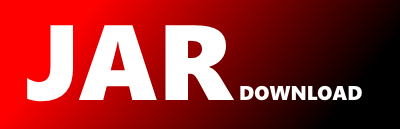
com.pulumi.googlenative.contentwarehouse.v1.kotlin.DocumentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.contentwarehouse.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.contentwarehouse.v1.DocumentArgs.builder
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.enums.DocumentContentCategory
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.enums.DocumentRawDocumentFileType
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1CloudAIDocumentOptionArgs
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1CloudAIDocumentOptionArgsBuilder
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1PropertyArgs
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1PropertyArgsBuilder
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1RequestMetadataArgs
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudContentwarehouseV1RequestMetadataArgsBuilder
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudDocumentaiV1DocumentArgs
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudDocumentaiV1DocumentArgsBuilder
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleIamV1PolicyArgs
import com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleIamV1PolicyArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Creates a document.
* @property cloudAiDocument Document AI format to save the structured content, including OCR.
* @property cloudAiDocumentOption Request Option for processing Cloud AI Document in Document Warehouse. This field offers limited support for mapping entities from Cloud AI Document to Warehouse Document. Please consult with product team before using this field and other available options.
* @property contentCategory Indicates the category (image, audio, video etc.) of the original content.
* @property createMask Field mask for creating Document fields. If mask path is empty, it means all fields are masked. For the `FieldMask` definition, see https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask.
* @property creator The user who creates the document.
* @property displayName Display name of the document given by the user. This name will be displayed in the UI. Customer can populate this field with the name of the document. This differs from the 'title' field as 'title' is optional and stores the top heading in the document.
* @property displayUri Uri to display the document, for example, in the UI.
* @property documentSchemaName The Document schema name. Format: projects/{project_number}/locations/{location}/documentSchemas/{document_schema_id}.
* @property inlineRawDocument Raw document content.
* @property location
* @property name The resource name of the document. Format: projects/{project_number}/locations/{location}/documents/{document_id}. The name is ignored when creating a document.
* @property plainText Other document format, such as PPTX, XLXS
* @property policy Default document policy during creation. This refers to an Identity and Access (IAM) policy, which specifies access controls for the Document. Conditions defined in the policy will be ignored.
* @property project
* @property properties List of values that are user supplied metadata.
* @property rawDocumentFileType This is used when DocAI was not used to load the document and parsing/ extracting is needed for the inline_raw_document. For example, if inline_raw_document is the byte representation of a PDF file, then this should be set to: RAW_DOCUMENT_FILE_TYPE_PDF.
* @property rawDocumentPath Raw document file in Cloud Storage path.
* @property referenceId The reference ID set by customers. Must be unique per project and location.
* @property requestMetadata The meta information collected about the end user, used to enforce access control for the service.
* @property textExtractionDisabled If true, text extraction will not be performed.
* @property textExtractionEnabled If true, text extraction will be performed.
* @property title Title that describes the document. This can be the top heading or text that describes the document.
* @property updater The user who lastly updates the document.
*/
public data class DocumentArgs(
public val cloudAiDocument: Output? = null,
public val cloudAiDocumentOption: Output? =
null,
public val contentCategory: Output? = null,
public val createMask: Output? = null,
public val creator: Output? = null,
public val displayName: Output? = null,
public val displayUri: Output? = null,
public val documentSchemaName: Output? = null,
public val inlineRawDocument: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val plainText: Output? = null,
public val policy: Output? = null,
public val project: Output? = null,
public val properties: Output>? = null,
public val rawDocumentFileType: Output? = null,
public val rawDocumentPath: Output? = null,
public val referenceId: Output? = null,
public val requestMetadata: Output? = null,
public val textExtractionDisabled: Output? = null,
public val textExtractionEnabled: Output? = null,
public val title: Output? = null,
public val updater: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.contentwarehouse.v1.DocumentArgs =
com.pulumi.googlenative.contentwarehouse.v1.DocumentArgs.builder()
.cloudAiDocument(cloudAiDocument?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.cloudAiDocumentOption(
cloudAiDocumentOption?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.contentCategory(contentCategory?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.createMask(createMask?.applyValue({ args0 -> args0 }))
.creator(creator?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.displayUri(displayUri?.applyValue({ args0 -> args0 }))
.documentSchemaName(documentSchemaName?.applyValue({ args0 -> args0 }))
.inlineRawDocument(inlineRawDocument?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.plainText(plainText?.applyValue({ args0 -> args0 }))
.policy(policy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.project(project?.applyValue({ args0 -> args0 }))
.properties(
properties?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.rawDocumentFileType(
rawDocumentFileType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rawDocumentPath(rawDocumentPath?.applyValue({ args0 -> args0 }))
.referenceId(referenceId?.applyValue({ args0 -> args0 }))
.requestMetadata(requestMetadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.textExtractionDisabled(textExtractionDisabled?.applyValue({ args0 -> args0 }))
.textExtractionEnabled(textExtractionEnabled?.applyValue({ args0 -> args0 }))
.title(title?.applyValue({ args0 -> args0 }))
.updater(updater?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DocumentArgs].
*/
@PulumiTagMarker
public class DocumentArgsBuilder internal constructor() {
private var cloudAiDocument: Output? = null
private var cloudAiDocumentOption: Output? =
null
private var contentCategory: Output? = null
private var createMask: Output? = null
private var creator: Output? = null
private var displayName: Output? = null
private var displayUri: Output? = null
private var documentSchemaName: Output? = null
private var inlineRawDocument: Output? = null
private var location: Output? = null
private var name: Output? = null
private var plainText: Output? = null
private var policy: Output? = null
private var project: Output? = null
private var properties: Output>? = null
private var rawDocumentFileType: Output? = null
private var rawDocumentPath: Output? = null
private var referenceId: Output? = null
private var requestMetadata: Output? = null
private var textExtractionDisabled: Output? = null
private var textExtractionEnabled: Output? = null
private var title: Output? = null
private var updater: Output? = null
/**
* @param value Document AI format to save the structured content, including OCR.
*/
@JvmName("elhfvbkwfycpfefl")
public suspend fun cloudAiDocument(`value`: Output) {
this.cloudAiDocument = value
}
/**
* @param value Request Option for processing Cloud AI Document in Document Warehouse. This field offers limited support for mapping entities from Cloud AI Document to Warehouse Document. Please consult with product team before using this field and other available options.
*/
@JvmName("equxkmkarqfvoqwn")
public suspend fun cloudAiDocumentOption(`value`: Output) {
this.cloudAiDocumentOption = value
}
/**
* @param value Indicates the category (image, audio, video etc.) of the original content.
*/
@JvmName("uivonjhxnjphoagy")
public suspend fun contentCategory(`value`: Output) {
this.contentCategory = value
}
/**
* @param value Field mask for creating Document fields. If mask path is empty, it means all fields are masked. For the `FieldMask` definition, see https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask.
*/
@JvmName("mfsgpjkbnckenwjl")
public suspend fun createMask(`value`: Output) {
this.createMask = value
}
/**
* @param value The user who creates the document.
*/
@JvmName("iaebbvxonrlmmjvh")
public suspend fun creator(`value`: Output) {
this.creator = value
}
/**
* @param value Display name of the document given by the user. This name will be displayed in the UI. Customer can populate this field with the name of the document. This differs from the 'title' field as 'title' is optional and stores the top heading in the document.
*/
@JvmName("hnepadueyypumrtn")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Uri to display the document, for example, in the UI.
*/
@JvmName("tcwgwcypkoinnugv")
public suspend fun displayUri(`value`: Output) {
this.displayUri = value
}
/**
* @param value The Document schema name. Format: projects/{project_number}/locations/{location}/documentSchemas/{document_schema_id}.
*/
@JvmName("wggbtsxvvgjvwdnm")
public suspend fun documentSchemaName(`value`: Output) {
this.documentSchemaName = value
}
/**
* @param value Raw document content.
*/
@JvmName("tvbxwqvvvaeqfkss")
public suspend fun inlineRawDocument(`value`: Output) {
this.inlineRawDocument = value
}
/**
* @param value
*/
@JvmName("tdkinbaaauqoeroa")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The resource name of the document. Format: projects/{project_number}/locations/{location}/documents/{document_id}. The name is ignored when creating a document.
*/
@JvmName("sdcxhvwgkyivtqlb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Other document format, such as PPTX, XLXS
*/
@JvmName("hgojuroiiubcektg")
public suspend fun plainText(`value`: Output) {
this.plainText = value
}
/**
* @param value Default document policy during creation. This refers to an Identity and Access (IAM) policy, which specifies access controls for the Document. Conditions defined in the policy will be ignored.
*/
@JvmName("gpemssetqkfeyqxv")
public suspend fun policy(`value`: Output) {
this.policy = value
}
/**
* @param value
*/
@JvmName("pxevnsjhpyrphuia")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value List of values that are user supplied metadata.
*/
@JvmName("hevhxyolwqpbdqlp")
public suspend fun properties(`value`: Output>) {
this.properties = value
}
@JvmName("tqtpfaorlsbdbvdm")
public suspend fun properties(vararg values: Output) {
this.properties = Output.all(values.asList())
}
/**
* @param values List of values that are user supplied metadata.
*/
@JvmName("dqegvhuhrhetyymk")
public suspend fun properties(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy