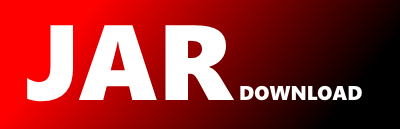
com.pulumi.googlenative.container.v1beta1.kotlin.NodePool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.container.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.MaxPodsConstraintResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeConfigResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeManagementResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeNetworkConfigResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodePoolAutoscalingResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.PlacementPolicyResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.StatusConditionResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.UpdateInfoResponse
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.UpgradeSettingsResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.MaxPodsConstraintResponse.Companion.toKotlin as maxPodsConstraintResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeConfigResponse.Companion.toKotlin as nodeConfigResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeManagementResponse.Companion.toKotlin as nodeManagementResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodeNetworkConfigResponse.Companion.toKotlin as nodeNetworkConfigResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.NodePoolAutoscalingResponse.Companion.toKotlin as nodePoolAutoscalingResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.PlacementPolicyResponse.Companion.toKotlin as placementPolicyResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.StatusConditionResponse.Companion.toKotlin as statusConditionResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.UpdateInfoResponse.Companion.toKotlin as updateInfoResponseToKotlin
import com.pulumi.googlenative.container.v1beta1.kotlin.outputs.UpgradeSettingsResponse.Companion.toKotlin as upgradeSettingsResponseToKotlin
/**
* Builder for [NodePool].
*/
@PulumiTagMarker
public class NodePoolResourceBuilder internal constructor() {
public var name: String? = null
public var args: NodePoolArgs = NodePoolArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NodePoolArgsBuilder.() -> Unit) {
val builder = NodePoolArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NodePool {
val builtJavaResource =
com.pulumi.googlenative.container.v1beta1.NodePool(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NodePool(builtJavaResource)
}
}
/**
* Creates a node pool for a cluster.
*/
public class NodePool internal constructor(
override val javaResource: com.pulumi.googlenative.container.v1beta1.NodePool,
) : KotlinCustomResource(javaResource, NodePoolMapper) {
/**
* Autoscaler configuration for this NodePool. Autoscaler is enabled only if a valid configuration is present.
*/
public val autoscaling: Output
get() = javaResource.autoscaling().applyValue({ args0 ->
args0.let({ args0 ->
nodePoolAutoscalingResponseToKotlin(args0)
})
})
public val clusterId: Output
get() = javaResource.clusterId().applyValue({ args0 -> args0 })
/**
* Which conditions caused the current node pool state.
*/
public val conditions: Output>
get() = javaResource.conditions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
statusConditionResponseToKotlin(args0)
})
})
})
/**
* The node configuration of the pool.
*/
public val config: Output
get() = javaResource.config().applyValue({ args0 ->
args0.let({ args0 ->
nodeConfigResponseToKotlin(args0)
})
})
/**
* This checksum is computed by the server based on the value of node pool fields, and may be sent on update requests to ensure the client has an up-to-date value before proceeding.
*/
public val etag: Output
get() = javaResource.etag().applyValue({ args0 -> args0 })
/**
* The initial node count for the pool. You must ensure that your Compute Engine [resource quota](https://cloud.google.com/compute/quotas) is sufficient for this number of instances. You must also have available firewall and routes quota.
*/
public val initialNodeCount: Output
get() = javaResource.initialNodeCount().applyValue({ args0 -> args0 })
/**
* [Output only] The resource URLs of the [managed instance groups](https://cloud.google.com/compute/docs/instance-groups/creating-groups-of-managed-instances) associated with this node pool. During the node pool blue-green upgrade operation, the URLs contain both blue and green resources.
*/
public val instanceGroupUrls: Output>
get() = javaResource.instanceGroupUrls().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The list of Google Compute Engine [zones](https://cloud.google.com/compute/docs/zones#available) in which the NodePool's nodes should be located. If this value is unspecified during node pool creation, the [Cluster.Locations](https://cloud.google.com/kubernetes-engine/docs/reference/rest/v1/projects.locations.clusters#Cluster.FIELDS.locations) value will be used, instead. Warning: changing node pool locations will result in nodes being added and/or removed.
*/
public val locations: Output>
get() = javaResource.locations().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* NodeManagement configuration for this NodePool.
*/
public val management: Output
get() = javaResource.management().applyValue({ args0 ->
args0.let({ args0 ->
nodeManagementResponseToKotlin(args0)
})
})
/**
* The constraint on the maximum number of pods that can be run simultaneously on a node in the node pool.
*/
public val maxPodsConstraint: Output
get() = javaResource.maxPodsConstraint().applyValue({ args0 ->
args0.let({ args0 ->
maxPodsConstraintResponseToKotlin(args0)
})
})
/**
* The name of the node pool.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Networking configuration for this NodePool. If specified, it overrides the cluster-level defaults.
*/
public val networkConfig: Output
get() = javaResource.networkConfig().applyValue({ args0 ->
args0.let({ args0 ->
nodeNetworkConfigResponseToKotlin(args0)
})
})
/**
* Specifies the node placement policy.
*/
public val placementPolicy: Output
get() = javaResource.placementPolicy().applyValue({ args0 ->
args0.let({ args0 ->
placementPolicyResponseToKotlin(args0)
})
})
/**
* [Output only] The pod CIDR block size per node in this node pool.
*/
public val podIpv4CidrSize: Output
get() = javaResource.podIpv4CidrSize().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* [Output only] Server-defined URL for the resource.
*/
public val selfLink: Output
get() = javaResource.selfLink().applyValue({ args0 -> args0 })
/**
* [Output only] The status of the nodes in this pool instance.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
/**
* [Output only] Deprecated. Use conditions instead. Additional information about the current status of this node pool instance, if available.
*/
@Deprecated(
message = """
[Output only] Deprecated. Use conditions instead. Additional information about the current status
of this node pool instance, if available.
""",
)
public val statusMessage: Output
get() = javaResource.statusMessage().applyValue({ args0 -> args0 })
/**
* [Output only] Update info contains relevant information during a node pool update.
*/
public val updateInfo: Output
get() = javaResource.updateInfo().applyValue({ args0 ->
args0.let({ args0 ->
updateInfoResponseToKotlin(args0)
})
})
/**
* Upgrade settings control disruption and speed of the upgrade.
*/
public val upgradeSettings: Output
get() = javaResource.upgradeSettings().applyValue({ args0 ->
args0.let({ args0 ->
upgradeSettingsResponseToKotlin(args0)
})
})
/**
* The version of Kubernetes running on this NodePool's nodes. If unspecified, it defaults as described [here](https://cloud.google.com/kubernetes-engine/versioning#specifying_node_version).
*/
public val version: Output
get() = javaResource.version().applyValue({ args0 -> args0 })
}
public object NodePoolMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.container.v1beta1.NodePool::class == javaResource::class
override fun map(javaResource: Resource): NodePool = NodePool(
javaResource as
com.pulumi.googlenative.container.v1beta1.NodePool,
)
}
/**
* @see [NodePool].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [NodePool].
*/
public suspend fun nodePool(name: String, block: suspend NodePoolResourceBuilder.() -> Unit): NodePool {
val builder = NodePoolResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [NodePool].
* @param name The _unique_ name of the resulting resource.
*/
public fun nodePool(name: String): NodePool {
val builder = NodePoolResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy