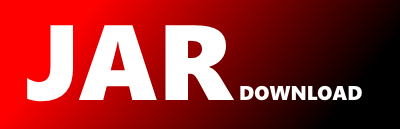
com.pulumi.googlenative.container.v1.kotlin.inputs.PrivateClusterConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.container.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.container.v1.inputs.PrivateClusterConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configuration options for private clusters.
* @property enablePrivateEndpoint Whether the master's internal IP address is used as the cluster endpoint.
* @property enablePrivateNodes Whether nodes have internal IP addresses only. If enabled, all nodes are given only RFC 1918 private addresses and communicate with the master via private networking.
* @property masterGlobalAccessConfig Controls master global access settings.
* @property masterIpv4CidrBlock The IP range in CIDR notation to use for the hosted master network. This range will be used for assigning internal IP addresses to the master or set of masters, as well as the ILB VIP. This range must not overlap with any other ranges in use within the cluster's network.
* @property privateEndpointSubnetwork Subnet to provision the master's private endpoint during cluster creation. Specified in projects/*/regions/*/subnetworks/* format.
* */*/*/
*/
public data class PrivateClusterConfigArgs(
public val enablePrivateEndpoint: Output? = null,
public val enablePrivateNodes: Output? = null,
public val masterGlobalAccessConfig: Output? = null,
public val masterIpv4CidrBlock: Output? = null,
public val privateEndpointSubnetwork: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.container.v1.inputs.PrivateClusterConfigArgs =
com.pulumi.googlenative.container.v1.inputs.PrivateClusterConfigArgs.builder()
.enablePrivateEndpoint(enablePrivateEndpoint?.applyValue({ args0 -> args0 }))
.enablePrivateNodes(enablePrivateNodes?.applyValue({ args0 -> args0 }))
.masterGlobalAccessConfig(
masterGlobalAccessConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.masterIpv4CidrBlock(masterIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.privateEndpointSubnetwork(privateEndpointSubnetwork?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PrivateClusterConfigArgs].
*/
@PulumiTagMarker
public class PrivateClusterConfigArgsBuilder internal constructor() {
private var enablePrivateEndpoint: Output? = null
private var enablePrivateNodes: Output? = null
private var masterGlobalAccessConfig: Output? = null
private var masterIpv4CidrBlock: Output? = null
private var privateEndpointSubnetwork: Output? = null
/**
* @param value Whether the master's internal IP address is used as the cluster endpoint.
*/
@JvmName("xsspcboartayvalq")
public suspend fun enablePrivateEndpoint(`value`: Output) {
this.enablePrivateEndpoint = value
}
/**
* @param value Whether nodes have internal IP addresses only. If enabled, all nodes are given only RFC 1918 private addresses and communicate with the master via private networking.
*/
@JvmName("vgrxpkaoxwksydjh")
public suspend fun enablePrivateNodes(`value`: Output) {
this.enablePrivateNodes = value
}
/**
* @param value Controls master global access settings.
*/
@JvmName("tmbmjkynjdtemsgg")
public suspend fun masterGlobalAccessConfig(`value`: Output) {
this.masterGlobalAccessConfig = value
}
/**
* @param value The IP range in CIDR notation to use for the hosted master network. This range will be used for assigning internal IP addresses to the master or set of masters, as well as the ILB VIP. This range must not overlap with any other ranges in use within the cluster's network.
*/
@JvmName("psvglyrpfmdhbtkk")
public suspend fun masterIpv4CidrBlock(`value`: Output) {
this.masterIpv4CidrBlock = value
}
/**
* @param value Subnet to provision the master's private endpoint during cluster creation. Specified in projects/*/regions/*/subnetworks/* format.
* */*/*/
*/
@JvmName("uqiaroaukryahruc")
public suspend fun privateEndpointSubnetwork(`value`: Output) {
this.privateEndpointSubnetwork = value
}
/**
* @param value Whether the master's internal IP address is used as the cluster endpoint.
*/
@JvmName("alyjblivrpjedmml")
public suspend fun enablePrivateEndpoint(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateEndpoint = mapped
}
/**
* @param value Whether nodes have internal IP addresses only. If enabled, all nodes are given only RFC 1918 private addresses and communicate with the master via private networking.
*/
@JvmName("nhycemrcwvfuelcx")
public suspend fun enablePrivateNodes(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateNodes = mapped
}
/**
* @param value Controls master global access settings.
*/
@JvmName("utuwetkpjyuhbnae")
public suspend fun masterGlobalAccessConfig(`value`: PrivateClusterMasterGlobalAccessConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterGlobalAccessConfig = mapped
}
/**
* @param argument Controls master global access settings.
*/
@JvmName("wuouqhwuvponhvan")
public suspend fun masterGlobalAccessConfig(argument: suspend PrivateClusterMasterGlobalAccessConfigArgsBuilder.() -> Unit) {
val toBeMapped = PrivateClusterMasterGlobalAccessConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.masterGlobalAccessConfig = mapped
}
/**
* @param value The IP range in CIDR notation to use for the hosted master network. This range will be used for assigning internal IP addresses to the master or set of masters, as well as the ILB VIP. This range must not overlap with any other ranges in use within the cluster's network.
*/
@JvmName("xqmqqvpcuaweuxay")
public suspend fun masterIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterIpv4CidrBlock = mapped
}
/**
* @param value Subnet to provision the master's private endpoint during cluster creation. Specified in projects/*/regions/*/subnetworks/* format.
* */*/*/
*/
@JvmName("huhdclkfnawrasnh")
public suspend fun privateEndpointSubnetwork(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEndpointSubnetwork = mapped
}
internal fun build(): PrivateClusterConfigArgs = PrivateClusterConfigArgs(
enablePrivateEndpoint = enablePrivateEndpoint,
enablePrivateNodes = enablePrivateNodes,
masterGlobalAccessConfig = masterGlobalAccessConfig,
masterIpv4CidrBlock = masterIpv4CidrBlock,
privateEndpointSubnetwork = privateEndpointSubnetwork,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy