.pulumi.googlenative.compute.v1.kotlin.outputs.ImageRawDiskResponse
import com.pulumi.googlenative.compute.v1.kotlin.outputs.InitialStateConfigResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.compute.v1.kotlin.outputs.CustomerEncryptionKeyResponse.Companion.toKotlin as customerEncryptionKeyResponseToKotlin
import com.pulumi.googlenative.compute.v1.kotlin.outputs.DeprecationStatusResponse.Companion.toKotlin as deprecationStatusResponseToKotlin
import com.pulumi.googlenative.compute.v1.kotlin.outputs.GuestOsFeatureResponse.Companion.toKotlin as guestOsFeatureResponseToKotlin
import com.pulumi.googlenative.compute.v1.kotlin.outputs.ImageRawDiskResponse.Companion.toKotlin as imageRawDiskResponseToKotlin
import com.pulumi.googlenative.compute.v1.kotlin.outputs.InitialStateConfigResponse.Companion.toKotlin as initialStateConfigResponseToKotlin
/**
* Builder for [Image].
*/
@PulumiTagMarker
public class ImageResourceBuilder internal constructor() {
public var name: String? = null
public var args: ImageArgs = ImageArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ImageArgsBuilder.() -> Unit) {
val builder = ImageArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Image {
val builtJavaResource = com.pulumi.googlenative.compute.v1.Image(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Image(builtJavaResource)
}
}
/**
* Creates an image in the specified project using the data included in the request.
*/
public class Image internal constructor(
override val javaResource: com.pulumi.googlenative.compute.v1.Image,
) : KotlinCustomResource(javaResource, ImageMapper) {
/**
* The architecture of the image. Valid values are ARM64 or X86_64.
*/
public val architecture: Output
get() = javaResource.architecture().applyValue({ args0 -> args0 })
/**
* Size of the image tar.gz archive stored in Google Cloud Storage (in bytes).
*/
public val archiveSizeBytes: Output
get() = javaResource.archiveSizeBytes().applyValue({ args0 -> args0 })
/**
* Creation timestamp in RFC3339 text format.
*/
public val creationTimestamp: Output
get() = javaResource.creationTimestamp().applyValue({ args0 -> args0 })
/**
* The deprecation status associated with this image.
*/
public val deprecated: Output
get() = javaResource.deprecated().applyValue({ args0 ->
args0.let({ args0 ->
deprecationStatusResponseToKotlin(args0)
})
})
/**
* An optional description of this resource. Provide this property when you create the resource.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Size of the image when restored onto a persistent disk (in GB).
*/
public val diskSizeGb: Output
get() = javaResource.diskSizeGb().applyValue({ args0 -> args0 })
/**
* The name of the image family to which this image belongs. The image family name can be from a publicly managed image family provided by Compute Engine, or from a custom image family you create. For example, centos-stream-9 is a publicly available image family. For more information, see Image family best practices. When creating disks, you can specify an image family instead of a specific image name. The image family always returns its latest image that is not deprecated. The name of the image family must comply with RFC1035.
*/
public val family: Output
get() = javaResource.family().applyValue({ args0 -> args0 })
/**
* Force image creation if true.
*/
public val forceCreate: Output?
get() = javaResource.forceCreate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of features to enable on the guest operating system. Applicable only for bootable images. To see a list of available options, see the guestOSfeatures[].type parameter.
*/
public val guestOsFeatures: Output>
get() = javaResource.guestOsFeatures().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> guestOsFeatureResponseToKotlin(args0) })
})
})
/**
* Encrypts the image using a customer-supplied encryption key. After you encrypt an image with a customer-supplied key, you must provide the same key if you use the image later (e.g. to create a disk from the image). Customer-supplied encryption keys do not protect access to metadata of the disk. If you do not provide an encryption key when creating the image, then the disk will be encrypted using an automatically generated key and you do not need to provide a key to use the image later.
*/
public val imageEncryptionKey: Output
get() = javaResource.imageEncryptionKey().applyValue({ args0 ->
args0.let({ args0 ->
customerEncryptionKeyResponseToKotlin(args0)
})
})
/**
* Type of the resource. Always compute#image for images.
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
/**
* A fingerprint for the labels being applied to this image, which is essentially a hash of the labels used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an image.
*/
public val labelFingerprint: Output
get() = javaResource.labelFingerprint().applyValue({ args0 -> args0 })
/**
* Labels to apply to this image. These can be later modified by the setLabels method.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy