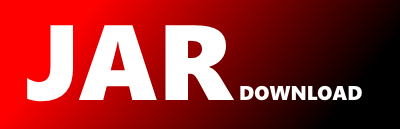
com.pulumi.googlenative.containeranalysis.v1.kotlin.inputs.DiscoveryOccurrenceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1.inputs.DiscoveryOccurrenceArgs.builder
import com.pulumi.googlenative.containeranalysis.v1.kotlin.enums.DiscoveryOccurrenceAnalysisStatus
import com.pulumi.googlenative.containeranalysis.v1.kotlin.enums.DiscoveryOccurrenceContinuousAnalysis
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides information about the analysis status of a discovered resource.
* @property analysisCompleted
* @property analysisError Indicates any errors encountered during analysis of a resource. There could be 0 or more of these errors.
* @property analysisStatus The status of discovery for the resource.
* @property analysisStatusError When an error is encountered this will contain a LocalizedMessage under details to show to the user. The LocalizedMessage is output only and populated by the API.
* @property continuousAnalysis Whether the resource is continuously analyzed.
* @property cpe The CPE of the resource being scanned.
* @property lastScanTime The last time this resource was scanned.
*/
public data class DiscoveryOccurrenceArgs(
public val analysisCompleted: Output? = null,
public val analysisError: Output>? = null,
public val analysisStatus: Output? = null,
public val analysisStatusError: Output? = null,
public val continuousAnalysis: Output? = null,
public val cpe: Output? = null,
public val lastScanTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1.inputs.DiscoveryOccurrenceArgs = com.pulumi.googlenative.containeranalysis.v1.inputs.DiscoveryOccurrenceArgs.builder()
.analysisCompleted(analysisCompleted?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.analysisError(
analysisError?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.analysisStatus(analysisStatus?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.analysisStatusError(
analysisStatusError?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.continuousAnalysis(
continuousAnalysis?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.cpe(cpe?.applyValue({ args0 -> args0 }))
.lastScanTime(lastScanTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DiscoveryOccurrenceArgs].
*/
@PulumiTagMarker
public class DiscoveryOccurrenceArgsBuilder internal constructor() {
private var analysisCompleted: Output? = null
private var analysisError: Output>? = null
private var analysisStatus: Output? = null
private var analysisStatusError: Output? = null
private var continuousAnalysis: Output? = null
private var cpe: Output? = null
private var lastScanTime: Output? = null
/**
* @param value
*/
@JvmName("yjpvnmmwxrjuqjek")
public suspend fun analysisCompleted(`value`: Output) {
this.analysisCompleted = value
}
/**
* @param value Indicates any errors encountered during analysis of a resource. There could be 0 or more of these errors.
*/
@JvmName("fogcfgabpmjlqayf")
public suspend fun analysisError(`value`: Output>) {
this.analysisError = value
}
@JvmName("umvbssoarlammmrv")
public suspend fun analysisError(vararg values: Output) {
this.analysisError = Output.all(values.asList())
}
/**
* @param values Indicates any errors encountered during analysis of a resource. There could be 0 or more of these errors.
*/
@JvmName("eenagssvhgnmfhpo")
public suspend fun analysisError(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy