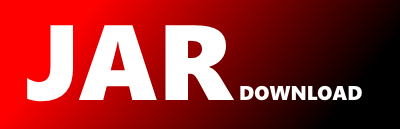
com.pulumi.googlenative.containeranalysis.v1alpha1.kotlin.inputs.GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Other properties of the build.
* @property buildFinishedOn The timestamp of when the build completed.
* @property buildInvocationId Identifies this particular build invocation, which can be useful for finding associated logs or other ad-hoc analysis.
* @property buildStartedOn The timestamp of when the build started.
* @property completeness Indicates that the builder claims certain fields in this message to be complete.
* @property reproducible If true, the builder claims that running invocation on materials will produce bit-for-bit identical output.
*/
public data class GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs(
public val buildFinishedOn: Output? = null,
public val buildInvocationId: Output? = null,
public val buildStartedOn: Output? = null,
public val completeness: Output? =
null,
public val reproducible: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs =
com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs.builder()
.buildFinishedOn(buildFinishedOn?.applyValue({ args0 -> args0 }))
.buildInvocationId(buildInvocationId?.applyValue({ args0 -> args0 }))
.buildStartedOn(buildStartedOn?.applyValue({ args0 -> args0 }))
.completeness(completeness?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.reproducible(reproducible?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs].
*/
@PulumiTagMarker
public class GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgsBuilder
internal constructor() {
private var buildFinishedOn: Output? = null
private var buildInvocationId: Output? = null
private var buildStartedOn: Output? = null
private var completeness:
Output? =
null
private var reproducible: Output? = null
/**
* @param value The timestamp of when the build completed.
*/
@JvmName("lqmjwfvwtoaawemr")
public suspend fun buildFinishedOn(`value`: Output) {
this.buildFinishedOn = value
}
/**
* @param value Identifies this particular build invocation, which can be useful for finding associated logs or other ad-hoc analysis.
*/
@JvmName("ugbcoplimplcdopq")
public suspend fun buildInvocationId(`value`: Output) {
this.buildInvocationId = value
}
/**
* @param value The timestamp of when the build started.
*/
@JvmName("rbwfpchccctxuojt")
public suspend fun buildStartedOn(`value`: Output) {
this.buildStartedOn = value
}
/**
* @param value Indicates that the builder claims certain fields in this message to be complete.
*/
@JvmName("dqckovtufedbgldr")
public suspend fun completeness(`value`: Output) {
this.completeness = value
}
/**
* @param value If true, the builder claims that running invocation on materials will produce bit-for-bit identical output.
*/
@JvmName("wdvsxoquocbvgxem")
public suspend fun reproducible(`value`: Output) {
this.reproducible = value
}
/**
* @param value The timestamp of when the build completed.
*/
@JvmName("urclmmjypeseaemv")
public suspend fun buildFinishedOn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildFinishedOn = mapped
}
/**
* @param value Identifies this particular build invocation, which can be useful for finding associated logs or other ad-hoc analysis.
*/
@JvmName("uhqdthfgkjbxmtpj")
public suspend fun buildInvocationId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildInvocationId = mapped
}
/**
* @param value The timestamp of when the build started.
*/
@JvmName("unoxfkgammhnlflw")
public suspend fun buildStartedOn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildStartedOn = mapped
}
/**
* @param value Indicates that the builder claims certain fields in this message to be complete.
*/
@JvmName("ronkvsqmvtbfuade")
public suspend fun completeness(`value`: GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaCompletenessArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.completeness = mapped
}
/**
* @param argument Indicates that the builder claims certain fields in this message to be complete.
*/
@JvmName("mobmshfkfwmqlhht")
public suspend fun completeness(argument: suspend GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaCompletenessArgsBuilder.() -> Unit) {
val toBeMapped =
GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaCompletenessArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.completeness = mapped
}
/**
* @param value If true, the builder claims that running invocation on materials will produce bit-for-bit identical output.
*/
@JvmName("qltaictinexkaput")
public suspend fun reproducible(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reproducible = mapped
}
internal fun build(): GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs = GoogleDevtoolsContaineranalysisV1alpha1SlsaProvenanceZeroTwoSlsaMetadataArgs(
buildFinishedOn = buildFinishedOn,
buildInvocationId = buildInvocationId,
buildStartedOn = buildStartedOn,
completeness = completeness,
reproducible = reproducible,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy