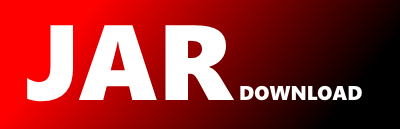
com.pulumi.googlenative.dataflow.v1b3.kotlin.TemplateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.dataflow.v1b3.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.dataflow.v1b3.TemplateArgs.builder
import com.pulumi.googlenative.dataflow.v1b3.kotlin.inputs.RuntimeEnvironmentArgs
import com.pulumi.googlenative.dataflow.v1b3.kotlin.inputs.RuntimeEnvironmentArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a Cloud Dataflow job from a template. Do not enter confidential information when you supply string values using the API.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
* @property environment The runtime environment for the job.
* @property gcsPath A Cloud Storage path to the template from which to create the job. Must be a valid Cloud Storage URL, beginning with `gs://`.
* @property jobName The job name to use for the created job.
* @property location The [regional endpoint] (https://cloud.google.com/dataflow/docs/concepts/regional-endpoints) to which to direct the request.
* @property parameters The runtime parameters to pass to the job.
* @property project
*/
public data class TemplateArgs(
public val environment: Output? = null,
public val gcsPath: Output? = null,
public val jobName: Output? = null,
public val location: Output? = null,
public val parameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy