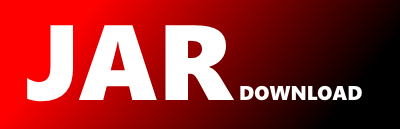
com.pulumi.googlenative.dataflow.v1b3.kotlin.Job.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataflow.v1b3.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.EnvironmentResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.ExecutionStageStateResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.JobExecutionInfoResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.JobMetadataResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.PipelineDescriptionResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.RuntimeUpdatableParamsResponse
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.StepResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.EnvironmentResponse.Companion.toKotlin as environmentResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.ExecutionStageStateResponse.Companion.toKotlin as executionStageStateResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.JobExecutionInfoResponse.Companion.toKotlin as jobExecutionInfoResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.JobMetadataResponse.Companion.toKotlin as jobMetadataResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.PipelineDescriptionResponse.Companion.toKotlin as pipelineDescriptionResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.RuntimeUpdatableParamsResponse.Companion.toKotlin as runtimeUpdatableParamsResponseToKotlin
import com.pulumi.googlenative.dataflow.v1b3.kotlin.outputs.StepResponse.Companion.toKotlin as stepResponseToKotlin
/**
* Builder for [Job].
*/
@PulumiTagMarker
public class JobResourceBuilder internal constructor() {
public var name: String? = null
public var args: JobArgs = JobArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend JobArgsBuilder.() -> Unit) {
val builder = JobArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Job {
val builtJavaResource = com.pulumi.googlenative.dataflow.v1b3.Job(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Job(builtJavaResource)
}
}
/**
* Creates a Cloud Dataflow job. To create a job, we recommend using `projects.locations.jobs.create` with a [regional endpoint] (https://cloud.google.com/dataflow/docs/concepts/regional-endpoints). Using `projects.jobs.create` is not recommended, as your job will always start in `us-central1`. Do not enter confidential information when you supply string values using the API.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
*/
public class Job internal constructor(
override val javaResource: com.pulumi.googlenative.dataflow.v1b3.Job,
) : KotlinCustomResource(javaResource, JobMapper) {
/**
* The client's unique identifier of the job, re-used across retried attempts. If this field is set, the service will ensure its uniqueness. The request to create a job will fail if the service has knowledge of a previously submitted job with the same client's ID and job name. The caller may use this field to ensure idempotence of job creation across retried attempts to create a job. By default, the field is empty and, in that case, the service ignores it.
*/
public val clientRequestId: Output
get() = javaResource.clientRequestId().applyValue({ args0 -> args0 })
/**
* The timestamp when the job was initially created. Immutable and set by the Cloud Dataflow service.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* If this is specified, the job's initial state is populated from the given snapshot.
*/
public val createdFromSnapshotId: Output
get() = javaResource.createdFromSnapshotId().applyValue({ args0 -> args0 })
/**
* The current state of the job. Jobs are created in the `JOB_STATE_STOPPED` state unless otherwise specified. A job in the `JOB_STATE_RUNNING` state may asynchronously enter a terminal state. After a job has reached a terminal state, no further state updates may be made. This field may be mutated by the Cloud Dataflow service; callers cannot mutate it.
*/
public val currentState: Output
get() = javaResource.currentState().applyValue({ args0 -> args0 })
/**
* The timestamp associated with the current state.
*/
public val currentStateTime: Output
get() = javaResource.currentStateTime().applyValue({ args0 -> args0 })
/**
* The environment for the job.
*/
public val environment: Output
get() = javaResource.environment().applyValue({ args0 ->
args0.let({ args0 ->
environmentResponseToKotlin(args0)
})
})
/**
* Deprecated.
*/
@Deprecated(
message = """
Deprecated.
""",
)
public val executionInfo: Output
get() = javaResource.executionInfo().applyValue({ args0 ->
args0.let({ args0 ->
jobExecutionInfoResponseToKotlin(args0)
})
})
/**
* This field is populated by the Dataflow service to support filtering jobs by the metadata values provided here. Populated for ListJobs and all GetJob views SUMMARY and higher.
*/
public val jobMetadata: Output
get() = javaResource.jobMetadata().applyValue({ args0 ->
args0.let({ args0 ->
jobMetadataResponseToKotlin(args0)
})
})
/**
* User-defined labels for this job. The labels map can contain no more than 64 entries. Entries of the labels map are UTF8 strings that comply with the following restrictions: * Keys must conform to regexp: \p{Ll}\p{Lo}{0,62} * Values must conform to regexp: [\p{Ll}\p{Lo}\p{N}_-]{0,63} * Both keys and values are additionally constrained to be <= 128 bytes in size.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy