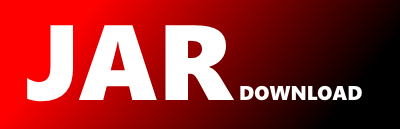
com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs.GoogleCloudDocumentaiV1DocumentPageTableArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.contentwarehouse.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.contentwarehouse.v1.inputs.GoogleCloudDocumentaiV1DocumentPageTableArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A table representation similar to HTML table structure.
* @property bodyRows Body rows of the table.
* @property detectedLanguages A list of detected languages together with confidence.
* @property headerRows Header rows of the table.
* @property layout Layout for Table.
* @property provenance The history of this table.
*/
public data class GoogleCloudDocumentaiV1DocumentPageTableArgs(
public val bodyRows: Output>? = null,
public val detectedLanguages: Output>? = null,
public val headerRows: Output>? = null,
public val layout: Output? = null,
public val provenance: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.contentwarehouse.v1.inputs.GoogleCloudDocumentaiV1DocumentPageTableArgs =
com.pulumi.googlenative.contentwarehouse.v1.inputs.GoogleCloudDocumentaiV1DocumentPageTableArgs.builder()
.bodyRows(
bodyRows?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.detectedLanguages(
detectedLanguages?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.headerRows(
headerRows?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.layout(layout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.provenance(provenance?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [GoogleCloudDocumentaiV1DocumentPageTableArgs].
*/
@PulumiTagMarker
public class GoogleCloudDocumentaiV1DocumentPageTableArgsBuilder internal constructor() {
private var bodyRows: Output>? = null
private var detectedLanguages:
Output>? = null
private var headerRows: Output>? = null
private var layout: Output? = null
private var provenance: Output? = null
/**
* @param value Body rows of the table.
*/
@JvmName("qpjmwlpvfigktobr")
public suspend fun bodyRows(`value`: Output>) {
this.bodyRows = value
}
@JvmName("ywgdqmemcdeuusts")
public suspend fun bodyRows(vararg values: Output) {
this.bodyRows = Output.all(values.asList())
}
/**
* @param values Body rows of the table.
*/
@JvmName("xjyeodnyjkgpknku")
public suspend fun bodyRows(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy