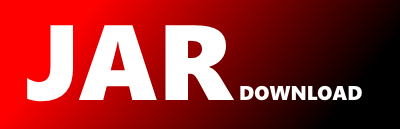
com.pulumi.googlenative.containeranalysis.v1alpha1.kotlin.inputs.VulnerabilityDetailsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.VulnerabilityDetailsArgs.builder
import com.pulumi.googlenative.containeranalysis.v1alpha1.kotlin.enums.VulnerabilityDetailsEffectiveSeverity
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Used by Occurrence to point to where the vulnerability exists and how to fix it.
* @property cvssV2 The CVSS v2 score of this vulnerability.
* @property cvssV3 The CVSS v3 score of this vulnerability.
* @property effectiveSeverity The distro assigned severity for this vulnerability when that is available and note provider assigned severity when distro has not yet assigned a severity for this vulnerability. When there are multiple package issues for this vulnerability, they can have different effective severities because some might come from the distro and some might come from installed language packs (e.g. Maven JARs or Go binaries). For this reason, it is advised to use the effective severity on the PackageIssue level, as this field may eventually be deprecated. In the case where multiple PackageIssues have different effective severities, the one set here will be the highest severity of any of the PackageIssues.
* @property packageIssue The set of affected locations and their fixes (if available) within the associated resource.
* @property type The type of package; whether native or non native(ruby gems, node.js packages etc). This may be deprecated in the future because we can have multiple PackageIssues with different package types.
* @property vexAssessment VexAssessment provides all publisher provided Vex information that is related to this vulnerability for this resource.
*/
public data class VulnerabilityDetailsArgs(
public val cvssV2: Output? = null,
public val cvssV3: Output? = null,
public val effectiveSeverity: Output? = null,
public val packageIssue: Output>? = null,
public val type: Output? = null,
public val vexAssessment: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.VulnerabilityDetailsArgs =
com.pulumi.googlenative.containeranalysis.v1alpha1.inputs.VulnerabilityDetailsArgs.builder()
.cvssV2(cvssV2?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cvssV3(cvssV3?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.effectiveSeverity(effectiveSeverity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.packageIssue(
packageIssue?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.type(type?.applyValue({ args0 -> args0 }))
.vexAssessment(vexAssessment?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [VulnerabilityDetailsArgs].
*/
@PulumiTagMarker
public class VulnerabilityDetailsArgsBuilder internal constructor() {
private var cvssV2: Output? = null
private var cvssV3: Output? = null
private var effectiveSeverity: Output? = null
private var packageIssue: Output>? = null
private var type: Output? = null
private var vexAssessment: Output? = null
/**
* @param value The CVSS v2 score of this vulnerability.
*/
@JvmName("yggebwrwjbqqveyy")
public suspend fun cvssV2(`value`: Output) {
this.cvssV2 = value
}
/**
* @param value The CVSS v3 score of this vulnerability.
*/
@JvmName("aqlenajgcboxwsqj")
public suspend fun cvssV3(`value`: Output) {
this.cvssV3 = value
}
/**
* @param value The distro assigned severity for this vulnerability when that is available and note provider assigned severity when distro has not yet assigned a severity for this vulnerability. When there are multiple package issues for this vulnerability, they can have different effective severities because some might come from the distro and some might come from installed language packs (e.g. Maven JARs or Go binaries). For this reason, it is advised to use the effective severity on the PackageIssue level, as this field may eventually be deprecated. In the case where multiple PackageIssues have different effective severities, the one set here will be the highest severity of any of the PackageIssues.
*/
@JvmName("ljwugcoycfwfnuin")
public suspend fun effectiveSeverity(`value`: Output) {
this.effectiveSeverity = value
}
/**
* @param value The set of affected locations and their fixes (if available) within the associated resource.
*/
@JvmName("aftkuiutybcfiymj")
public suspend fun packageIssue(`value`: Output>) {
this.packageIssue = value
}
@JvmName("cvygtmuypukpdulp")
public suspend fun packageIssue(vararg values: Output) {
this.packageIssue = Output.all(values.asList())
}
/**
* @param values The set of affected locations and their fixes (if available) within the associated resource.
*/
@JvmName("maatwrxsmjeduapn")
public suspend fun packageIssue(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy