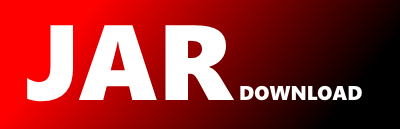
com.pulumi.googlenative.containeranalysis.v1beta1.kotlin.inputs.SbomReferenceIntotoPayloadArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.containeranalysis.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.containeranalysis.v1beta1.inputs.SbomReferenceIntotoPayloadArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The actual payload that contains the SBOM Reference data. The payload follows the intoto statement specification. See https://github.com/in-toto/attestation/blob/main/spec/v1.0/statement.md for more details.
* @property predicate Additional parameters of the Predicate. Includes the actual data about the SBOM.
* @property predicateType URI identifying the type of the Predicate.
* @property subject Set of software artifacts that the attestation applies to. Each element represents a single software artifact.
* @property type Identifier for the schema of the Statement.
*/
public data class SbomReferenceIntotoPayloadArgs(
public val predicate: Output? = null,
public val predicateType: Output? = null,
public val subject: Output>? = null,
public val type: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.containeranalysis.v1beta1.inputs.SbomReferenceIntotoPayloadArgs =
com.pulumi.googlenative.containeranalysis.v1beta1.inputs.SbomReferenceIntotoPayloadArgs.builder()
.predicate(predicate?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.predicateType(predicateType?.applyValue({ args0 -> args0 }))
.subject(
subject?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SbomReferenceIntotoPayloadArgs].
*/
@PulumiTagMarker
public class SbomReferenceIntotoPayloadArgsBuilder internal constructor() {
private var predicate: Output? = null
private var predicateType: Output? = null
private var subject: Output>? = null
private var type: Output? = null
/**
* @param value Additional parameters of the Predicate. Includes the actual data about the SBOM.
*/
@JvmName("oybnsyvoxbymjuip")
public suspend fun predicate(`value`: Output) {
this.predicate = value
}
/**
* @param value URI identifying the type of the Predicate.
*/
@JvmName("uwaerjrojpqfronn")
public suspend fun predicateType(`value`: Output) {
this.predicateType = value
}
/**
* @param value Set of software artifacts that the attestation applies to. Each element represents a single software artifact.
*/
@JvmName("dnbcprkqtpetxhii")
public suspend fun subject(`value`: Output>) {
this.subject = value
}
@JvmName("tbshgahstitsqqvt")
public suspend fun subject(vararg values: Output) {
this.subject = Output.all(values.asList())
}
/**
* @param values Set of software artifacts that the attestation applies to. Each element represents a single software artifact.
*/
@JvmName("mmfhbiwtrjuxytqx")
public suspend fun subject(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy