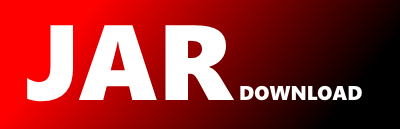
com.pulumi.googlenative.containeranalysis.v1.kotlin.inputs.DetailArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1.inputs.DetailArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A detail for a distro and package affected by this vulnerability and its associated fix (if one is available).
* @property affectedCpeUri The [CPE URI](https://cpe.mitre.org/specification/) this vulnerability affects.
* @property affectedPackage The package this vulnerability affects.
* @property affectedVersionEnd The version number at the end of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
* @property affectedVersionStart The version number at the start of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
* @property description A vendor-specific description of this vulnerability.
* @property fixedCpeUri The distro recommended [CPE URI](https://cpe.mitre.org/specification/) to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_cpe_uri.
* @property fixedPackage The distro recommended package to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_package.
* @property fixedVersion The distro recommended version to update to that contains a fix for this vulnerability. Setting this to VersionKind.MAXIMUM means no such version is yet available.
* @property isObsolete Whether this detail is obsolete. Occurrences are expected not to point to obsolete details.
* @property packageType The type of package; whether native or non native (e.g., ruby gems, node.js packages, etc.).
* @property severityName The distro assigned severity of this vulnerability.
* @property source The source from which the information in this Detail was obtained.
* @property sourceUpdateTime The time this information was last changed at the source. This is an upstream timestamp from the underlying information source - e.g. Ubuntu security tracker.
* @property vendor The name of the vendor of the product.
*/
public data class DetailArgs(
public val affectedCpeUri: Output,
public val affectedPackage: Output,
public val affectedVersionEnd: Output? = null,
public val affectedVersionStart: Output? = null,
public val description: Output? = null,
public val fixedCpeUri: Output? = null,
public val fixedPackage: Output? = null,
public val fixedVersion: Output? = null,
public val isObsolete: Output? = null,
public val packageType: Output? = null,
public val severityName: Output? = null,
public val source: Output? = null,
public val sourceUpdateTime: Output? = null,
public val vendor: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1.inputs.DetailArgs =
com.pulumi.googlenative.containeranalysis.v1.inputs.DetailArgs.builder()
.affectedCpeUri(affectedCpeUri.applyValue({ args0 -> args0 }))
.affectedPackage(affectedPackage.applyValue({ args0 -> args0 }))
.affectedVersionEnd(
affectedVersionEnd?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.affectedVersionStart(
affectedVersionStart?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.fixedCpeUri(fixedCpeUri?.applyValue({ args0 -> args0 }))
.fixedPackage(fixedPackage?.applyValue({ args0 -> args0 }))
.fixedVersion(fixedVersion?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.isObsolete(isObsolete?.applyValue({ args0 -> args0 }))
.packageType(packageType?.applyValue({ args0 -> args0 }))
.severityName(severityName?.applyValue({ args0 -> args0 }))
.source(source?.applyValue({ args0 -> args0 }))
.sourceUpdateTime(sourceUpdateTime?.applyValue({ args0 -> args0 }))
.vendor(vendor?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DetailArgs].
*/
@PulumiTagMarker
public class DetailArgsBuilder internal constructor() {
private var affectedCpeUri: Output? = null
private var affectedPackage: Output? = null
private var affectedVersionEnd: Output? = null
private var affectedVersionStart: Output? = null
private var description: Output? = null
private var fixedCpeUri: Output? = null
private var fixedPackage: Output? = null
private var fixedVersion: Output? = null
private var isObsolete: Output? = null
private var packageType: Output? = null
private var severityName: Output? = null
private var source: Output? = null
private var sourceUpdateTime: Output? = null
private var vendor: Output? = null
/**
* @param value The [CPE URI](https://cpe.mitre.org/specification/) this vulnerability affects.
*/
@JvmName("qsvxqpjycbmvbqlq")
public suspend fun affectedCpeUri(`value`: Output) {
this.affectedCpeUri = value
}
/**
* @param value The package this vulnerability affects.
*/
@JvmName("eepyumcvvqqdsjva")
public suspend fun affectedPackage(`value`: Output) {
this.affectedPackage = value
}
/**
* @param value The version number at the end of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("vncmdmyjdashhdps")
public suspend fun affectedVersionEnd(`value`: Output) {
this.affectedVersionEnd = value
}
/**
* @param value The version number at the start of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("xuousbkdevuiekbq")
public suspend fun affectedVersionStart(`value`: Output) {
this.affectedVersionStart = value
}
/**
* @param value A vendor-specific description of this vulnerability.
*/
@JvmName("msqfxjdejgvpwkwn")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The distro recommended [CPE URI](https://cpe.mitre.org/specification/) to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_cpe_uri.
*/
@JvmName("aqiirgoohsejxlkb")
public suspend fun fixedCpeUri(`value`: Output) {
this.fixedCpeUri = value
}
/**
* @param value The distro recommended package to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_package.
*/
@JvmName("ceclqswkxihuxglc")
public suspend fun fixedPackage(`value`: Output) {
this.fixedPackage = value
}
/**
* @param value The distro recommended version to update to that contains a fix for this vulnerability. Setting this to VersionKind.MAXIMUM means no such version is yet available.
*/
@JvmName("syaangkfmmdnmppi")
public suspend fun fixedVersion(`value`: Output) {
this.fixedVersion = value
}
/**
* @param value Whether this detail is obsolete. Occurrences are expected not to point to obsolete details.
*/
@JvmName("hoxftgrrnaswehmk")
public suspend fun isObsolete(`value`: Output) {
this.isObsolete = value
}
/**
* @param value The type of package; whether native or non native (e.g., ruby gems, node.js packages, etc.).
*/
@JvmName("arpfsfuitdldqmmb")
public suspend fun packageType(`value`: Output) {
this.packageType = value
}
/**
* @param value The distro assigned severity of this vulnerability.
*/
@JvmName("vbynqnmhghdwagcb")
public suspend fun severityName(`value`: Output) {
this.severityName = value
}
/**
* @param value The source from which the information in this Detail was obtained.
*/
@JvmName("xlqxewkqkpthxtdl")
public suspend fun source(`value`: Output) {
this.source = value
}
/**
* @param value The time this information was last changed at the source. This is an upstream timestamp from the underlying information source - e.g. Ubuntu security tracker.
*/
@JvmName("qlpsjyllgalywdjk")
public suspend fun sourceUpdateTime(`value`: Output) {
this.sourceUpdateTime = value
}
/**
* @param value The name of the vendor of the product.
*/
@JvmName("yfiprpywlxtofhpc")
public suspend fun vendor(`value`: Output) {
this.vendor = value
}
/**
* @param value The [CPE URI](https://cpe.mitre.org/specification/) this vulnerability affects.
*/
@JvmName("rpecvcfblngwjgps")
public suspend fun affectedCpeUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.affectedCpeUri = mapped
}
/**
* @param value The package this vulnerability affects.
*/
@JvmName("ocuxdqhtqrcohnoi")
public suspend fun affectedPackage(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.affectedPackage = mapped
}
/**
* @param value The version number at the end of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("sojvbjfrrerkpfkf")
public suspend fun affectedVersionEnd(`value`: VersionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.affectedVersionEnd = mapped
}
/**
* @param argument The version number at the end of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("pashdmbpuxlquumo")
public suspend fun affectedVersionEnd(argument: suspend VersionArgsBuilder.() -> Unit) {
val toBeMapped = VersionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.affectedVersionEnd = mapped
}
/**
* @param value The version number at the start of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("qwqvstiucnpwllkg")
public suspend fun affectedVersionStart(`value`: VersionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.affectedVersionStart = mapped
}
/**
* @param argument The version number at the start of an interval in which this vulnerability exists. A vulnerability can affect a package between version numbers that are disjoint sets of intervals (example: [1.0.0-1.1.0], [2.4.6-2.4.8] and [4.5.6-4.6.8]) each of which will be represented in its own Detail. If a specific affected version is provided by a vulnerability database, affected_version_start and affected_version_end will be the same in that Detail.
*/
@JvmName("iobapnrexaubauig")
public suspend fun affectedVersionStart(argument: suspend VersionArgsBuilder.() -> Unit) {
val toBeMapped = VersionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.affectedVersionStart = mapped
}
/**
* @param value A vendor-specific description of this vulnerability.
*/
@JvmName("ktmnphxuuilxlnrr")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The distro recommended [CPE URI](https://cpe.mitre.org/specification/) to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_cpe_uri.
*/
@JvmName("yafmcqrdxeqmwpbd")
public suspend fun fixedCpeUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fixedCpeUri = mapped
}
/**
* @param value The distro recommended package to update to that contains a fix for this vulnerability. It is possible for this to be different from the affected_package.
*/
@JvmName("hgtphhrksbfrensi")
public suspend fun fixedPackage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fixedPackage = mapped
}
/**
* @param value The distro recommended version to update to that contains a fix for this vulnerability. Setting this to VersionKind.MAXIMUM means no such version is yet available.
*/
@JvmName("nviabggyqvrbglrq")
public suspend fun fixedVersion(`value`: VersionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fixedVersion = mapped
}
/**
* @param argument The distro recommended version to update to that contains a fix for this vulnerability. Setting this to VersionKind.MAXIMUM means no such version is yet available.
*/
@JvmName("ybktwphlkbpvpmxl")
public suspend fun fixedVersion(argument: suspend VersionArgsBuilder.() -> Unit) {
val toBeMapped = VersionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fixedVersion = mapped
}
/**
* @param value Whether this detail is obsolete. Occurrences are expected not to point to obsolete details.
*/
@JvmName("qlgitcnyrkldxiny")
public suspend fun isObsolete(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isObsolete = mapped
}
/**
* @param value The type of package; whether native or non native (e.g., ruby gems, node.js packages, etc.).
*/
@JvmName("nfpywwlypkneyauq")
public suspend fun packageType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.packageType = mapped
}
/**
* @param value The distro assigned severity of this vulnerability.
*/
@JvmName("bpyjsubpcxbmqxvq")
public suspend fun severityName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.severityName = mapped
}
/**
* @param value The source from which the information in this Detail was obtained.
*/
@JvmName("vmtotagedwfxhnbq")
public suspend fun source(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.source = mapped
}
/**
* @param value The time this information was last changed at the source. This is an upstream timestamp from the underlying information source - e.g. Ubuntu security tracker.
*/
@JvmName("nmjfqepegegoygmq")
public suspend fun sourceUpdateTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceUpdateTime = mapped
}
/**
* @param value The name of the vendor of the product.
*/
@JvmName("bqxnvmtldywddjwp")
public suspend fun vendor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vendor = mapped
}
internal fun build(): DetailArgs = DetailArgs(
affectedCpeUri = affectedCpeUri ?: throw PulumiNullFieldException("affectedCpeUri"),
affectedPackage = affectedPackage ?: throw PulumiNullFieldException("affectedPackage"),
affectedVersionEnd = affectedVersionEnd,
affectedVersionStart = affectedVersionStart,
description = description,
fixedCpeUri = fixedCpeUri,
fixedPackage = fixedPackage,
fixedVersion = fixedVersion,
isObsolete = isObsolete,
packageType = packageType,
severityName = severityName,
source = source,
sourceUpdateTime = sourceUpdateTime,
vendor = vendor,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy