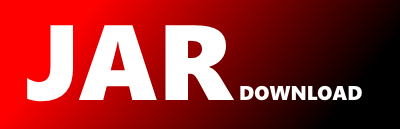
com.pulumi.googlenative.containeranalysis.v1beta1.kotlin.inputs.DocumentOccurrenceArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1beta1.inputs.DocumentOccurrenceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* DocumentOccurrence represents an SPDX Document Creation Information section: https://spdx.github.io/spdx-spec/v2.3/document-creation-information/
* @property createTime Identify when the SPDX file was originally created. The date is to be specified according to combined date and time in UTC format as specified in ISO 8601 standard
* @property creatorComment A field for creators of the SPDX file to provide general comments about the creation of the SPDX file or any other relevant comment not included in the other fields
* @property creators Identify who (or what, in the case of a tool) created the SPDX file. If the SPDX file was created by an individual, indicate the person's name
* @property documentComment A field for creators of the SPDX file content to provide comments to the consumers of the SPDX document
* @property externalDocumentRefs Identify any external SPDX documents referenced within this SPDX document
* @property id Identify the current SPDX document which may be referenced in relationships by other files, packages internally and documents externally
* @property licenseListVersion A field for creators of the SPDX file to provide the version of the SPDX License List used when the SPDX file was created
* @property namespace Provide an SPDX document specific namespace as a unique absolute Uniform Resource Identifier (URI) as specified in RFC-3986, with the exception of the ‘#’ delimiter
* @property title Identify name of this document as designated by creator
*/
public data class DocumentOccurrenceArgs(
public val createTime: Output? = null,
public val creatorComment: Output? = null,
public val creators: Output>? = null,
public val documentComment: Output? = null,
public val externalDocumentRefs: Output>? = null,
public val id: Output? = null,
public val licenseListVersion: Output? = null,
public val namespace: Output? = null,
public val title: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1beta1.inputs.DocumentOccurrenceArgs =
com.pulumi.googlenative.containeranalysis.v1beta1.inputs.DocumentOccurrenceArgs.builder()
.createTime(createTime?.applyValue({ args0 -> args0 }))
.creatorComment(creatorComment?.applyValue({ args0 -> args0 }))
.creators(creators?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.documentComment(documentComment?.applyValue({ args0 -> args0 }))
.externalDocumentRefs(externalDocumentRefs?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.id(id?.applyValue({ args0 -> args0 }))
.licenseListVersion(licenseListVersion?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.title(title?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DocumentOccurrenceArgs].
*/
@PulumiTagMarker
public class DocumentOccurrenceArgsBuilder internal constructor() {
private var createTime: Output? = null
private var creatorComment: Output? = null
private var creators: Output>? = null
private var documentComment: Output? = null
private var externalDocumentRefs: Output>? = null
private var id: Output? = null
private var licenseListVersion: Output? = null
private var namespace: Output? = null
private var title: Output? = null
/**
* @param value Identify when the SPDX file was originally created. The date is to be specified according to combined date and time in UTC format as specified in ISO 8601 standard
*/
@JvmName("goltfmtdcqqupvvk")
public suspend fun createTime(`value`: Output) {
this.createTime = value
}
/**
* @param value A field for creators of the SPDX file to provide general comments about the creation of the SPDX file or any other relevant comment not included in the other fields
*/
@JvmName("turvlwapxvapspfy")
public suspend fun creatorComment(`value`: Output) {
this.creatorComment = value
}
/**
* @param value Identify who (or what, in the case of a tool) created the SPDX file. If the SPDX file was created by an individual, indicate the person's name
*/
@JvmName("igllkdxtkffkyogo")
public suspend fun creators(`value`: Output>) {
this.creators = value
}
@JvmName("ixhrxwnhqwaefqcj")
public suspend fun creators(vararg values: Output) {
this.creators = Output.all(values.asList())
}
/**
* @param values Identify who (or what, in the case of a tool) created the SPDX file. If the SPDX file was created by an individual, indicate the person's name
*/
@JvmName("sjgtrmbypmyxqaxg")
public suspend fun creators(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy